Question
The University of NoGoldApples-Upstate, home of the Great Lames has decided to update its student registration system from its clay tablet based rolodex to a
The University of NoGoldApples-Upstate, home of the Great Lames has decided to update its student registration system from its clay tablet based rolodex to a new scheduling system called the Lunar System. They will identify their students by a unique identifier called the webid, which will consist of the first letter and the last name of the student. When students log in to the Lunar System, they will be able to view their classes, add a class, drop a class, and sort their classes by courseId or semester. The registrar must be able to see all the students that took a given course in a given semester, register, and deregister students. Once a student is deregistered, their past courses should disappear, as NoGoldApples-Upstate believes in revisionist history. Finally, as they have no gold apples to keep it going, the Lunar System is expected to go down a lot (some say, even more often than WolfieNet) for maintenance. In order to save face, UNoGoldApples should be able to save the state of the Lunar System (we will do this using serialization), and reload the data once the system is restarted.
You will develop the lunar system. As the most often accesses will be done by webid, you must store students in a HashMap, with the key being the webid.
NOTE: All exceptions explicitly thrown in Required Classes except for IllegalArgumentException are custom exceptions that need to be made by you.
Required Classes
Student
Write a fully-documented class named Student that will serve as the stored element of the Lunar System database. Each Student object includes a webID and a list of courses the student has taken, and getters and setters for each. Must implement the Serializable interface.
private String webID
private List
Course
Write a full-documented class named Course. Courses will be associated with the Students that are taking them. Each course will have a designated department (Ex. CSE), a three-digit course number (Ex. 214), and a semester associated with it (Ex. F2017). As always, each data field must have getters and setters. Must implement the Serializable interface.
private string department
private int number
private string semester
CourseNameComparator
Write a fully-documented class named CourseNameComparator that allows us to compare two course names with the following priority: department and then number. The CourseNameComparator class should implement the Comparator interface and override the compare method.
int compare (Course left, Course right)
Should return: -1 if the left course name is less than the right course name, 0 if they are equal, and 1 if the left course name is greater than the right course.
SemesterComparator
Write a fully-documented class named Semester that allows us to compare two courses based on the semester they were offered. The SemesterComparator class should implement the Comparator interface and override the compare method.
int compare (Course left, Course right)
Should return: -1 if the left courses semester is less than the right, 0 if the semesters are equal, and 1 if the lefts semester is greater than the right.
LunarSystem
Write a full-documented class named LunarSystem that will allow the user to interact with a database of Students. Provide the user with a menu-based interface that allows them to add, remove, and edit Students, as well as their courses. You will need to be able to serialize (save) the database at the end of the program and deserialize (load) the database if a file containing the database already exists. The database will have a Students netID as the key and the associated Student object as the value.
On startup, the LunarSystem should check to see if the file Lunar.ser exists in the current directory. If it does, then the file should be loaded and deserialized into the database instance. If the file does not exist, a new HashMap should be created and used instead. In either case, the user should be allowed to fully interact with the LunarSystem without issues.
private HashMap
Public static void main(String[] args)
Serializable Interface
In this homework, you will also work with the idea of persistence. This means that our program should save all data from session to session. When we terminate a program, normally the data will be lost. We will preserve this data by using Serializable Java API and binary object files. All your classes should simply implement the java.io.Serializable interface (with the exception of the LunarSystem class)
Example: (Note - class names here intentionally are different than the homework description above)
To serialize an object, you need to create an ObjectOutputStream to send the data to, and then use the writeObject method to send the data to the stream, which is stored in the specified file. In the following code snippet, we have an instance of the StorageTable class that implements the Serializable Interface.
StorageTable storage = new StorageTable(/*Constructor Parameters*/);
// missing code here adds Storage objects to the table.
FileOutputStream file = new FileOutputStream("storage.obj");
ObjectOutputStream outStream = new ObjectOutputStream(file);
// the following line will save the object in the file
outStream.writeObject(storage);
outStream.close();
When the same application (or another application) runs again, you can initialize the member using the serialized data saved from step 1 so you don't have to recreate the object from scratch. To do this, you need to create an ObjectInputStream to read the data from, and then use the readObject method to read the hash from the stream:
FileInputStream file = new FileInputStream("storage.obj");
ObjectInputStream inStream = new ObjectInputStream(file);
StorageTable storage;
storage = (StorageTable) inStream.readObject();
inStream.close();
// missing code here can use StorageTable constructed previously
Note: If you change any data fields or structure of the serialized class, old saved objects will become incompatible.
UI Required Functions
L-Login/Logout
WebID or REGISTRAR to login as registrar
Student Options:
A-Add A Class
Department
Course Number
Semester (S or F followed by a number 2010-2025)
D-Drop A Class
Department
Course Number
C- List classes by Department
S-List Classes by semester
Registrar Option
R-Register A Student
D-De-Register A Student
E-View students enrolled in a class
Department
Course Number
General options (available when logged out only)
X-Save and quit
Q-Quit without saving state (and delete any save-file)
Note: please make sure that the menu is NOT case sensitive (so selecting A should be the same as selecting a).
Program Sample
Welcome to the Lunar System, a second place course registration system for second rate courses at a second class school.
No previous data found.
Menu:
L)Login
X)Save state and quit
Q)Quit without saving state.
Please select an option: L
Please enter webid: REGISTRAR
Welcome Registrar.
Options:
R) Register a student
D) De-register a student
E) View course enrollment
L) Logout
Please select an option: R
Please enter a webid for the new student: CWolf
CWolf registered.
Please select an option: R
Please enter a webid for the new student: SStanleyJrMD
SStanleyJrMD registered.
Please select an option: R
Please enter a webid for the new student: SStanleyJrMD
SStanleyJrMD is already registered.
Please select an option: R
Please enter a webid for the new student: MBashtook
MBashtook registered.
Please select an option: L
Registrar logged out.
Menu:
L)Login
X)Save state and quit
Q)Quit without saving state.
Please select an option: L
Please enter webid: mbashtook
Welcome MBashtook.
Options:
A)Add a class
D)Drop a class
C)View your classes sorted by course name/department
S)View your courses sorted by semester
Please select an option: A
Please enter course name: CSE 114
Please select a semester: F2017
CSE 114 added in Fall 2017.
Please select an option: A
Please enter course name: CSE 214
Please select a semester: S2018
CSE 214 added in Spring 2018.
Please select an option: A
Please enter course name: CSE 220
Please select a semester: F2018
CSE 220 added in Fall 2018.
Please select an option: A
Please enter course name: WRT 102
Please select a semester: S2018
WRT 102 added in Spring 2018.
Please select an option: A
Please enter course name: AMS 210
Please select a semester: S2018
AMS 210 added in Spring 2018.
Please select an option: C
Dept. Course Semester
-------------------------------
AMS 210 S2018
CSE 114 F2017
CSE 214 S2018
CSE 220 F2018
WRT 102 S2018
Please select an option: S
Dept. Course Semester
-------------------------------
CSE 114 F2017
AMS 210 S2018
CSE 214 S2018
WRT 102 S2018
CSE 220 F2018
Please select an option: L
MBashtook logged out.
Menu:
L)Login
X)Save state and quit
Q)Quit without saving state.
Please select an option: L
Please enter webid: SStanleyJrMd
Welcome SStanleyJrMd.
Options:
A)Add a class
D)Drop a class
C)View your classes sorted by course name/department
S)View your courses sorted by semester
Please select an option: A
Please enter course name: WRT 102
Please select a semester: S2018
WRT 102 added in Spring 2018.
Please select an option: A
Please enter course name: AMS 210
Please select a semester: S2018
AMS 210 added in Spring 2018.
Please select an option: A
Please enter course name: BIO 203
Please select a semester: S2018
BIO 203 added in Spring 2018.
Please select an option: C
Dept. Course Semester
-------------------------------
AMS 210 S2018
BIO 203 S2018
WRT 102 S2018
Please select an option: L
SStanleyJrMd logged out.
Menu:
L)Login
X)Save state and quit
Q)Quit without saving state.
Please select an option: X
System state saved, system shut down for maintenance.
//Program is restarted by user.
Welcome to the Lunar System, a second place course registration system for second rate courses at a second class school.
Previous data loaded.
Menu:
L)Login
X)Save state and quit
Q)Quit without saving state.
Please select an option: L
Please enter webid: cwolf
Welcome CWolf.
Options:
A)Add a class
D)Drop a class
C)View your classes sorted by course name/department
S)View your courses sorted by semester
Please select an option: A
Please enter course name: CSE 114
Please select a semester: F2018
CSE 114 added in Fall 2018.
Please select an option: A
Please enter course name: CSE 214
Please select a semester: S2018
CSE 214 added in Spring 2018.
Please select an option: A
Please enter course name: CSE 220
Please select a semester: F2018
CSE 220 added in Fall 2018.
Please select an option: L
CWolf logged out.
Menu:
L)Login
X)Save state and quit
Q)Quit without saving state.
Please select an option: L
Please enter webid: REGISTRAR
Welcome Registrar.
Options:
R) Register a student
D) De-register a student
E) View course enrollment
L) Logout
Please select an option: E
Please enter course: CSE 114
Students Registered in CSE 114:
Student Semester
-------------------
CWolf S2018
MBashtook S2017
Please select an option: D
Please enter a webid for the student to be deregistered: CWolf
CWolf deregistered.
Please select an option: E
Please enter course: CSE 114
Students Registered in CSE 114:
Student Semester
-------------------
MBashtook S2017
Please select an option: L
Registrar logged out.
Menu:
L)Login
X)Save state and quit
Q)Quit without saving state.
Please select an option: Q
Good bye, please pick the right SUNY next time!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
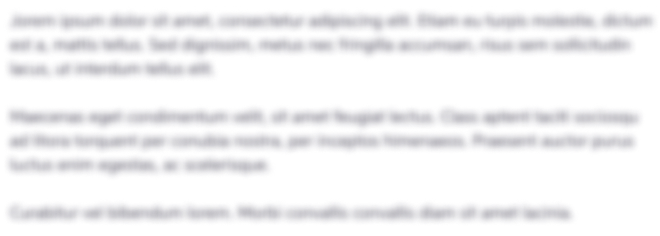
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started