THE QUESTION IS: Complete the skeleton program where it asks to insert missing code. IN JAVA
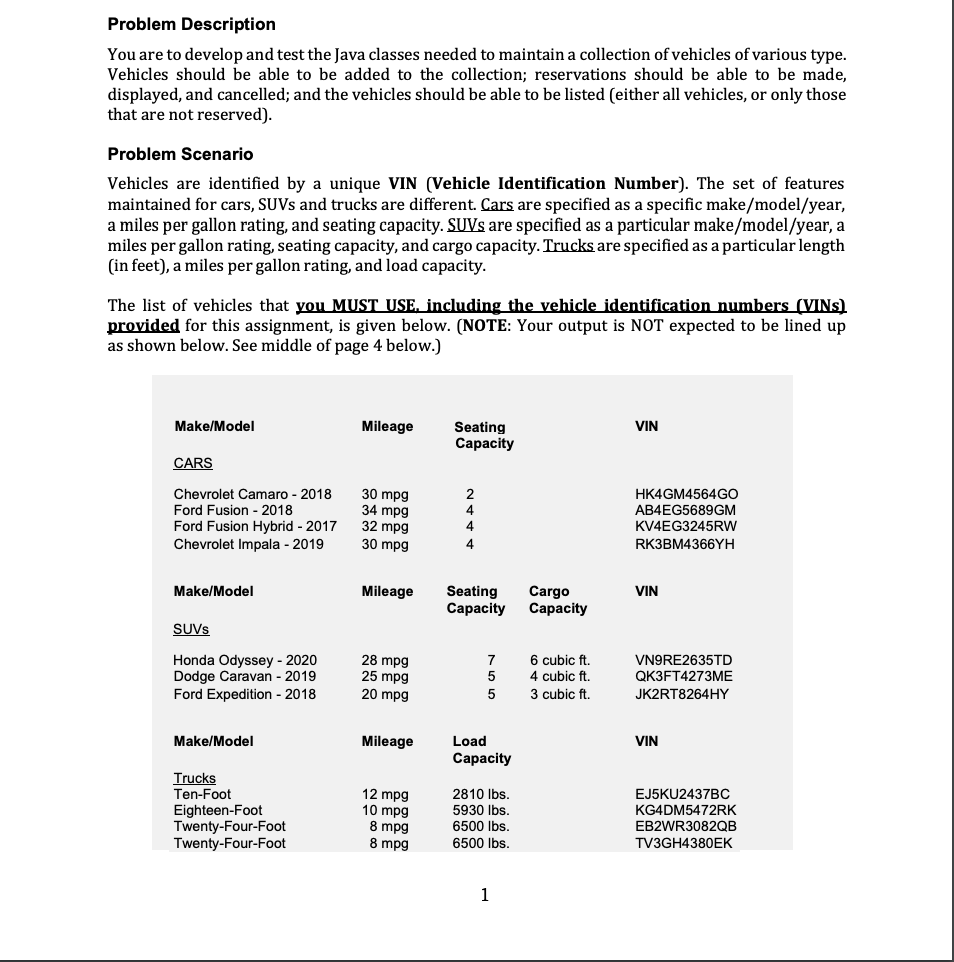
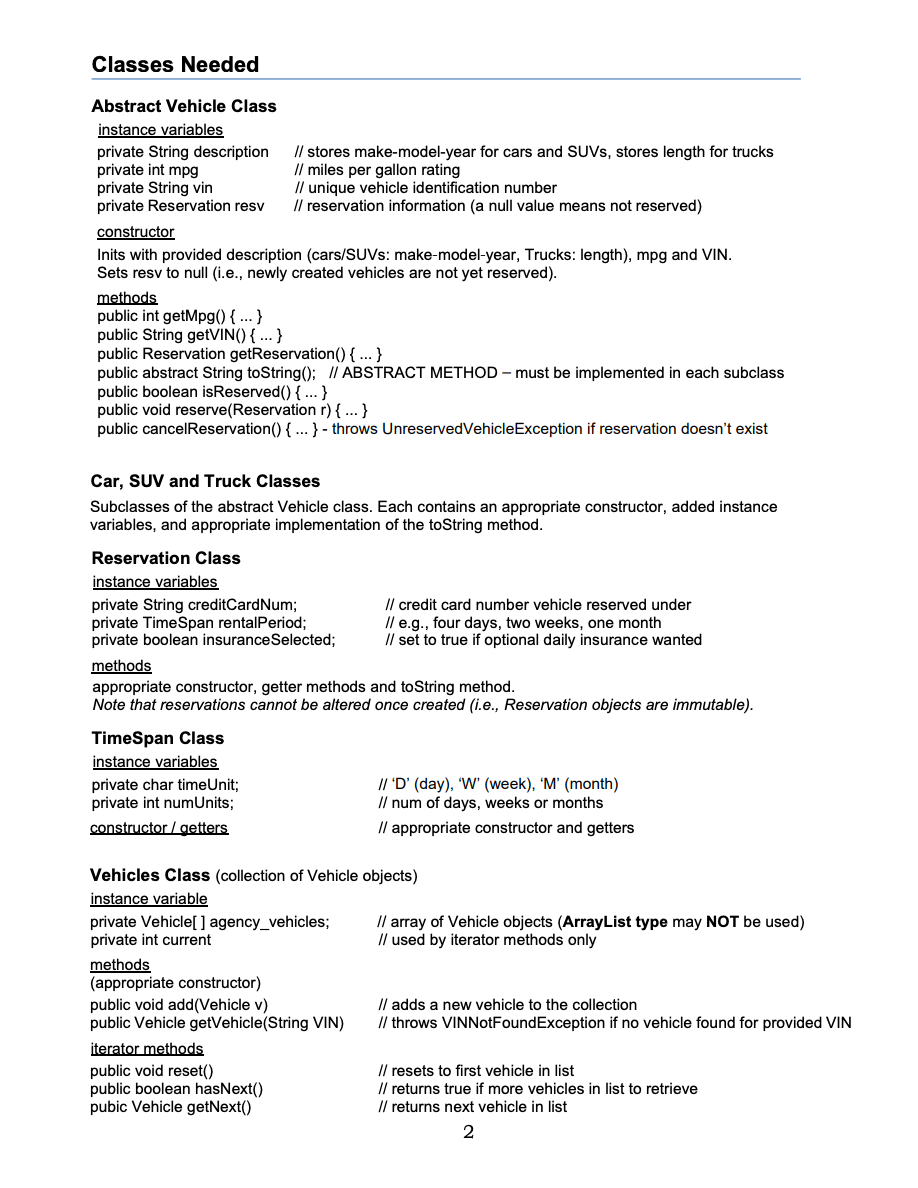
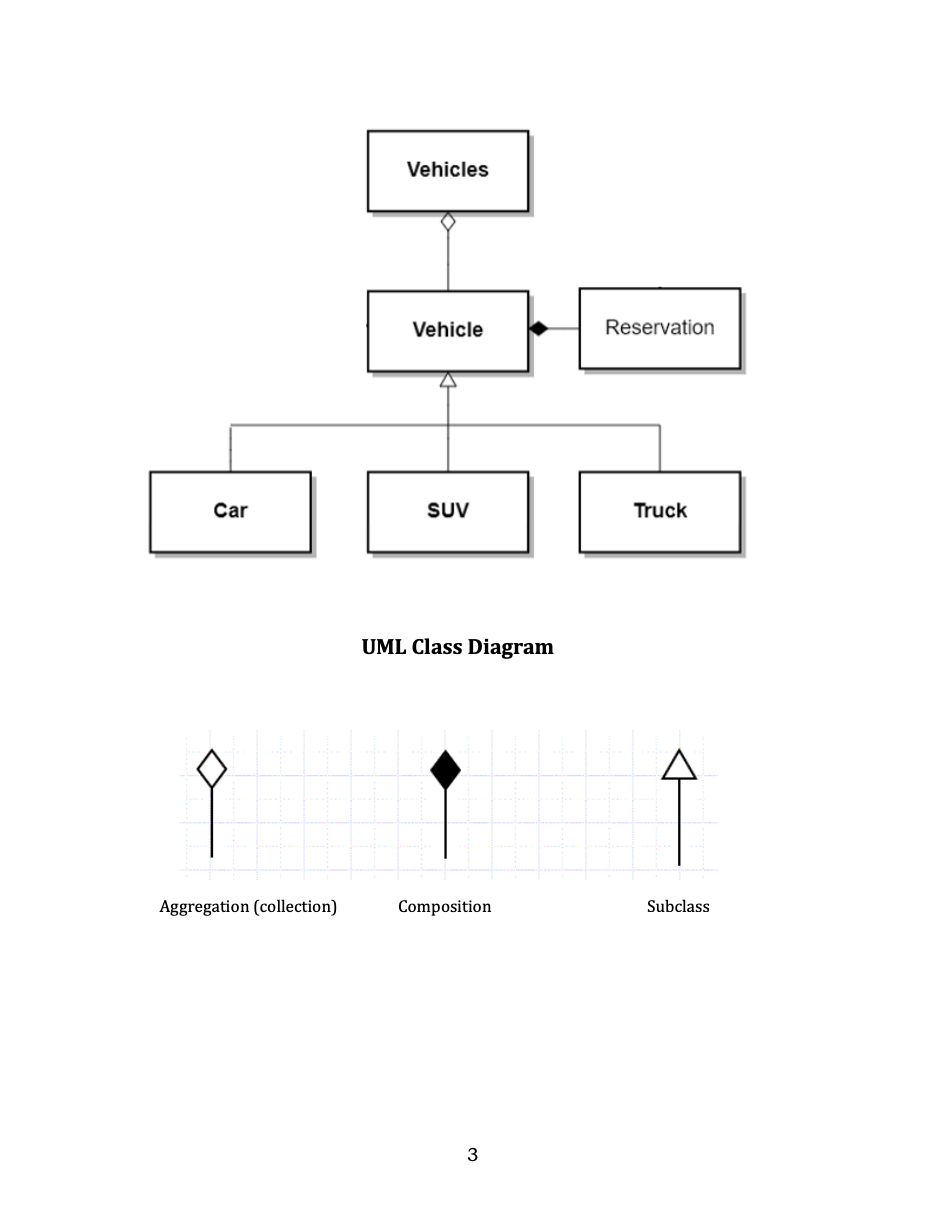
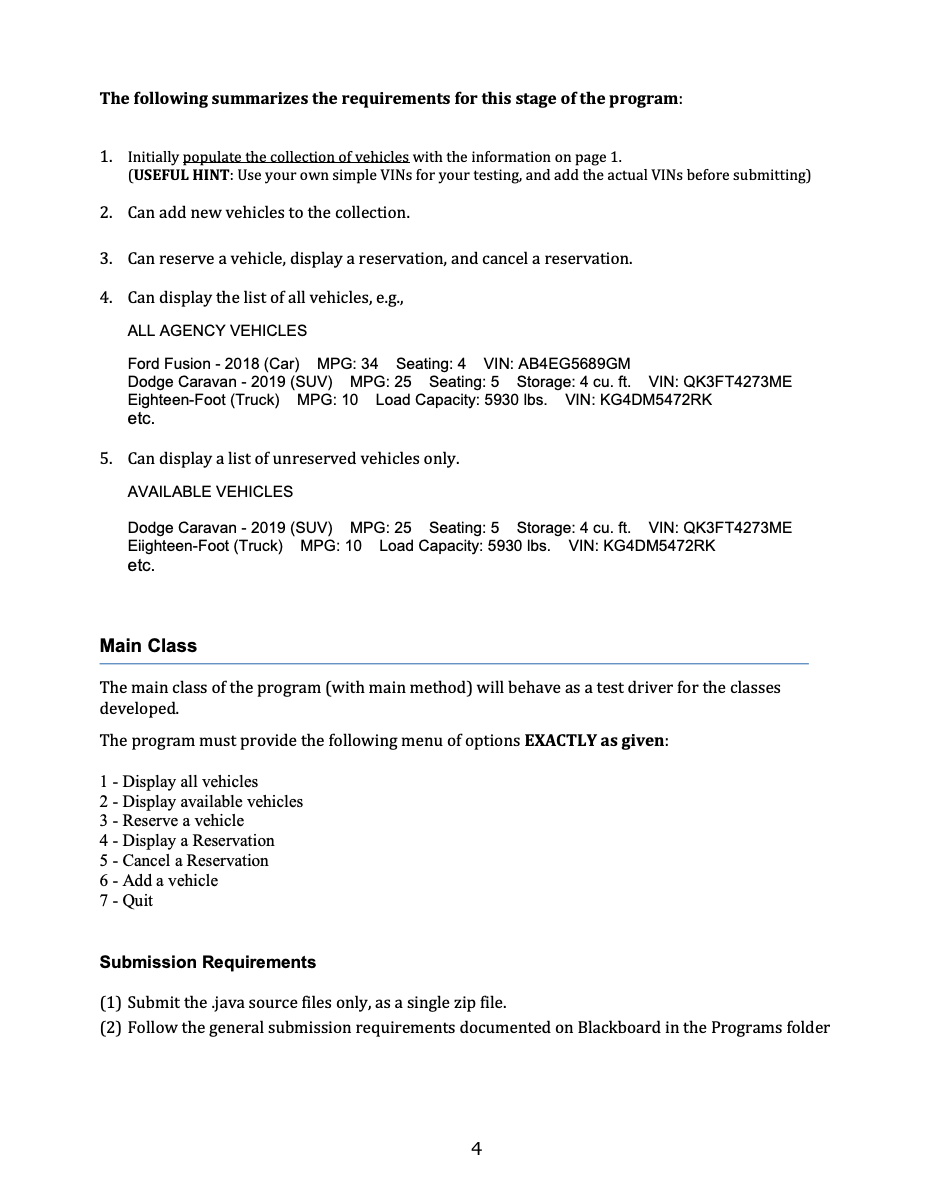
// import all vehicles-related classes
// and exceptions
import vehicles.Car;
import vehicles.SUV;
import vehicles.Truck;
import vehicles.Vehicle;
import vehicles.Vehicles;
import vehicles.Exceptions.*;
// import all utility classes
// and exceptions DONE
import utilities.*;
import utilities.Exceptions.*;
import utilities.Exceptions.NonDigitFoundException;
import utilities.Exceptions.InvalidNumberCharsException;
// import from Java API packages
import java.util.Scanner;
import java.util.InputMismatchException;
public class Main {
// ---------------------------------------------------------
// INITIALIZATION
// ---------------------------------------------------------
// symbolic constants
public static final int CAR = 1;
public static final int SUV = 2;
public static final int TRUCK = 3;
// create empty collection of vehicles DONE
private static Vehicles vehs = new Vehicles();
List list = Collections.EMPTY_LIST;
// init user input variables
private static boolean quit = false;
private static Scanner input = new Scanner(System.in);
// ---------------------------------------------------------
// COMMAND LOOP
// ---------------------------------------------------------
// main
public static void main(String[] args) {
// assign hardcoded set of vehicles
populateVehicles(vehs);
// command loop (for selection/execution of use cases)
int selection;
while(!quit){
displayMenu();
selection = getSelection();
execute(selection);
}
}
// ---------------------------------------------------------
// COMMAND LOOP SUPPORTING METHODS
// ---------------------------------------------------------
private static void displayMenu(){
// displays list of menu options
System.out.println(); // skip line
System.out.println("1 - Display All Vehicles");
System.out.println("2 - Display Available Vehicles");
System.out.println("3 - Reserve a Vehicle");
System.out.println("4 - Cancel a Reservation");
System.out.println("5 - Display Reservation");
System.out.println("6 - Add a Vehicle");
System.out.println("7 - Remove a Vehicle");
System.out.println("8 - Quit");
System.out.println();
}
private static int getSelection(){
// returns value between 1-8, inclusive
// reprompts user until valid entry entered
// to complete
}
private static void execute(int selection){
// executes the corresponding method implementing each use case
System.out.println(); // skip line
switch(selection){
case 1: displayAllVehicles(); break;
case 2: displayAvailVehicles(); break;
case 3: makeReservation(); break;
case 4: cancelReservation(); break;
case 5: displayReservation(); break;
case 6: addVehicle(); break;
case 7: removeVehicle(); break;
case 8: quit = true;
}
}
// ---------------------------------------------------------
// COMMAND IMPLEMENTATIONS
// ---------------------------------------------------------
// ---------------------------------------------------------
private static void displayAllVehicles(){
// ---------------------------------------------------------
// displays all vehicles (reserved and unreserved)
// .........................................................
Vehicle v;
vehs.reset();
while(vehs.hasNext()) {
v = vehs.getNextVehicle();
// to complete
}
// ---------------------------------------------------------
private static void displayAvailVehicles(){
// ---------------------------------------------------------
// displays all unreserved vehicles
// .........................................................
// to complete
}
// ---------------------------------------------------------
private static void makeReservation(){
// ---------------------------------------------------------
// request reservation information from user
// assigns reservation vehicle reserved
// .........................................................
String entered_VIN;
String entered_creditcard = ""; // init
String entered_rentalperiod = ""; // init
char YN_char;
boolean daily_insur_selected = false; // init
Vehicle veh = null;
// display heading
System.out.println("Reservation Entry ...");
try{
// get VIN of vehicle to reserve
// get vehicle for given VIN
veh = vehs.getVehicle(VIN);
// get desired rental period
// (e.g., D2 - two days, W3-three weeks, M1-one month)
unit = 'D'
num = 4
TimeSpan t = new TimeSpan(unit, num);
t.getUnit() - returns 'D', 'W' or 'M'
t.getNum() - return number
// request if insurance desired
// display details of vehicle found
// input and validate credit card
Reservation r = new Reservation(.., ..., ...);
// create reservation
veh.reserve(r);
}
catch(FailedVINEntryAttemptsException e){
System.out.println("Failed attempt at VIN entry.");
System.out.println("Returning to main menu ...");
}
catch(FailedRentalPeriodEntryException e){
System.out.println(); // skip line
System.out.println("Failed attempt at rental period entry.");
System.out.println("Returning to main menu ...");
}
catch(FailedCreditCardEntryAttemptsException e){
System.out.println("Failed attempt at credit card entry.");
System.out.println(); // skip line
System.out.println("Returning to main menu ...");
}
}
// ---------------------------------------------------------
private static void cancelReservation(){
// ---------------------------------------------------------
// requests credit card number of reservation to cancel
// .........................................................
String entered_creditcard = "";
char YN_char;
Vehicle veh;
// display heading
System.out.println("Cancel Reservation Entry ...");
try{
// get credit card number of reservation from user
// get reservation
// display reservation info
// confirm cancellation
}
catch(FailedCreditCardEntryAttemptsException e){
System.out.println("Failed attempt at credit card entry.");
System.out.println(); // skip line
System.out.println("Returning to main menu ...");
}
catch(ReservationNotFoundException e){
System.out.print("* Reservation Not Found for ");
System.out.println("Credit Card Number " + entered_creditcard + " *");
}
}
// ---------------------------------------------------------
private static void displayReservation(){
// ---------------------------------------------------------
// display the details of a given reservation
// .........................................................
String entered_creditcard = ""; // init
Vehicle veh;
// display heading
System.out.println("Display Reservation Entry ...");
try{
// get credit card number of reservation from user
// get reservation
// display reservation info
System.out.println(); // skip line
System.out.println("RESERVATION INFORMATION");
System.out.println(veh.toString());
System.out.println(veh.getReservation().toString())
}
catch(FailedCreditCardEntryAttemptsException e){
System.out.println("Failed attempt at credit card entry.");
System.out.println(); // skip line
System.out.println("Returning to main menu ...");
}
catch(ReservationNotFoundException e){
System.out.print("* RESERVATION NOT FOUND FOR ");
System.out.println("Credit Card Number " + entered_creditcard + " *");
}
}
// ---------------------------------------------------------
private static void addVehicle(){
// ---------------------------------------------------------
// requests vehicle information from user and adds to Vehicles collection
String veh_descript;
String VIN;
int mpg;
char YN_char;
int vehicle_type;
String value1 = ""; // init
String value2 = ""; // init
Vehicle new_veh = null;
// display heading
System.out.println("New Vehicle Entry ... ");
try{
// get vehicle type
// get vehicle description
//input.nextLine();
// get mpg
}
// get VIN
// add new vehicle
}
catch(FailedVINEntryAttemptsException e){
System.out.println("Failed attempts at VIN entry ...");
System.out.println("Returning to main menu ...");
}
catch(FailedCreditCardEntryAttemptsException e){
System.out.println("Failed attempts at credit card entry ...");
}
// add new vehicle to collection
// display confirmation info
}
private static void removeVehicle(){
// removes specified vehicle from the collection
String VIN = "";
char YN_char;
// display heading
System.out.println("Remove Vehicle Entry ...");
try{
// get credit card number of reservation from user
// display details of vehicle to remove
// confirm cancellatio
}
catch(FailedVINEntryAttemptsException e){
System.out.println("* Valid VIN not successfully entered *");
System.out.println(); // skip line
System.out.println("Returning to Main Menu ...");
}
}
// ---------------------------------------------------------
// GENERAL SUPPORTING METHODS
// ---------------------------------------------------------
private static void populateVehicles(Vehicles vehs){
// harded set of init vehicles
vehs.add(new Car("Chevrolet Camaro", 30, "2", "HK4GM4564GO"));
vehs.add(new Car("Ford Fusion", 34, "4", "AB4EG5689GM"));
vehs.add(new SUV("Honda Odyssey", 28, "7", "6", "VN9RE2635TD"));
vehs.add(new Truck("Ten-Foot", 12, "2810", "EJ5KU2437BC"));
// * add the rest of the vehicles here *
}
private static int getVehicleType(){
// prompts user for vehicle type (1-Car, 2-SUV, 3-Truck)
// returns symbolic value CAR, SUV or TRUCK
// return appropriate defined symbolic constant
}
private static boolean validVINChars(String vin){
// returns true if vin contains only A..Z and digits 0..9.
// returns false otherwise
}
// NOT NEEDED (can remove)------------------------------------------
private static boolean validateRentalPeriod(String rental_period)
throws InvalidRentalPeriodFormatException {
// returns true if rental_period formatted as
// where = 'D', 'W', or 'M', an integer value
// otherwise, return false. Throws InvalidRentalPeriodFormatException
// if rental_period found improperly formatted
// verifies that rental period of proper form
// rental period found invalid
// rental period verified OK
}
private static String getValidVINFromUser(){
// prompts user for valid VIN (i.e., containing only capital letters and digits).
// keeps prompting user until a valid VIN entered
}
private static String getValidExistingVINFromUser()
throws FailedVINEntryAttemptsException{
// prompts user for valid VIN (i.e., containing only capital letters and digits).
// throws a FailedVINEntryAttemptsException if failure to enter a proper
// VIN for an existing vehicle after three attempts
}
private static String getValidCreditCard()
throws FailedCreditCardEntryAttemptsException{
// prompts user for a properly formatted credit card (i.e., 16 digits),
// and throws a FailedCreditCardEntryAttemptsException if failure to enter a
// properly formatted rental period after three attempts
}
private static String getValidRentalPeriod() throws FailedRentalPeriodEntryException{
// prompts user for a properly formatted rental period, and returns
// throws a FailedRentalEntryAttemptsException if failure to enter a
// properly formatted rental period after three attempts
}
private static Vehicle getReservedVehicleByCreditCard(String credit_card)
throws ReservationNotFoundException {
// returns Vehicle object reserved with provided credit card number
// if no such vehicle found, throws a ReservationNotFoundException
}
}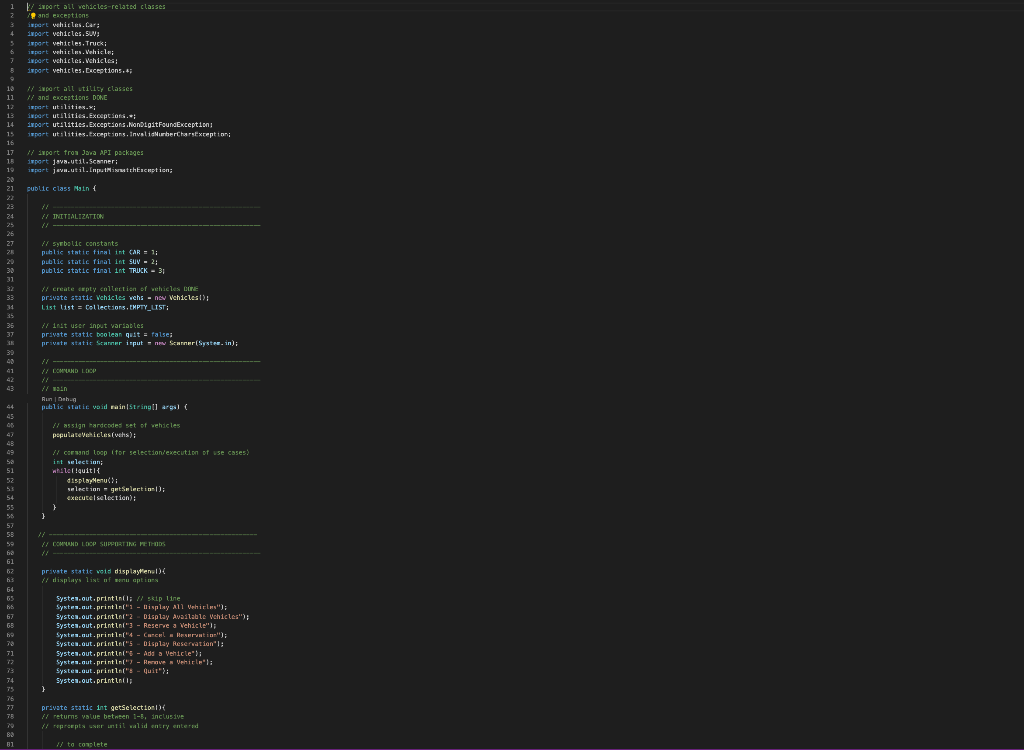
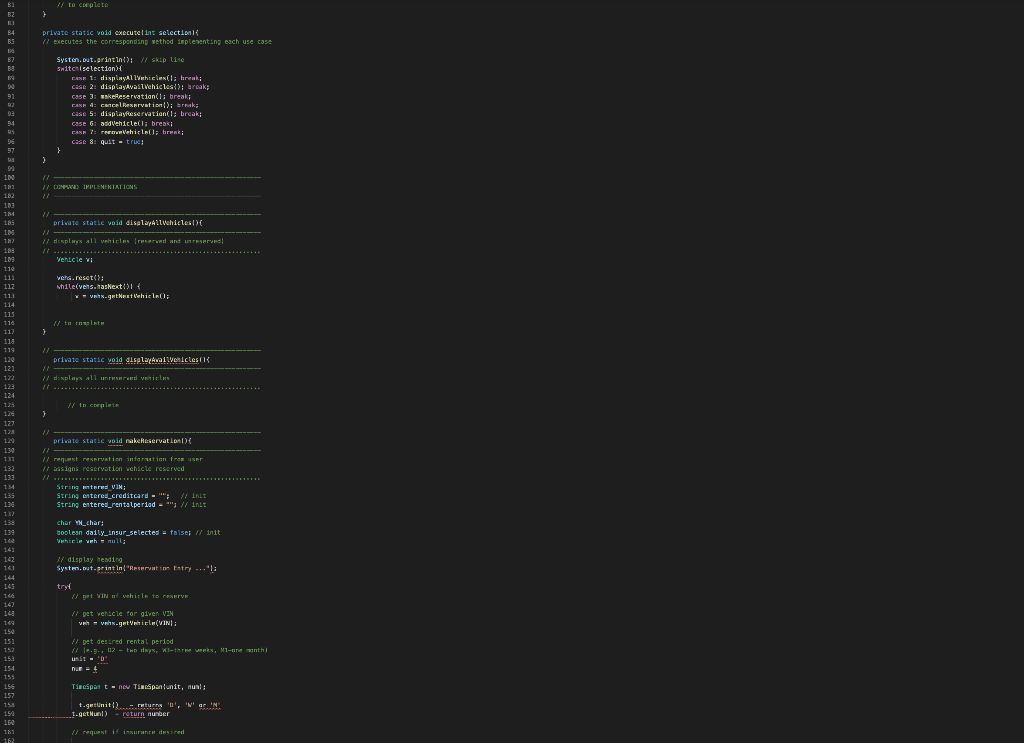
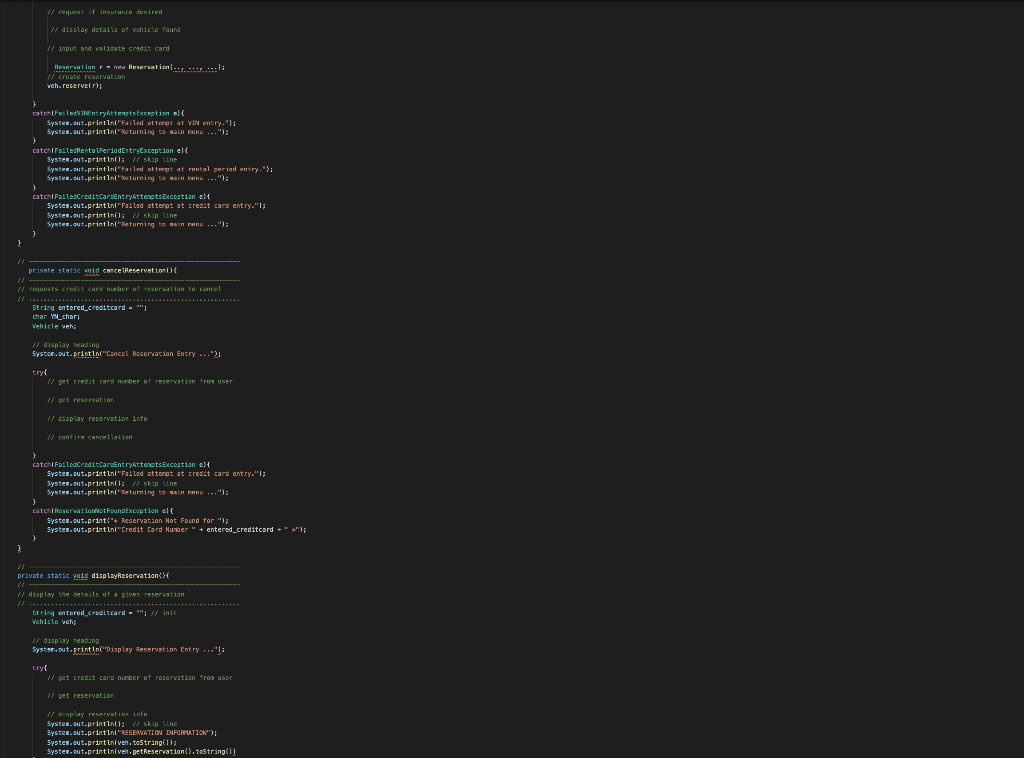
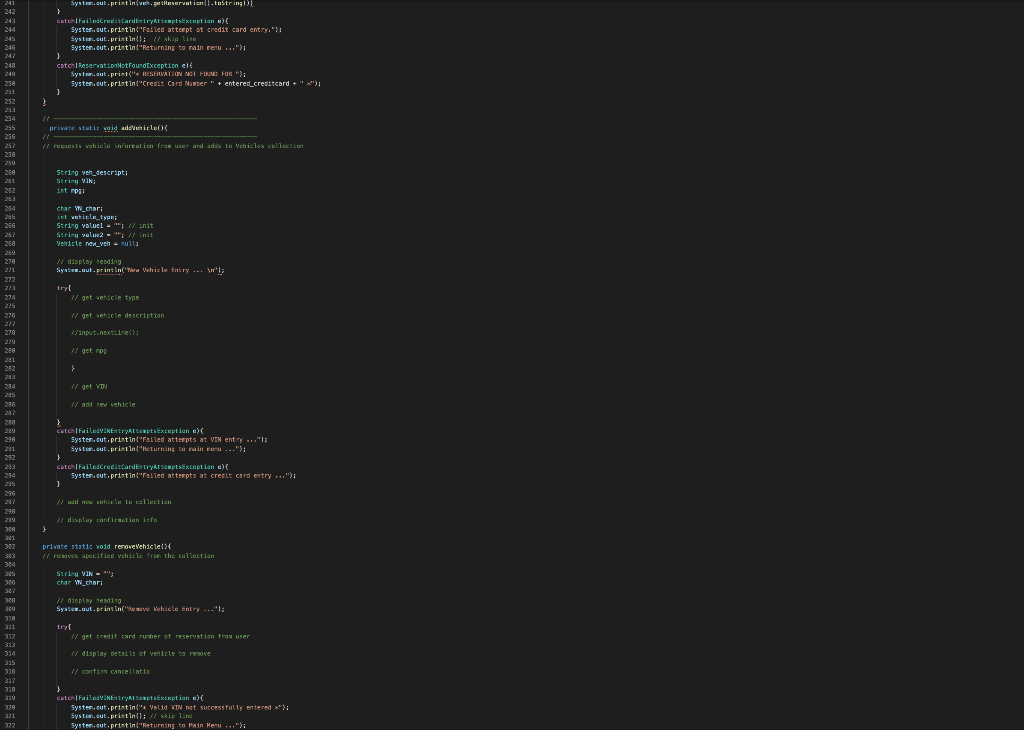
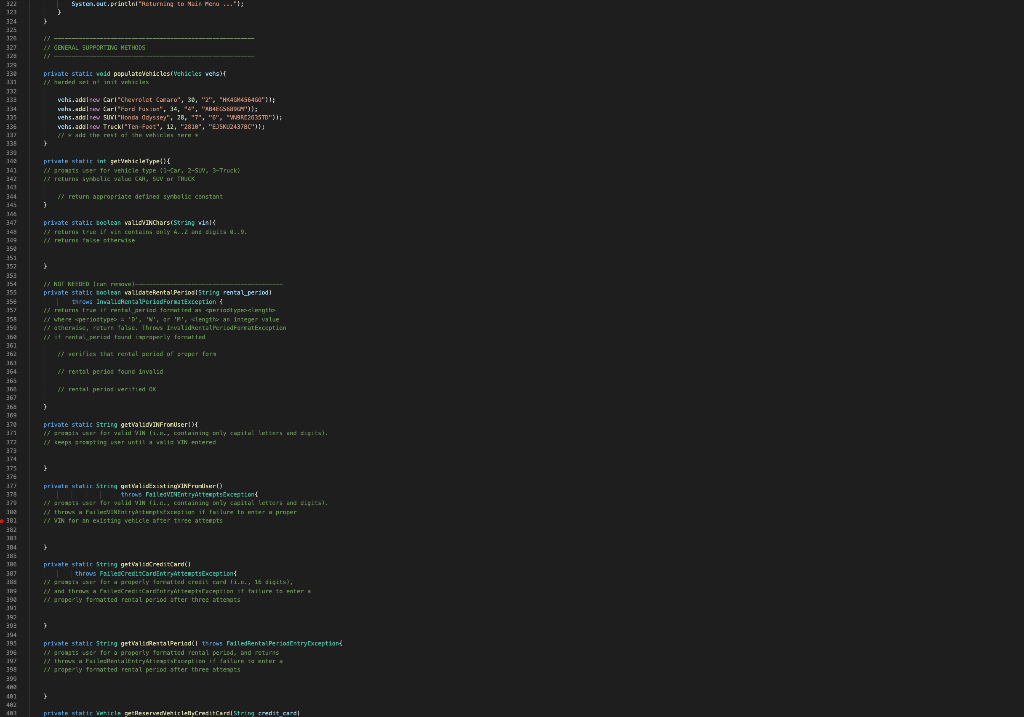
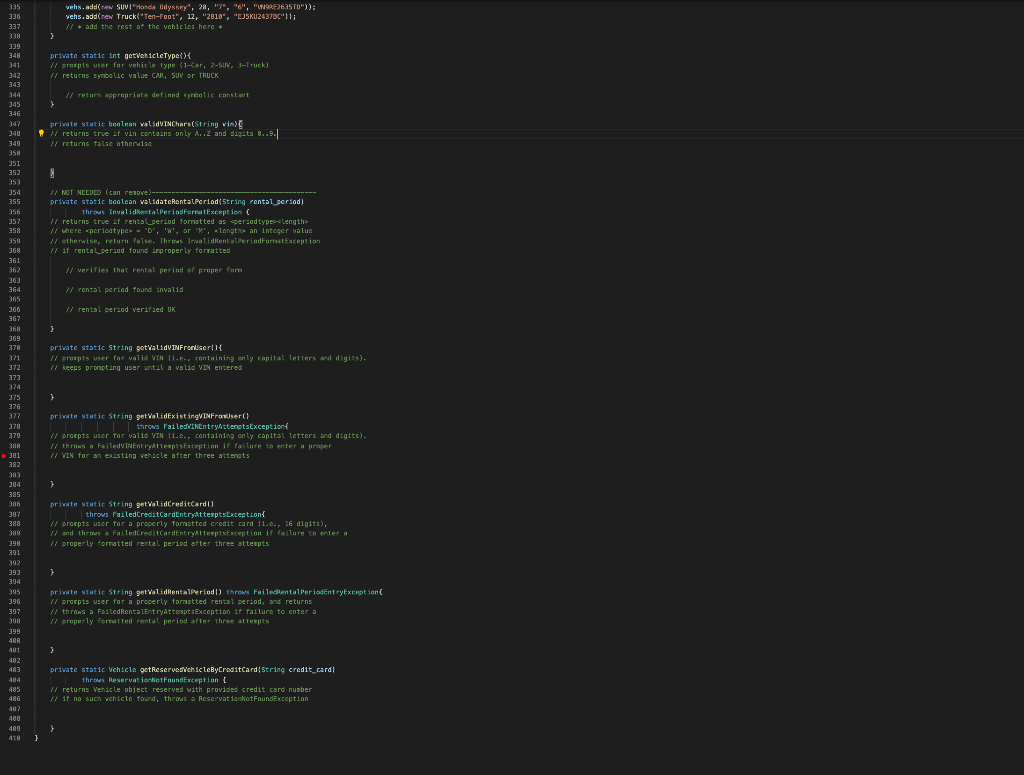
Problem Description You are to develop and test the Java classes needed to maintain a collection of vehicles of various type. Vehicles should be able to be added to the collection; reservations should be able to be made, displayed, and cancelled; and the vehicles should be able to be listed (either all vehicles, or only those that are not reserved). Problem Scenario Vehicles are identified by a unique VIN (Vehicle Identification Number). The set of features maintained for cars, SUVs and trucks are different. Cars are specified as a specific make/model/year, a miles per gallon rating, and seating capacity. SUVs are specified as a particular make/model/year, a miles per gallon rating, seating capacity, and cargo capacity. Trucks are specified as a particular length (in feet), a miles per gallon rating, and load capacity. The list of vehicles that you MUST USE, including the vehicle identification numbers (VINS) provided for this assignment, is given below. (NOTE: Your output is NOT expected to be lined up as shown below. See middle of page 4 below.) Make/Model Mileage VIN Seating Capacity CARS Chevrolet Camaro - 2018 Ford Fusion - 2018 Ford Fusion Hybrid - 2017 Chevrolet Impala - 2019 30 mpg 34 mpg 32 mpg 30 mpg HK4GM4564GO AB4EG5689GM KV4EG3245RW RK3BM4366YH 4 Make/Model Mileage VIN Seating Capacity Cargo Capacity SUVs Honda Odyssey - 2020 Dodge Caravan - 2019 Ford Expedition - 2018 28 mpg 25 mpg 20 mpg 7 5 5 6 cubic ft. 4 cubic ft 3 cubic ft. VNSRE2635TD QK3FT4273ME JK2RT8264HY Make/Model Mileage VIN Load Capacity Trucks Ten-Foot Eighteen-Foot Twenty-Four-Foot Twenty-Four-Foot 12 mpg 10 mpg 8 mpg 8 mpg 2810 lbs. 5930 lbs. 6500 lbs. 6500 lbs. EJ5KU2437BC KG4DM5472RK EB2WR3082QB TV3GH4380EK 1 Classes Needed Abstract Vehicle Class instance variables private String description Il stores make-model-year for cars and SUVs, stores length for trucks private int mpg Il miles per gallon rating private String vin I/ unique vehicle identification number private Reservation resv Il reservation information (a null value means not reserved) constructor Inits with provided description (cars/SUVs: make-model-year, Trucks: length), mpg and VIN. Sets resv to null (i.e., newly created vehicles are not yet reserved). methods public int getMpg() { ... } public String getVIN() { ... } public Reservation getReservation() { ... } public abstract String toString(); // ABSTRACT METHOD - must be implemented in each subclass public boolean isReserved() { ... } public void reserve (Reservation e) {...} public cancelReservation() { ... } - throws Unreserved VehicleException if reservation doesn't exist Car, SUV and Truck Classes Subclasses of the abstract Vehicle class. Each contains an appropriate constructor, added instance variables, and appropriate implementation of the toString method. Reservation Class instance variables private String credit CardNum; Il credit card number vehicle reserved under private Time Span rentalPeriod; Il e.g., four days, two weeks, one month private boolean insurance Selected; Il set to true if optional daily insurance wanted methods appropriate constructor, getter methods and to String method. Note that reservations cannot be altered once created (i.e., Reservation objects are immutable). TimeSpan Class instance variables private char timeUnit; // 'D' (day), 'W' (week), 'M' (month) private int numUnits; Il num of days, weeks or months constructor / getters Il appropriate constructor and getters Vehicles Class (collection of Vehicle objects) instance variable private Vehicle[ ] agency_vehicles; Il array of Vehicle objects (ArrayList type may NOT be used) private int current // used by iterator methods only methods (appropriate constructor) public void add(Vehicle v) Il adds a new vehicle to the collection public Vehicle getVehicle(String VIN) // throws VINNotFoundException if no vehicle found for provided VIN iterator methods public void reset() Il resets to first vehicle in list public boolean hasNext() // returns true if more vehicles in list to retrieve pubic Vehicle getNext() // returns next vehicle in list 2 Vehicles Vehicle Reservation Car SUV Truck UML Class Diagram Aggregation (collection) Composition Subclass 3 The following summarizes the requirements for this stage of the program: 1. Initially populate the collection of vehicles with the information on page 1. (USEFUL HINT: Use your own simple VINs for your testing, and add the actual VINs before submitting) 2. Can add new vehicles to the collection. 3. Can reserve a vehicle, display a reservation, and cancel a reservation. 4. Can display the list of all vehicles, e.g., ALL AGENCY VEHICLES Ford Fusion - 2018 (Car) MPG: 34 Seating: 4 VIN: AB4EG5689GM Dodge Caravan - 2019 (SUV) MPG: 25 Seating: 5 Storage: 4 cu. ft. VIN: QK3FT4273ME Eighteen-Foot (Truck) MPG: 10 Load Capacity: 5930 lbs. VIN: KG4DM5472RK etc. 5. Can display a list of unreserved vehicles only. AVAILABLE VEHICLES Dodge Caravan - 2019 (SUV) MPG: 25 Seating: 5 Storage: 4 cu. ft. VIN: QK3FT4273ME Elighteen-Foot (Truck) MPG: 10 Load Capacity: 5930 lbs. VIN: KG4DM5472RK etc. Main Class The main class of the program (with main method) will behave as a test driver for the classes developed. The program must provide the following menu of options EXACTLY as given: 1 - Display all vehicles 2 - Display available vehicles 3 - Reserve a vehicle 4 - Display a Reservation 5 - Cancel a Reservation 6 - Add a vehicle 7 - Quit Submission Requirements (1) Submit the .java source files only, as a single zip file. (2) Follow the general submission requirements documented on Blackboard in the Programs folder 4 1 2 2 suport all vehicles related classes and exceptions Gore import whicles.Car; suport vehicles. SUV 1 port vehicles. True import white. Vehicle suport white chicles; eport vehicles. Exceptions.t; 12 11 12 13 14 15 15 17 15 19 23 21 W suport all utility classes w and exception: DONE 1 port utilities. import utilities. Exceptions. suport utilities. Exceptions.Non igitFoundException Teport utilities.Exceptions. Invalid urbercharsexception 1/ suport from Ja API packages sport java.util.Semineri ieport java.util. InputhEaception public class NL 23 24 25 X INITIALIZATION 27 25 27 sebalic constants public static final in CAR = 1; public static final int SUV - 2; public static final int TRUCK - 31 31 // create cupty collection of vehicles DONE private static vehicles vaht - now Vehiclesi); List List = Collections.EMPTY_LIST: 33 11 35 35 37 init usor input variables private static boolean quit = false; private static Scanner input = new Scanner(Systein); 32 27 // COMAND LOOP 42 //win Runeb public static void main(Stringfl 941 ( 44 45 00 1/35ign hardcodes set of wehicles populateVehiclesives); 58 51 // cond loop for selection'execution of use cases) int selection whiler!quitit displayMenu(); selection = getSelection); execute selection); 55 54 55 XX COMMOND LOOP SUPPORTING PETHODS 57 53 59 ba 61 62 13 64 private static void displayten14 // displays list of wonu options 6 67 he 73 71 System.out.printlnil; // skip line System.out.println("! - Display All Vehitle"); System.out.println("2 - Display Available Vehicles"); Systes.out.println - Reserve a vehicle"; System.out.println("4 - Concel. Heservation'); System.out.println("5 - Display Display ": "; Systes.out.println("s - Add a Vehicle"); Systes.out.println(") - Reuve Vehirle): System.out.printlos - Quit's System.out.printlnti; } 73 74 TS 76 77 TE private static int getSelection y return value between 1-3, inclusive reproept er until walid entry entered 82 81 to complete 17 to complete Bi B2 34 BS B7 B! private static void CRCUT in selection // executes the corresponding method implementing each use case System.out.grintin); // skip line switchiselection CASH 1: displayAll Vehicles(); break; case 2: displayAvailvehicles): areak; case 3: Eskeservation ti break; CASH &: cancel Reservation); reak; Case S: displayfueservation, break; case G: asdVehicled; break; CAKE 7: removeVehitle(); break; Case 8: quit = true; 03 31 04 96 97 12 E IMPLEMENTATIONS private static void disalaltwhicle displays all vehicles reserved and reserved Vehicle 100 11 182 103 18 185 10 11 188 109 112 111 112 11.1 114 11 115 116 112 11! 119 120 121 veta.reset(); whilevens.nashext) Y-verk.gutest Vehicle; private static void disalavat Wenicek 17 displays all unreserve 11 // to ceplete private static void nakeReservation rest reservation infarction free use // assigns reservation vehicle reserved String entend VIN; String onteres_creditcard - "" // init String enteres_rentalperiod - "" // init char _chari boolean daily_insur_selected = false; // init Vehicle wah = null; 123 124 125 126 127 134 1N 130 111 132 133 154 135 136 13) 138 139 142 141 142 16 144 145 146 147 143 149 152 151 152 153 154 155 156 157 153 150 100 161 162 // display heading System.out.println("Reservation Entry -.. try get VIN a vehicle to exerVR pet vehicle for given VIN V = var.geli (VIN) veget: U pet desired rental period ul... 12 - two day, WITH , M1-ane minth NE 4 Tiucapan t-new TiueSpanlunit, nul; t.getUnit...-. turns 'B', ' War IN 1- I get Nunt) - return nunber A requl insurance desired // request if insurance desire // display details of vehicle found 17 input and validate credit card Retion reservation.........: // create reservation weh. reserver) } catch(ledvinntryktteaptstxception et Systen.out.println("Failed attempt at VIN entry." System.out.println("Returning to main menu ..."); } catchiFailed RentalPeriodEntryException el System.out.printin skip line Systes.out.println("Failed at at rental period entry."); System.out.println("keturning to main menu ..."); } catchFileCredit CardEntryAttenptsException et System.out.println("Failed attempt at credit card entry."); System.out.println; skip line Syster.out.println("Returning to sin menu ..."); } } 77 private static void cancelReservation { roguests credit card number of reservation to cancel String entered_creditcard - "" char Yuchari Wehicle veh: w display reading System.out.println("Cancel Reservation Entry ... -2: try 17 pet credit card number of reservation from user // get reservation // display reservation info i confin cancellation } catch FileCreditcardentryAttestsException et System.out.println("Failed sttorgt at credit card entry."); System.out.println; // skip line Systes.out.println("Returning to ani nenu..."); ) catchReservationNotFoundException el System.out.print Reservation Not Found for "); Syster.out.printlniCredit Card Nunter " + entered_creditcard + " "); 1 } 11 private static void display Reservation { display the details of a given reservation String entered_creditcard - "; // init Vehicle veh; { display ten System.out.println("Display Reservation Entry ...1: try 17 pot credit card number of reservation from user 17 pet reservation 3/ display reservation info System.out.printl0; // skip line System.out.println("RESERVATION INFORMATION"); System.out.printiniveh.toString(); System.out.printiniven.petReservation().tostring() 21 242 244 245 246 System.out.printlneh. geservation.tostring } catchFailud Credit Card EntryAttemptsextuption ) System.out.println("Failed attept st credit card entry," System.out.println(h / skip line System.out.print in Returning to rain renu ...") ] catch Reservation NotFoundException ek System.out.print("RESERVATION NOT FOUND FOR "); System.out.println("Credit Card Nawber" + entered_creditcard + "X"); ] 248 250 251 252 254 255 256 private stetit wid advico .... rests vehicle information from user and add to Vehicles collection 258 259 258 String veh_descripti String Viki int mal 252 204 256 char Wi_char int wielu_type: String valuel - ""; // init String value=''; // init Vehicle new_veh rulli 258 269 278 221 272 wa 274 { display resing Systes.out.println('n Vohicle Entry ... : tryl s! get vehicle type 276 get vehicle description 278 /input.nextLines 218 ! get me 282 //get VN 284 245 286 My addrew vehicle 288 298 } catch FailedVINEntryAEG PESException e) System.out.println("Failed attempts at VIN entry ..."); System.out.println("Returning to main rou ..."); } catch FailedCreditcard EntryAttemptsException e) { System.out.print intrailed attempts at credit card entry ..."); 3 292 233 294 296 // whicle to collection 298 XX display confirmation info } 3A 301 332 303 334 305 386 private static void remove Vehicles 1/ reves specified vehicle from the collection String VIN- char N chari w display tending Systes.out.println("we vehicle Entry ...! try 17 get credit card munber of reservation tras user !! display details of vehicle to remove 338 330 329 318 321 312 313 314 315 316 317 310 319 328 321 327 // contin cancellatio } catch FailedVINEntryAttemptsException e) System.out.println(": Vsud VIN nat successfully entered *"); Systcut.println(li stip line . System.out.printin("Returning to Main Menu ..."); System.out.printl"Returning to Male Peru...'13 ) 2 X GENERAL SUPPORTING METHODS private static void populatechiclesichicles vehe) harded at at init vehicles 322 121 324 325 120 327 323 129 332 331 132 333 334 335 335 1 338 331 102 41 vehsadalow Cari"Chevrolet Camaro", 30, "z", "HKAM456460"; vwhw.adra Gurl"Ford Fusion", 44, 45, 4S')); vehs.adstre SUVI Honda Odyssey", 28, 7", "P", W9RE2635TO")); vehs.adal row Trucki"Ton Fool', 12. "2010, "EJSK1243780 Beads the rest of the vehemere } printe stwir int gat VehicleType: Ogrowts user for vehicle type 11-Car. 2-5W, 3-Truck i returns symbolic value CAH, SUV or THJK return appropriate defined symbolic constant ) 141 344 345 100 347 349 private static coolean vaLLOWINChars(String via i returns true ir vis contain only 6.2 206 sigits ... 1} returns talse etteris 352 351 HOT NEEDED True) private static toolean valtateRentalPerios String rental period throws ImalltalPeriodFormatexception { returns true if rental period formatted parasit precettes x where speriostype = 'p', 'N', ar '', length an integer walue atherwise, return false. Throws InvalidentalierlodFormatException // if rental period found iroperly formutted di verifies that rental period of seper fora 353 154 355 356 152 358 35 362 361 262 161 364 365 163 367 363 169 370 371 172 373 374 375 376 17 rental perios found invalid Ortal perin: versti OK ) private static String getValidFrom User!) // srests wer for valid VIN (1.1., containing only capital letters and diits). craps routing user until vid TK entered 2 private stutis String et validerintingwintruder ) throws FailedVINEntryAttemptsException Ni sretsser for valid VIN (i..., containing only capital letters and salts). // throws a HiVIntrattepstruption if failure to ter proper VIN for an existing vehicle after three attempts 378 37 2012 381 382 11 384 385 18 387 383 103 392 101 private static String get validCreditcard throws FilesCreditcard EntryAttemptsException? 17 sretser for a properly formatted credit card (i..., 16 digits), // and throws a l'ailes creditcardinti treptsaction it tailure to enter Agroperty formatted rental period after three attempts 192 393 394 395 29 19 393 392 412 4A) private static String get validRentalPeriodil throws Failed Rental PeriodentryException aromats user for a property formatted rental period, and retains thross FailukentalEntryAttestatsception if failure to enter aroperty formatted rental period after three attempts } 41 private statir Vehirle getReserved irleyCreditcardString credit card 335 336 337 vehs.add(new SUV Honda Odyssey, 28, "7", "6", "W9RE2635TD")); vehs.add(new Truck Ten-foot", 12, "2018", "LJSKU243700"; 17+ add the rest of the vehicles here + 2 339 340 341 342 343 private static int getehicleType() { // prompts user for vehicle type (1-ar, 2-SUV, 3-Truck) 1, 2- XX returns symbolic value CAR, SUV or TRUCK 1/ return appropriate defined syntetie constant > private statie boolean walidhinchars(String wing . I returns true at vin contains only A..Z and dagats 8.9.|| {{ returns falsc otherise // NOT NEEDLD (can remove) -- private static boolean validate RentalPeriod(String rental period) throws InvalidentalPeriodFormatException { // returns true af rental_geriod formatted as period type-length // where periodtype = 'D', 'W', or "",
384 385 386 307 388 389 private static String get validCreditcard throws FailedCreditcardEntryAttemptsException 1/ prorpts user for a properly foratted credit card 11.C., 16 digits), W/ and throws a FailedCreditcardEntryAttemptsException if failure to enter a // properly formatted rental period after three attempts 398 391 392 393 394 395 396 397 390 399 408 481 482 483 private static String get validRentalPeriod) throws FailedientalPeriodEntryException xx prompts user for a properly fomatted rental period, and returns // throws a FailedRentalEntryAttarptsException it failure to enter a // properly formatted rental period after three attests private static Vchicle getReservedvehicleByCreditcard String credit card) throus Reservation NotFoundException ! // returns Vehicle object reserved with provided credit card number // if no such vehicle found, throus a ReservationNotFoundException 484 485 406 487 480 489 418 }