Answered step by step
Verified Expert Solution
Question
1 Approved Answer
# The SL_Board class simulates a Snakes & Ladders board class SL_Board: def __init__(self, width, snakes, ladders): # Assumes the board is square (width wide
# The SL_Board class simulates a Snakes & Ladders board class SL_Board: def __init__(self, width, snakes, ladders): # Assumes the board is square (width wide by width tall) # Paramter: snakes: A list of integer tuples (head, tail), # where 1boardConfig.txt
6 14 3 23 10 31 13 34 25 4 13 17 28 8 29 24 26 29 33ExampleOutput.txt
SNAKES AND LADDERS The board: 1 2 S1T 4 5 6 12 11 S2T 9 L2B 7 S3T S1H 15 16 L1B 18 L3B S2H 22 21 20 19 S4T L3T 27 L1T L2T 30 36 35 S4H 33 32 S3H Player 1 = Aaron (q): 1 Player 2 = Quinn (a): 1 Aaron (q): 3 Quinn (a): 6 Aaron (q): 4 Quinn (a): 10 Aaron (q): 10 Quinn (a): 16 Aaron (q): 3 Quinn (a): 20 Aaron (q): 4 Quinn (a): 26 Aaron (q): 5 Quinn (a): 32 Aaron (q): 29 Quinn (a): 36 WINNER Quinn aAssignment Instructions: Carefully review the SI_Board class that is provided in the file Sl_Board.py file and the Coin class in Chapter 10 of your text book Part I: Write a Python class called Die (the singular of Dice!). Store it in a file called Die.py. This class should define the following methods: o definit (self) "Initializes the data attribute self. faceUp o def throw (self) Uses a random number generate to changee faceUp attribute. o def get faceUp (self): * Returns the current value of the faceUp attribute. Part II: Write a Python class called Player. Store it in a file called Player.py. The class should define the following meth o def init (self): *Initializes the data attributes self.__name and self. _symbol, both should be empty strings. It also initializes the attribute self. position to 1 Changes the attribute self. Changes the attribute self._symbol to a random character in the range 'a' to o def set name (self, name) name to the parameter passed as name o def set_symbol (self): * o def get name (self): o def get symbol (self) o def set position (self, position): 'z' (inclusive of both 'a' and 'z') returns the current value of the self. name attribute returns the current value of the self. symbol attribute Changes the attribute self. position to the parameter passed as position o def get position (self): * returns the current value of the self._position attribute de f-str-(self) : returns a string that contains the current value of each of the three attributes in the following format: value of self. name (value of self._symbol): self. position Part III: Write a Python program (stored in a file called SL_Game. py) that plays and displays a Snakes and Ladders game. Here are the specifications: -> Set up: o o The game starts by creating 1 board, 1 die and 2 player objects The data needed to initialize the board will be found in the file boardConfig.txt and has the following format: The first line contains a single number, size, representing the number of rows and columns on the board. The second line contains pairs of numbers in the range [2, size*size - 1] where the numbers 2 and size*size - 1 are included. The first number of each pair is certain to be larger than the second number. Each pair of numbers represents the location of the head and the location of the tail of a snake on the board. (Thus, the number of numbers on this line, divided by 2, is the number of snakes on the board.) Each pair of numbers should be grouped into a tuple and stored in a list of tuples. * The third line contains pairs of numbers in the range [2, size*size - 1] * where the numbers 2 and size*size - 1 are included. The first number of each pair is certain to be smaller than the second number. Each pair of numbers represents the locations of the bottom and the location of the top of a ladder on the board. (Thus, the number of numbers on this line, divided by 2, is the number of ladders on the board.) Each pair of numbers should be grouped into a tuple and stored in a list of tuples. Pass the number and two lists of tuples to the initializer method of the board. (A function (called boardData) that reads the configuration data from a file and calls the SL Board initializer is provided.) Print the full board to the output. Use mutator method(s) of the Player class to change the players' names to Aaron and Quinn Make sure the two player's symbols are different. Change one if needed using a mutator method of the Player class. o o o o Print out the full information for both players, in the following format: name (symbol): position * -> Playing the game: o The game is run completely without human interaction, as that is not necessary. o The program alternates giving the two players turns, as follows: A turn starts with a throw of the die. Change the player's position on the board * Add the value of the die throw to the player's current position . o if the position is greater than the length of the board, the position should be changed to the last square on the board. If there is the head of a snake or the bottom of a ladder on that square of the board, the position to is updated appropriately. (Note that the list element, that represents either a snake head or a ladder bottom, of the board object contains the number of the square that is either the tail of the snake or the top of the ladder.) . Print out the full information for the player, using format: name (symbol): position. * Winning the game: o The first player that ends the turn on the last square of the board is declared the winner Print out the word WINNER, followed by the name and symbol of the winning * The third line contains pairs of numbers in the range [2, size*size - 1] * where the numbers 2 and size*size - 1 are included. The first number of each pair is certain to be smaller than the second number. Each pair of numbers represents the locations of the bottom and the location of the top of a ladder on the board. (Thus, the number of numbers on this line, divided by 2, is the number of ladders on the board.) Each pair of numbers should be grouped into a tuple and stored in a list of tuples. Pass the number and two lists of tuples to the initializer method of the board. (A function (called boardData) that reads the configuration data from a file and calls the SL Board initializer is provided.) Print the full board to the output. Use mutator method(s) of the Player class to change the players' names to Aaron and Quinn Make sure the two player's symbols are different. Change one if needed using a mutator method of the Player class. o o o o Print out the full information for both players, in the following format: name (symbol): position * -> Playing the game: o The game is run completely without human interaction, as that is not necessary. o The program alternates giving the two players turns, as follows: A turn starts with a throw of the die. Change the player's position on the board * Add the value of the die throw to the player's current position . o if the position is greater than the length of the board, the position should be changed to the last square on the board. If there is the head of a snake or the bottom of a ladder on that square of the board, the position to is updated appropriately. (Note that the list element, that represents either a snake head or a ladder bottom, of the board object contains the number of the square that is either the tail of the snake or the top of the ladder.) . Print out the full information for the player, using format: name (symbol): position. * Winning the game: o The first player that ends the turn on the last square of the board is declared the winner Print out the word WINNER, followed by the name and symbol of the winning * Exclusively use objects: o one board, one die and two player objects *must* be used exclusively to store and manipulate all the data needed to for the game. A small exception: one integer and two lists of tuples can be used *only* to receive the configuration data from the input file and call the initializer method for the board (as shown in the called boardData function provided). o If, for example, the input file has the following data: 14 3 23 10 31 13 34 25 4 13 17 28 8 29 24 26 29 33 then resulting output should be: then resulting output should be: SNAKES AND LADDERS The board: 1 12 S3T L3B S4T 36 2 SIH S2H L3T 35 S1T S2T 15 4 9 16 21 L1T L2B LIB 20 2T 32 7 18 19 30 S3H 27 S4H Player 1- Aaron (q)1 Player 2- Quinn (a): 1 Aaron (q): 3 Quinn (a) 6 Aaron (q): 4 Quinn (a) 10 Aaron (g): 10 Quinn (a): 16 Aaron (g): 3 Quinn (a): 20 Aaron (q): 4 Quinn (a): 26 Aaron (q): 5 Quinn (a) 32 Aaron (g) 29 Quinn (a): 36 WINNER Quinn a (Recall that the die throws are random!) Grading This assign is worth 4% of your course grade. It will be graded as follows: 1 Mark: The Die class (found in the Die.py file) executes without error and a test program can create Die obiects and call the throw and get faceUp methods. The get faceUp method shows a random number between 1 and 6 (inclusive.) Grading This assign is worth 4% of your course grade. It will be graded as follows: 1 Mark: The Die class (found in the Die.py file) executes without error and a test program can create Die objects and call the throw and get_faceup methods. The get_faceUp method shows a random number between 1 and 6 (inclusive.) 2 Marks: The Player class (found in the Player.py file) executes without error and a test program can create Player objects, call the accessor and mutator methods. The_str__method produces the expected result. 2 Marks: The Snakes and Ladders game program submitted (in the file sL_Game.py) runs without error and produces a winner on the last line of the output If the above marks are achieved, then the submitted files will be inspected for the following: from thesi omnois and poastions and to prinr t: Marks: methods from the SL_Board and Player classes are used *exclusively* to print the board, change the player names, symbols and positions and to print the players 2 Marks: a function is defined that contains all the activities required for a single player's turn; it calls the methods of the Die, Player and SL_Board classes as appropriate. The function is called repeatedly for each player, thus avoiding repeated, sequential lines of similar code. 2 .1 Mark: Everything is documented appropriately, as defined in the style guidelines files Assignment Instructions: Carefully review the SI_Board class that is provided in the file Sl_Board.py file and the Coin class in Chapter 10 of your text book Part I: Write a Python class called Die (the singular of Dice!). Store it in a file called Die.py. This class should define the following methods: o definit (self) "Initializes the data attribute self. faceUp o def throw (self) Uses a random number generate to changee faceUp attribute. o def get faceUp (self): * Returns the current value of the faceUp attribute. Part II: Write a Python class called Player. Store it in a file called Player.py. The class should define the following meth o def init (self): *Initializes the data attributes self.__name and self. _symbol, both should be empty strings. It also initializes the attribute self. position to 1 Changes the attribute self. Changes the attribute self._symbol to a random character in the range 'a' to o def set name (self, name) name to the parameter passed as name o def set_symbol (self): * o def get name (self): o def get symbol (self) o def set position (self, position): 'z' (inclusive of both 'a' and 'z') returns the current value of the self. name attribute returns the current value of the self. symbol attribute Changes the attribute self. position to the parameter passed as position o def get position (self): * returns the current value of the self._position attribute de f-str-(self) : returns a string that contains the current value of each of the three attributes in the following format: value of self. name (value of self._symbol): self. position Part III: Write a Python program (stored in a file called SL_Game. py) that plays and displays a Snakes and Ladders game. Here are the specifications: -> Set up: o o The game starts by creating 1 board, 1 die and 2 player objects The data needed to initialize the board will be found in the file boardConfig.txt and has the following format: The first line contains a single number, size, representing the number of rows and columns on the board. The second line contains pairs of numbers in the range [2, size*size - 1] where the numbers 2 and size*size - 1 are included. The first number of each pair is certain to be larger than the second number. Each pair of numbers represents the location of the head and the location of the tail of a snake on the board. (Thus, the number of numbers on this line, divided by 2, is the number of snakes on the board.) Each pair of numbers should be grouped into a tuple and stored in a list of tuples. * The third line contains pairs of numbers in the range [2, size*size - 1] * where the numbers 2 and size*size - 1 are included. The first number of each pair is certain to be smaller than the second number. Each pair of numbers represents the locations of the bottom and the location of the top of a ladder on the board. (Thus, the number of numbers on this line, divided by 2, is the number of ladders on the board.) Each pair of numbers should be grouped into a tuple and stored in a list of tuples. Pass the number and two lists of tuples to the initializer method of the board. (A function (called boardData) that reads the configuration data from a file and calls the SL Board initializer is provided.) Print the full board to the output. Use mutator method(s) of the Player class to change the players' names to Aaron and Quinn Make sure the two player's symbols are different. Change one if needed using a mutator method of the Player class. o o o o Print out the full information for both players, in the following format: name (symbol): position * -> Playing the game: o The game is run completely without human interaction, as that is not necessary. o The program alternates giving the two players turns, as follows: A turn starts with a throw of the die. Change the player's position on the board * Add the value of the die throw to the player's current position . o if the position is greater than the length of the board, the position should be changed to the last square on the board. If there is the head of a snake or the bottom of a ladder on that square of the board, the position to is updated appropriately. (Note that the list element, that represents either a snake head or a ladder bottom, of the board object contains the number of the square that is either the tail of the snake or the top of the ladder.) . Print out the full information for the player, using format: name (symbol): position. * Winning the game: o The first player that ends the turn on the last square of the board is declared the winner Print out the word WINNER, followed by the name and symbol of the winning * The third line contains pairs of numbers in the range [2, size*size - 1] * where the numbers 2 and size*size - 1 are included. The first number of each pair is certain to be smaller than the second number. Each pair of numbers represents the locations of the bottom and the location of the top of a ladder on the board. (Thus, the number of numbers on this line, divided by 2, is the number of ladders on the board.) Each pair of numbers should be grouped into a tuple and stored in a list of tuples. Pass the number and two lists of tuples to the initializer method of the board. (A function (called boardData) that reads the configuration data from a file and calls the SL Board initializer is provided.) Print the full board to the output. Use mutator method(s) of the Player class to change the players' names to Aaron and Quinn Make sure the two player's symbols are different. Change one if needed using a mutator method of the Player class. o o o o Print out the full information for both players, in the following format: name (symbol): position * -> Playing the game: o The game is run completely without human interaction, as that is not necessary. o The program alternates giving the two players turns, as follows: A turn starts with a throw of the die. Change the player's position on the board * Add the value of the die throw to the player's current position . o if the position is greater than the length of the board, the position should be changed to the last square on the board. If there is the head of a snake or the bottom of a ladder on that square of the board, the position to is updated appropriately. (Note that the list element, that represents either a snake head or a ladder bottom, of the board object contains the number of the square that is either the tail of the snake or the top of the ladder.) . Print out the full information for the player, using format: name (symbol): position. * Winning the game: o The first player that ends the turn on the last square of the board is declared the winner Print out the word WINNER, followed by the name and symbol of the winning * Exclusively use objects: o one board, one die and two player objects *must* be used exclusively to store and manipulate all the data needed to for the game. A small exception: one integer and two lists of tuples can be used *only* to receive the configuration data from the input file and call the initializer method for the board (as shown in the called boardData function provided). o If, for example, the input file has the following data: 14 3 23 10 31 13 34 25 4 13 17 28 8 29 24 26 29 33 then resulting output should be: then resulting output should be: SNAKES AND LADDERS The board: 1 12 S3T L3B S4T 36 2 SIH S2H L3T 35 S1T S2T 15 4 9 16 21 L1T L2B LIB 20 2T 32 7 18 19 30 S3H 27 S4H Player 1- Aaron (q)1 Player 2- Quinn (a): 1 Aaron (q): 3 Quinn (a) 6 Aaron (q): 4 Quinn (a) 10 Aaron (g): 10 Quinn (a): 16 Aaron (g): 3 Quinn (a): 20 Aaron (q): 4 Quinn (a): 26 Aaron (q): 5 Quinn (a) 32 Aaron (g) 29 Quinn (a): 36 WINNER Quinn a (Recall that the die throws are random!) Grading This assign is worth 4% of your course grade. It will be graded as follows: 1 Mark: The Die class (found in the Die.py file) executes without error and a test program can create Die obiects and call the throw and get faceUp methods. The get faceUp method shows a random number between 1 and 6 (inclusive.) Grading This assign is worth 4% of your course grade. It will be graded as follows: 1 Mark: The Die class (found in the Die.py file) executes without error and a test program can create Die objects and call the throw and get_faceup methods. The get_faceUp method shows a random number between 1 and 6 (inclusive.) 2 Marks: The Player class (found in the Player.py file) executes without error and a test program can create Player objects, call the accessor and mutator methods. The_str__method produces the expected result. 2 Marks: The Snakes and Ladders game program submitted (in the file sL_Game.py) runs without error and produces a winner on the last line of the output If the above marks are achieved, then the submitted files will be inspected for the following: from thesi omnois and poastions and to prinr t: Marks: methods from the SL_Board and Player classes are used *exclusively* to print the board, change the player names, symbols and positions and to print the players 2 Marks: a function is defined that contains all the activities required for a single player's turn; it calls the methods of the Die, Player and SL_Board classes as appropriate. The function is called repeatedly for each player, thus avoiding repeated, sequential lines of similar code. 2 .1 Mark: Everything is documented appropriately, as defined in the style guidelines files
Step by Step Solution
There are 3 Steps involved in it
Step: 1
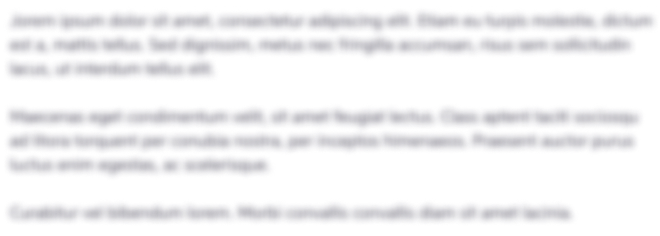
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started