Question
. The user is required to guess letters for a randomly selected word similar to the Wheel of Fortune television game show. The game utilizes
. The user is required to guess letters for a randomly selected word similar to the Wheel of Fortune television game show. The game utilizes data that is stored in a file named words1.txt. This file is the same as used in lab10in and consists of 1149 unique words placed on each line as shown below:
ability
account
accumulated
accumulating
accuracy
..
windows
within
wollborn
workshop
workshops
yellow
The same set of images used in lab10in that corresponds to the alphabet is provided in alpha.zip. Examples of the images are shown below:
a.bmp b.bmp y.bmp z.bmp - Each letter is 32.x32
The following class, modified from lab10in, Game is used when implementing this lab.
#ifndef GAME_H
#define GAME_H
#include "GenPoint.h"
class Game
{
private:
char* fileName;
char* word;
int* bananas; //Array of object numbers for each banana image
GenPoint* keys_ul; //Array of GenPoint for upper left coordinates
GenPoint* keys_lr; //Array of GenPoint for lower right coordinates
public:
Game();
~Game();
char* getFileName();
void setFileName(char* fn);
void setWord(int rand_no);
char* getWord();
void draw();
void play();
};
#endif
The guidelines for main involve the following steps:
1. Declare Game object
2.Prompt the user for the file name containing the words to be processed.
3. Invoke setFileName method that sets the fileName private data field.
4. Generate random number between 0 and 1148
5. Invoke setWord method that selects a random word from the file and sets the private data field word.
5. Invoke the draw method that displays the banana tree, bananas, text messages, a colored line, blank rectangles, and a virtual keyboard consisting of the alphabetic images.
6. Invoke the play method that allows the user to play the game by selecting letters. The specific rules of the game are given in a subsequent section.
The example prompt from main is shown below:
The resulting graphics display as created from the draw method is shown:
|
|
The draw method displays the initial scene for the game. The elements of this initial scene are created by the draw method as outlined below:
1. The randomly selected word for this example has 7 letters, thus 7 white rectangle are displayed starting from (100,250) each with width of 32 and height of 32.
2. A banana for each letter of the randomly selected word is also displayed in the banana tree - 7 bananas for this example - although only 2 bananas are visible. Each banana is numbered from 0 to N-1, where N is the length of the word. Each even numbered banana is displayed at (125,65) using the image banana.bmp. Each odd numbered banana is displayed at (132,65) using the image banana1.bmp. Banana images are therefore stacked on top of each other - only displaying the most recently displayed odd/even banana.
3. The text information indicating the number of bananas is shown at (400,100).
4. The banana_tree.bmp image is displayed at (100,0)
5. The virtual keyboard consists of 26 images - a.bmp thru z.bmp. Each image has the dimensions of 32x32. The image a.bmp is displayed at (100,300). Each subsequent image is displayed with an offset of 33 in the x direction.
Playing the Game
The user can begin playing the Falling Banana Game after the initial scene for the game is displayed by invoking the draw the scene. The user plays the game by selecting (i.e., clicking) on letters of the virtual keyboard. For example, suppose the user clicks on the E button. The resulting display is shown below:
The letter of E of the virtual keyboard is grayed out indicating that it cannot be used again. All occurrences of the letter E within the randomly selected word are displayed at the corresponding white rectangles. The letter E occurs in 2 locations for the example given above. The example word begins with the letter E and has the letter E in the 6 position. Suppose the user clicks on the letter A as shown below:
|
The letter A is not found in the word. The last banana added - #6 - falls from the even (left) side as shown above. Use moveObject in order to make the banana fall. The letter A is also grayed out. The banana is removed once it reaches the ground (y-coordinate = 175) and the number of bananas remaining is equal to 6. The resulting display once the banana reaches the ground is shown below:
|
Note the total number of bananas has been decremented by 1. Suppose the user clicks on the letter Z. Z was not found in the word, and the banana falls from the odd (right) side this time as shown below:
Once the banana has fallen to the ground (y-coordinate = 175), the number of bananas is again decremented as shown below:
The game continues as the player clicks on the letters that he believes are in the word. The results for clicking on C,N,H,I,U,W,T,R are shown below:
Note only banana is left because the user has incorrectly guessed 6 letters. Only one letter remains in the word as well. Suppose the user guesses the letter S as shown below:
The player is also prompted to play the game again as shown below:
Upon entering y, the console is cleared along with the graphics window. A new randomly selected word is selected and the draw method displays the following scene:
The user plays the game as before. Suppose the user cannot guess the word as shown below: (only 1 banana left)
If the player clicks on E, the last banana falls and the following is displayed:
The user can then exit the program by entering n.
Data Field Description
char* fileName;
The name of the file containing the words used by the game. For our lab, it will be words1.txt.
char* word;
The randomly selected word from the file containing the word. Set by the
void setWord(int rand_no);
method.
int* bananas; //Array of object numbers for each banana image
Dynamically allocated array of integers containing the object number for each displayed banana. This array should be allocated and set by the draw method.
GenPoint* keys_ul; //Array of GenPoint for upper left coordinates
A dynamically allocated array of GenPoints containing the upper left coordinate for each displayed image (i.e., letter) of the virtual keyboard. Useful for detecting which letter was pressed. Parallel array with keys_lr. This array should be allocated and initialized by the draw method.
GenPoint* keys_lr;
A dynamically allocated array of GenPoints containing the lower right coordinate for each displayed image (i.e., letter) of the virtual keyboard. Useful for detecting which letter was pressed. Parallel array with keys_ul. This array should be allocated and initialized by the draw method.
Methods Description
Game();
Default Constructor. Sets datafields to NULL.
char* getFileName();
Getter for fileName field.
void setFileName(char* fn);
Setter for fileName field.
void setWord(int rand_no);
Setter for word data field. This method performs the following steps.
1. Opens a file associated with the fileName data field for reading (input)
2. Tests the file stream to assure it is opened correctly
3. Reads one word at a time from the file
4. Accumulates a counter for the number of words in the file
5. Stops reading once the counter is equal to input parameter rand_no
6. Assigns the word data field to randomly selected word
char* getWord();
Getter for the word data field.
void draw();
Draws the initial scene for a randomly selected word as shown on page 3.
void play();
Allows the user to play the Falling Banana Game based on the rules/scenarios outlined on pages 4 thru 10. The user plays the games by performing the following steps:
1. The player guesses letters in the word by clicking on images (i.e., letters) of the virtual keyboard.
2. Display all occurrences of the clicked letter in the corresponding white rectangles located above the virtual keyboard.
3. A banana is dropped from the tree for each guessed letter that is not found.
4. The player looses once the total number of bananas in the tree is 0.
5. The player wins if he correctly guesses all letters in the word.
What you Submit
Submit all source code via Blackboard.
Guidelines
You will be graded according to the following guidelines:
You are required to submit all source (.cpp) files via Blackboard.
Your source file should be adequately commented and must contain the required header at its top.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
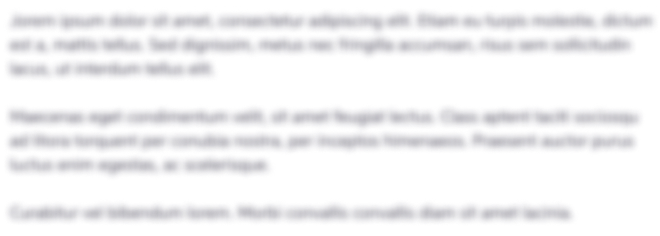
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started