Question
The skeleton code is provided for you. The sort and swap procedures are taken from the textbook. The program will allocate an array of length
The skeleton code is provided for you. The sort and swap procedures are taken from the textbook. The program will allocate an array of length 20, and initialize all elements with 0. The program will ask the user how many integers (n) they want to input, and then the program will take n integers as input and store those into the array. The read_array procedure will read n and then use a loop to read n integers. The print_array procedure will print the sorted array. Include appropriate prompts for input and output. Sorting_Skeleton.asm below
.data ### Declare myArray, an array of length 20 and initialize with 0. ### ### Declare appropriate strings and the space character for I/O prompts ### .text main: ### call the procedure for reading the array ### ### Save the size of the array as returned in v0 from the read_array procedure to s0 ### ### Assign appropriate values to a0 and a1 that will be the arguments for the sort procedure. ### ### Check the description of the sort procedure ### ### call the sort procedure ### ### move s0 to a1. a1 will be used by the print_array procedure ### ### call the print_array procedure ### j exit read_array: ### Read value of n and then read n integers to myArray. Use appropriate input prompts ### ### you need to create a while loop ### ### Use t registers for counters and array indices ### ### the size of the array (n) will be saved to v0 before returning to main #### sort: # Two arguments: a0 for the starting address of the array; a1 is the number of integers addi $sp,$sp,-20 # make room on stack for 5 registers sw $ra, 16($sp) # save $ra on stack sw $s3,12($sp) # save $s3 on stack sw $s2, 8($sp) # save $s2 on stack sw $s1, 4($sp) # save $s1 on stack sw $s0, 0($sp) # save $s0 on stack move $s2, $a0 # save $a0 into $s2 move $s3, $a1 # save $a1 into $s3 move $s0, $zero # i = 0 for1tst: slt $t0, $s0, $s3 # $t0 = 0 if $s0 $s3 (i n) beq $t0, $zero, exit1 # go to exit1 if $s0 $s3 (i n) addi $s1, $s0,-1 # j = i 1 for2tst: slti $t0, $s1, 0 # $t0 = 1 if $s1 < 0 (j < 0) bne $t0, $zero, exit2 # go to exit2 if $s1 < 0 (j < 0) sll $t1, $s1, 2 # $t1 = j * 4 add $t2, $s2, $t1 # $t2 = v + (j * 4) lw $t3, 0($t2) # $t3 = v[j] lw $t4, 4($t2) # $t4 = v[j + 1] slt $t0, $t4, $t3 # $t0 = 0 if $t4 $t3 beq $t0, $zero, exit2 # go to exit2 if $t4 $t3 move $a0, $s2 # 1st param of swap is v (old $a0) move $a1, $s1 # 2nd param of swap is j jal swap # call swap procedure addi $s1, $s1,-1 # j = 1 j for2tst # jump to test of inner loop exit2: addi $s0, $s0, 1 # i += 1 j for1tst # jump to test of outer loop exit1: lw $s0, 0($sp) # restore $s0 from stack lw $s1, 4($sp) # restore $s1 from stack lw $s2, 8($sp) # restore $s2 from stack lw $s3,12($sp) # restore $s3 from stack lw $ra,16($sp) # restore $ra from stack addi $sp,$sp, 20 # restore stack pointer jr $ra # return to calling routine swap: sll $t1, $a1, 2 # $t1 = k * 4 add $t1, $a0, $t1 # $t1 = v+(k*4) (address of v[k]) lw $t0, 0($t1) # $t0 (temp) = v[k] lw $t2, 4($t1) # $t2 = v[k+1] sw $t2, 0($t1) # v[k] = $t2 (v[k+1]) sw $t0, 4($t1) # v[k+1] = $t0 (temp) jr $ra # return to calling routine print_array: ### print the sorted array, myArray. The size of the array will be in a1. Use appropriate output text. ### ### you need to create a while loop ### ### Use t registers for counters and array indices ### exit: li $v0, 10 syscall
Right now I have code below and I need help on part print_array
.data myArray: .word 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0 inputPrompt: .asciiz "Enter the number of integers: " numPrompt: .asciiz "Enter an integer: " outputPrompt: .asciiz "The sorted array is: " space: .asciiz " " .text main: li $v0, 4 la $a0, inputPrompt syscall
li $v0, 5 syscall
move $s0, $v0 la $a0, myArray move $a1, $s0 jal read_array
la $a0, myArray move $a1, $s0 jal sort
li $v0, 4 la $a0, outputPrompt syscall
move $a1, $s0 jal print_array
j exit
read_array: li $t0, 0 move $s1, $s0 la $s2, myArray while: bge $t0, $s1, endWhile li $v0, 4 la $a0, numPrompt syscall
li $v0, 5 syscall
sw $v0, 0($s2) addi $s2, $s2, 4 addi $t0, $t0, 1 j while endWhile: move $v0, $s0 jr $ra
sort: # Two arguments: a0 for the starting address of the array; a1 is the number of integers addi $sp,$sp,-20 # make room on stack for 5 registers sw $ra, 16($sp) # save $ra on stack sw $s3,12($sp) # save $s3 on stack sw $s2, 8($sp) # save $s2 on stack sw $s1, 4($sp) # save $s1 on stack sw $s0, 0($sp) # save $s0 on stack move $s2, $a0 # save $a0 into $s2 move $s3, $a1 # save $a1 into $s3 move $s0, $zero # i = 0 for1tst: slt $t0, $s0, $s3 # $t0 = 0 if $s0 $s3 (i n) beq $t0, $zero, exit1 # go to exit1 if $s0 $s3 (i n) addi $s1, $s0,-1 # j = i 1 for2tst: slti $t0, $s1, 0 # $t0 = 1 if $s1 < 0 (j < 0) bne $t0, $zero, exit2 # go to exit2 if $s1 < 0 (j < 0) sll $t1, $s1, 2 # $t1 = j * 4 add $t2, $s2, $t1 # $t2 = v + (j * 4) lw $t3, 0($t2) # $t3 = v[j] lw $t4, 4($t2) # $t4 = v[j + 1] slt $t0, $t4, $t3 # $t0 = 0 if $t4 $t3 beq $t0, $zero, exit2 # go to exit2 if $t4 $t3 move $a0, $s2 # 1st param of swap is v (old $a0) move $a1, $s1 # 2nd param of swap is j jal swap # call swap procedure addi $s1, $s1,-1 # j = 1 j for2tst # jump to test of inner loop exit2: addi $s0, $s0, 1 # i += 1 j for1tst # jump to test of outer loop exit1: lw $s0, 0($sp) # restore $s0 from stack lw $s1, 4($sp) # restore $s1 from stack lw $s2, 8($sp) # restore $s2 from stack lw $s3,12($sp) # restore $s3 from stack lw $ra,16($sp) # restore $ra from stack addi $sp,$sp, 20 # restore stack pointer jr $ra # return to calling routine swap: sll $t1, $a1, 2 # $t1 = k * 4 add $t1, $a0, $t1 # $t1 = v+(k*4) (address of v[k]) lw $t0, 0($t1) # $t0 (temp) = v[k] lw $t2, 4($t1) # $t2 = v[k+1] sw $t2, 0($t1) # v[k] = $t2 (v[k+1]) sw $t0, 4($t1) # v[k+1] = $t0 (temp) jr $ra # return to calling routine
print_array: ### print the sorted array, myArray. The size of the array will be in a1. Use appropriate output text. ### ### you need to create a while loop ### ### Use t registers for counters and array indices ###
exit: li $v0, 10 syscall
Step by Step Solution
There are 3 Steps involved in it
Step: 1
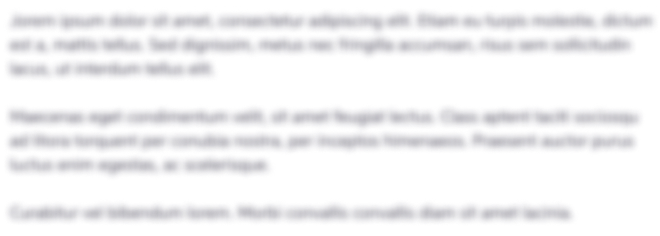
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started