Question
There are files of the c++ for the object oriented programming please explain it in detail about how it works. There are total three files.
There are files of the c++ for the object oriented programming please explain it in detail about how it works. There are total three files. Explain each file in detail on how it is working File 1: Department.h
#ifndef SDDS_DEPARTMENT_H_ #define SDDS_DEPARTMENT_H_ namespace sdds {
const int MAX_TOPIC_LENGTH = 25;
struct Project { char m_topic[MAX_TOPIC_LENGTH + 1]; double m_cost; };
class Department { char* deptName = nullptr; Project* prj = nullptr; int numPrj = 0; double bdgt = 15345.99;
public:
void updateName(const char* newname); void updateBudget(double change); bool addProject(Project& newproject); void createDepartment(const char* newname, Project& newproject, double change);
Project* fetchProjects() const { return prj; };
int fetchNumProjects() const { return numPrj; };
double fetchBudget() const { return bdgt; };
char* fetchName() const { return deptName; };
double totalexpenses() { double sum = 0; for (int i = 0; i < numPrj; i++) { sum += prj[i].m_cost; } return sum; };
double remainingBudget() { return bdgt - totalexpenses(); };
void clearDepartment() { delete[] deptName; delete[] prj; numPrj = 0; } };
void display(const Project& project); void display(const Department& department);
} #endif // !SDDS_DEPARTMENT_H_ File 2 : Department.cpp
#define _CRT_SECURE_NO_WARNINGS #include
#include
#include "Department.h"
using namespace std;
namespace sdds {
void Department::updateName(const char* newname) { if (!newname) return;
if (deptName) { delete[] deptName; }
const size_t strsize = strlen(newname) + 1; deptName = new char[strsize]; strncpy(deptName, newname, strsize); }
void Department::updateBudget(double change) { bdgt += change; }
bool Department::addProject(Project& project) { if (remainingBudget() < project.m_cost) return false;
if (!prj) { numPrj = 1; prj = new Project[numPrj]; prj[0] = project; return true; }
Project* tmparr = new Project[numPrj + 1]; for (int i = 0; i < numPrj; i++) { tmparr[i] = prj[i]; }
tmparr[numPrj] = project;
delete[] prj; numPrj++;
prj = new Project[numPrj]; for (int i = 0; i < numPrj; i++) { prj[i] = tmparr[i]; }
delete[] tmparr; return true; }
//creating the department void Department::createDepartment(const char* newname, Project& newproject, double change) { updateName(newname); addProject(newproject); updateBudget(change); }
void display(const Project& project) { cout << "Project " << project.m_topic << " will cost a total of " << project.m_cost << " C$." << endl; }
//displaying the department void display(const Department& department) { Project* temp = department.fetchProjects(); int projects = department.fetchNumProjects(); cout << "Department " << department.fetchName() << " details:" << endl; cout << "Budget: " << department.fetchBudget() << " and the number of projects in progress is: " << projects << endl; cout << "Below are the details of the projects we are currently working on: " << endl; for (int i = 0; i < projects; i++) { display(temp[i]); } } } File 3: main.cpp
#include
int main() {
Project testProject = { "Base",551.55 };
Project myprojects[5] = { {"Synergy", 5041.55}, {"Mecha", 2925.99}, {"Chroma", 3995.45}, {"Force", 6988.45}, {"Oculus", 7441.22} };
Department SDDS{};
//Test1 cout << "**********Create Department TEST START**********" << endl; SDDS.createDepartment("School of Software development", testProject, 1); display(SDDS); cout << "**********Create Department TEST END**********" << endl;
//Test2 cout << endl << "**********Update Name TEST Start**********" << endl; SDDS.updateName("School of Software development and design"); display(SDDS); cout << "**********Update Name TEST END**********" << endl;
//Test3 cout << endl << "**********Update Budget TEST Start**********" << endl; SDDS.updateBudget(5555.99); display(SDDS); cout << "**********Update Budget TEST END**********" << endl;
//Test4 cout << endl << "**********Expenses and Remaining Budget TEST Start**********" << endl; cout << "Our current total Expenses are: " << SDDS.totalexpenses() << endl; cout << "Our remaining budget is: " << SDDS.remainingBudget() << endl; cout << "**********Expenses and Remaining Budget TEST END**********" << endl;
//Test5 cout << endl << "**********Add Project TEST START**********" << endl; for (int i = 0; i < 5 && SDDS.addProject(myprojects[i]); i++); display(SDDS); cout << "**********Add Project TEST END**********" << endl;
SDDS.clearDepartment(); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
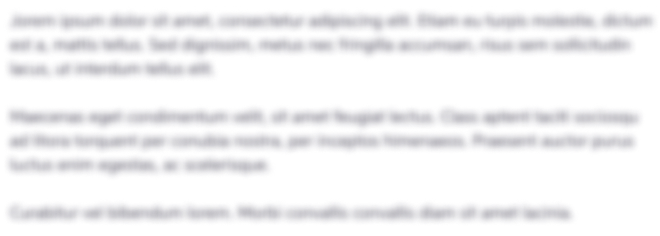
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started