Question
The program reads a text file with student records (first name, last name and grade) and prints them in the terminal window. After the name
The program reads a text file with student records (first name, last name and grade) and prints them in the terminal window.
After the name and grade it also prints the type of the grade: excellent (> 89), ok [60,89], and failure (< 60).
Then the program prints the number of students, and their average grade and also the number of students and their average in each grade category (excellent, ok, and failure).
For example, with the input file students.txt the program must print the following:
John Smith 90 excellent
Barack Obama 95 excellent
Al Clark 80 ok
Sue Taylor 55 failure
Ann Miller 75 ok
George Bush 58 failure
John Miller 65 ok
There are 7 students with average grade 74.0
There are 2 excellent students with average grade 92.5
There are 3 ok students with average grade 73.3333
There are 2 failre students with average grade 56.5
Requirements and restrictions
Use the Student.java and Students.java classes from the course website to represent and process student records and modify them accordingly:
The encapsulation principle must be strictly enforced.
The main method in class Students should only read the text file, and print student records (student names, grade and grade type) and statistics (number of students and averages) using methods of class Student.
All counting, totaling, computing averagas, checking grade intervals, and assigning grade types to students should be implemented in class Student. Hints: use static variables in class Student and add methods to compute and return (or print) averages. Modify the toString() method to return the grade type too.
When you write your program
use proper names for the variables suggesting their purpose.
format your code accordingly using indentation and spacing.
use multiple line comment in the beginning of the code and write your name, e-mail address, class, and section.
for each line of code add a short comment to explain its meaning.
Extra credit (2 points): The program also prints the student with the highest and the student with the lowest grade.
Students
import java.io.*; import java.util.*; public class Students { public static void main (String[] args) throws IOException { String first_name, last_name; int grade; double avg = 0; String comment = " "; double eGrade,oGrade,fGrade; int eCount,oCount,fCount,s,highest,lowest;//counts the number of excellent, ok , failure, average, highest, lowest highest = 0; lowest = 101; String hname,lname; hname=lname = " "; eGrade = oGrade= fGrade=eCount=oCount=fCount=s=0; Scanner fileInput = new Scanner(new File("students.txt"));//specify the location of the code while (fileInput.hasNext()) { first_name = fileInput.next(); last_name = fileInput.next(); grade = fileInput.nextInt();
Student st = new Student(first_name, last_name, grade); // grade = st.getGrade(); // if(grade > highest)//finds the student with the highest grade // { // highest = grade; // hname = first_name+" "+last_name; // } // if(grade < lowest)//find the student with the lowest grade // { // lowest = grade; // lname = first_name+" "+last_name; // }
System.out.println(st + " " + st.getGradetype()); s++; avg = avg+grade; } System.out.printf(" There are %d students with average grade %.2f",s,(avg/s));//prints out the average grade of all students together System.out.printf(" There are %d excellent student with average grade %.2f ", eCount,(eGrade/eCount));//prints out the student with the excellent grade System.out.printf(" There are %d ok with average grade %.2f", oCount,(oGrade/oCount));//prints out the students with the okey grade System.out.printf(" There are %d failure with average grade %.2f " ,fCount,(fGrade/fCount));//prints the number of students failing System.out.println(" "+hname+" score highest grade ="+highest);//prints out student highest grade System.out.println(" "+lname +" score lowest grade ="+lowest);//prints out student with lowest grade
} }
Student
public class Student { private String fname, lname; private int grade;
public Student(String fname, String lname, int grade) { this.fname = fname; this.lname = lname; this.grade = grade;
}
public int getGrade() { return grade; }
public String toString() { return fname + " " + lname + "\t" + grade;
}
public String getGradetype() {
if (grade > 89) { return "Excellent!"; }
else if (grade > 59) { return "OK"; }
return "Failure"; } }
This is what i have so far. am stuck becuase i did it before but i did it wrong and i need to make sure that
The main method in class Students should only read the text file, and print student records (student names, grade and grade type) and statistics (number of students and averages) using methods of class Student.
All counting, totaling, computing averagas, checking grade intervals, and assigning grade types to students should be implemented in class Student. Hints: use static variables in class Student and add methods to compute and return (or print) averages. Modify the toString() method to return the grade type too.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
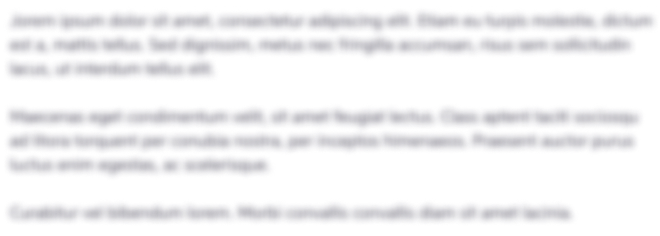
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started