Question
The Python function mergesort is shown below. It recursively sorts a list of numbers U using a recursive divide-and-conquer algorithm, then returns a sorted list
The Python function mergesort is shown below. It recursively sorts a list of numbers U using a recursive divide-and-conquer algorithm, then returns a sorted list S. The function head returns the first element of a non-empty list Q, and the function tail returns all but the first element of a non-empty list Q. Lines 0607 detect if U is trivially sorted. Lines 0916 split U into two halves, L and R, of approximately equal lengths. Lines 1718 recursively sort L and R. Lines 1928 merge the sorted L and R back into a sorted list S.
01 def head(Q): 02 return Q[0] 03 def tail(Q): 04 return Q[1:] 05 def mergesort(U): 06 if U == [] or tail(U) == []: 07 return U 08 else: 09 L = [] 10 R = [] 11 while U != [] and tail(U) != []: 12 L = L + [head(U)] 13 U = tail(U) 14 R = R + [head(U)] 15 U = tail(U) 16 L = L + U 17 L = mergesort(L) 18 R = mergesort(R) 19 S = [] 20 while L != [] and R != []: 21 if head(L) <= head(R): 22 S = S + [head(L)] 23 L = tail(L) 24 else: 25 S = S + [head(R)] 26 R = tail(R) 27 S = S + L + R 28 return S
Prove that mergesorts splitting loop is correct (lines 0916). Do not prove that the rest of mergesort is correct. Like the proofs in Cormens text, you must use a loop invariant. Your proof must have three parts: initialization, maintenance, and termination.
1a. |
| Find a loop invariant for the splitting loop. |
|
|
|
1b. |
| Use your loop invariant to prove the initialization part. |
|
|
|
1c. |
| Use your loop invariant to prove the maintenance part. |
|
|
|
1d. |
| Use your loop invariant to prove the termination part. |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
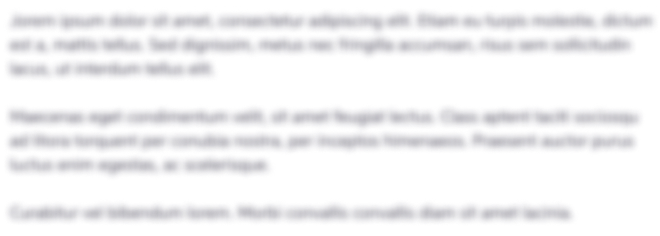
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started