Answered step by step
Verified Expert Solution
Question
1 Approved Answer
there is also some starter code: complex.h : #ifndef COMPLEX_H #define COMPLEX_H #include using namespace std; /* YOUR TASK HERE IS TO GIVE THE COMPLETE
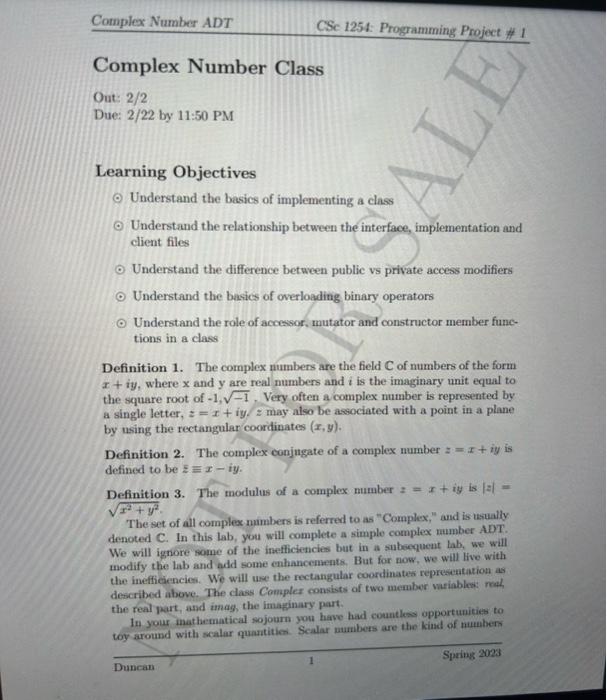
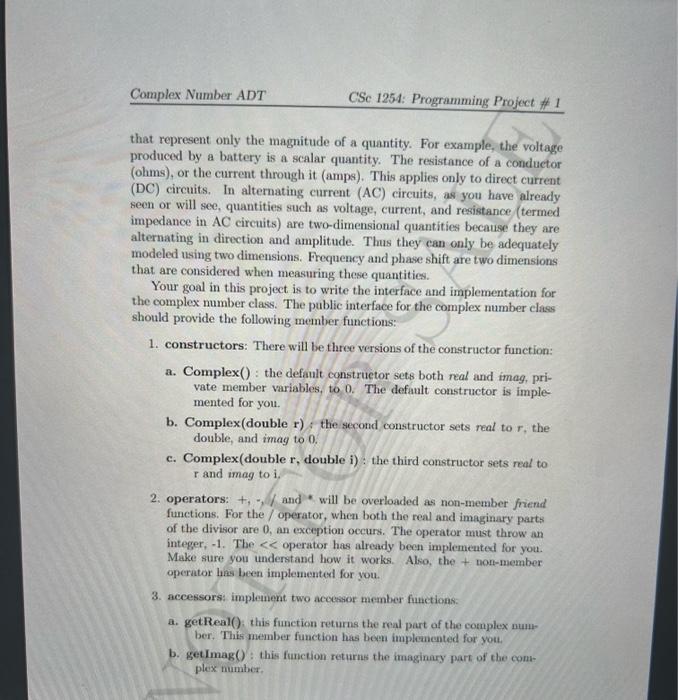
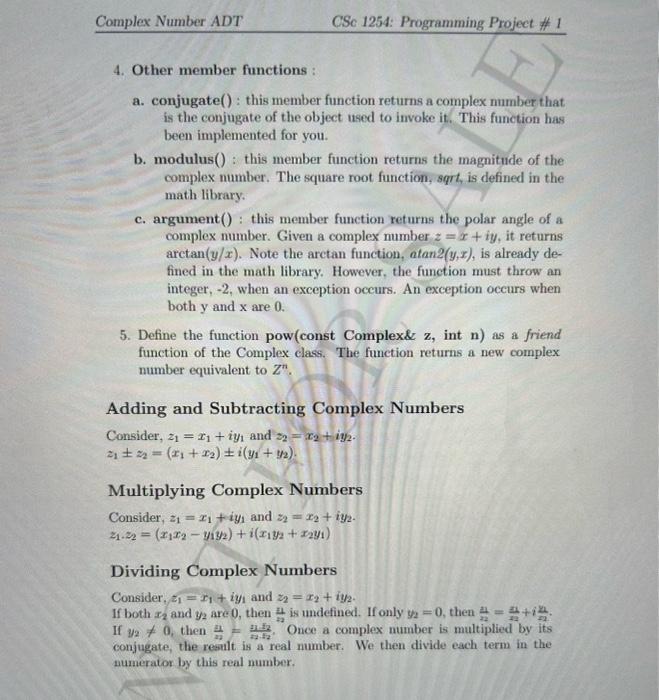
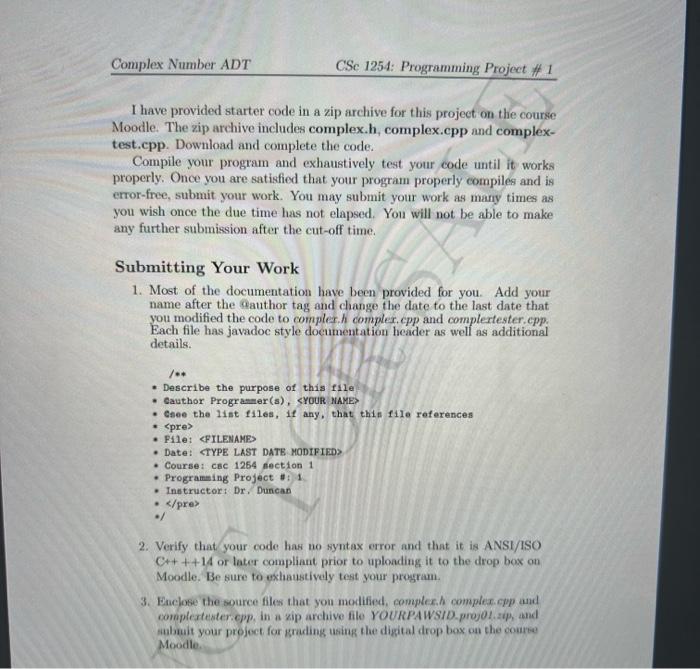
there is also some starter code:
complex.h :
#ifndef COMPLEX_H
#define COMPLEX_H
#include
using namespace std;
/* YOUR TASK HERE IS TO GIVE THE COMPLETE DEFINITION OF
THE class Complex AS DESCRIBED IN THE PRJECT HANDOUT.
BE SURE TO INCLUDE A DESCRIPTION OF EACH OF THE FUNCTIONS.
YOU WILL PROVIDE ONLY THE PUBLIC INTERFACE OF THE MEMBER
AND FRIEND FUNCTIONS, NOT THEIR DEFINITIONS.
THE MEMBER AND FRIEND FUNCTIONS WILL BE DEFINED IN THE
IMPLEMENTATION FILE.
DEFINE THE CLASS BELOW.
*/
#endif
complex.cpp:
#include
#include
#include "complex.h"
/* SOME FUNCTION HAVE BEEN IMPLEMENTED FOR YOU. IMPLEMENT
ALL OTHER FUNCTIONS DESCRIBED ON THE PROJECT HANDOUT.
WHOSE PROTOTYPES APPEAR IN THE CLASS.
*/
Complex::Complex()
{
real = 0.0;
imag = 0.0;
}
double Complex::getReal() const
{
return real;
}
Complex Complex::conjugate() const
{
return Complex(real,-imag);
}
Complex operator +(const Complex& z1, const Complex& z2)
{
return Complex(z1.real+z2.real,z1.imag+z2.imag);
}
ostream& operator
{
if (z.real == 0 && z.imag == 0)
{
out
return out;
}
if (z.real == 0)
{
if (z.imag
{
if (z.imag != -1)
out
else
out
}
else
{
if (z.imag != 1)
out
else
out
}
return out;
}
if (z.imag == 0)
{
out
return out;
}
out
if (z.imag
{
if (z.imag != -1)
out
else
out
}
else
{
if (z.imag != 1)
out
else
out
}
return out;
}
complextest:
#include
#include "complex.h"
#include
using namespace std;
int main(int argc, char **argv)
{
Complex z0(3.6,4.8);
Complex z1(4,-2);
Complex z2(-4,2);
Complex z3(-4,-3);
Complex z4(3,-4);
Complex z5;
Complex z6;
Complex z7;
double ang;
cout
cout
cout
cout
cout
cout
cout
cout
cout
cout
cout
cout
cout
cout
cout
try
{
cout
z6 = ((z2+z3)*(z3-z4))/z3;
cout
cout
cout
cout
cout
cout
cout
cout
cout
/* Add statement here to compute ((z1-z2).(z3/z4))/(z4/z3)
and print the result:*/
ang = (z1+z2).argument();
cout
/* Put a comment here explaining what happened and why?
*/
}
catch(int e)
{
if (e == -1)
cerr
Complex Number Class Out: 2/2 Due: 2/22 by 11:50 PM Learning Objectives Understand the basics of implementing a class Understand the relationship between the interface, implementation and client files Understand the difference between public vs private access modifiers Understand the basics of overloading binary operators Understand the role of accessor, mutator and constructor member functions in a class Definition 1. The complex numbers are the field C of numbers of the form x+iy, where x and y are real numbers and i is the imaginary unit equal to the square root of 1,1. Very often a complex number is represented by a single letter, z=x+iy. z may also be associated with a point in a plane by using the rectangular coordinates (x,y). Definition 2. The complex conjugate of a complex number z=x+iy is defined to be xiy. Definition 3. The modulus of a complex number z=x+ it is z= x2+y2. The set of all complex numbers is referred to as "Complex," and is usually denoted C. In this lab, you will complete a simple complex number ADT. We will ignore some of the inefficiencies but in a subequent lab, we will modify the lab and add some enhancements. But for now, we will live with the inefficiencies. We will use the rectangular coordinates representation as described above. The class Compler consists of two member variables: neul, the real part, and imag, the imaginary part. In your mathematical sojourn you have had conntkos opportunities to toy around with scalar quantities. Sealar numbers are the kind of numbers that represent only the magnitude of a quantity. For example, the voltage produced by a battery is a scalar quantity. The resistance of a conductor (ohms), or the current through it (amps). This applies only to direct current (DC) circuits. In alternating current (AC) circuits, as you have already seen or will see, quantities such as voltage, current, and resistance (termed impedance in AC circuits) are two-dimensional quantities because they are alternating in direction and amplitude. Thus they can only be adequately modeled using two dimensions. Frequency and phase shift are two dimensions that are considered when measuring these quantities. Your goal in this project is to write the interface and implementation for the complex number class. The public interface for the complex number class should provide the following member functions: 1. constructors: There will be three versions of the constructor function: a. Complex () : the default constructor sets both real and imag, private member variables, to 0 . The default constructor is implemented for you. b. Complex(double r ) the second constructor sets real to r, the double, and imag to 0 . c. Complex(double r, double i) : the third constructor sets real to r and imag to i. 2. operators: +,+/ and " will be overloaded as non-member friend functions. For the / operator, when both the real and imaginary parts of the divisor are 0 , an exception occurs. The operator must throw an integer, 1. The operator has already been implemented for you. Make sure you understand how it works. Also, the + non-member operator has been implemented for you. 3. accessors: implement two acoessor member functions: a. getReal(): this function returns the real part of the complex number. This member function has been implemented for you. b. getImag() : this function returns the imaginary part of the complex number. 4. Other member functions : a. conjugate() : this member function returns a complex number that is the conjugate of the object used to invoke it. This function has been implemented for you. b. modulus () : this member function returns the magnitude of the complex number. The square root function, sqrt, is defined in the math library. c. argument () : this member function returns the polar angle of a complex number. Given a complex number z=x+iy, it returns arctan(y/x). Note the arctan function, atan2(y,x), is already defined in the math library. However, the function must throw an integer, -2, when an exception occurs. An exception occurs when both y and x are 0 . 5. Define the function pow (const Complex\& z, int n ) as a friend function of the Complex class. The function returns a new complex number equivalent to Zn. Adding and Subtracting Complex Numbers Consider, z1=x1+iy1 and z2=x2+iy2. z1z2=(x1+x2)i(y1+y2). Multiplying Complex Numbers Consider, z1=x1+iy1 and z2=x2+iy2. z1z2=(x1x2y1y2)+i(x1y2+x2y1) Dividing Complex Numbers Consider, z1=x1+iy1 and z2=x2+iy2. If both x2 and y2 are 0 , then 27 is undefined. If only y2=0, then 2a=x2a+i222. If y2=0, then 4a=2723.z2, Once a complex number is multiplied by its conjugate, the result is a real number. We then divide each term in the numerator by this real number. I have provided starter code in a zip archive for this project on the course Moodle. The zip archive includes complex.h, complex.cpp and complextest.cpp. Download and complete the code. Compile your program and exhaustively test your code until it works properly. Once you are satisfied that your program properly compiles and is error-free, submit your work. You may submit your work as many times as you wish once the due time has not elapsed. You will not be able to make any further submission after the cut-off time. Submitting Your Work 1. Most of the documentation have been provided for you. Add your name after the Qauthor tag and change the date to the last date that you modified the code to compler h compler, cpp and complextester,.pp. Each file has javadoc style doeumentation header as well as additional details. - Describe the purpose of this file - Cauthor Progranmer(s), SYOUR MAYE> - Csee the list 111 es, if any, that this file references - pre> - File: QFILENAME - Date: TYPE LAST DATE MODIFIED? - Course: ces 1254 mection 1 - Programing Project \#t 1. - Inetructor: Dr. Duncas - / pre i) 2. Verify that your code has no syntax error and that it is ANSI/ISO C++++14 or later compliant prior to uploading it to the drop box on Moodle. Be sure to exhaustively test your program. 3. Eaclose the source files that you modified, compler.h complex cpp and complecteater. CP, in a zip archive file YOURPAWSID. projol.zip, and aubuit your project for grading using the digital drop box on the course Moodle else if (e == -2)
cerr
}
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
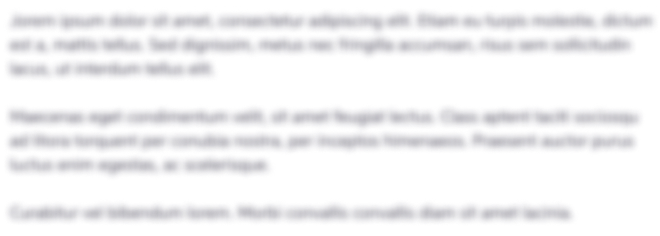
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started