Question
This assignment covers both Unit 2 (Classes and Objects, Part 1) and Unit 3 (Classes and Objects, Part 2). You will submit it under the
This assignment covers both Unit 2 (Classes and Objects, Part 1) and Unit 3 (Classes and Objects, Part 2). You will submit it under the Unit 3 homework link.
In this assignment you will practice creating classes and enumerations in the context of a larger system. Overall, we are building pieces of a bus route booking system that allows passengers to book travel buses that travel throughout the United States. The classes that we are constructing in this assignment will take care of representing the bus data in Java. The final booking system should be able to search for bus routes from a list of commercial bus types (e.g. BoltBus, Greyhound), and locate various routes between two bus stations. Don't panic! We will go through this system step by step. For the sake of simplifying the problem, we will make a couple of assumptions:
There in only one timezone.
All bus routes occur in the same day.
Already provided for you is a file called Driver.java. A "driver" is a common term for the main program that manages a set of objects, and manipulates them. It contains the entry point (main) for an entire project. The file you have been provided contains five static methods that can be called from main. These are testing methods that will run sample operations on the enumerations and classes you will be constructing. Initally, they are all commented out. As you implement parts of the assignment, you should uncomment lines in the test methods, in order to see how your program behaves. Also attached is the file sample_output.txt. This file contains the sample output for running this Driver with all of the included tests. You should check the output of your program against the sample output. Plan to test your files one at a time, for example: make sure to test your BusType and BusStation enumerations before you start on Bus. Don't start working on Bus until those two classes work completely. Time is independent of BusType, BusStation, and Bus, but is complicated enough that you'll want the experience of implementing those other questions before you start it.
Q1: Create a BusType enumeration. This is a datatype that represents bus types in our system. Include three values: Greyhound, BoltBus, and Megabus. This should be a single file containing the enum declaration. [2 points]
Q2: Create a smart BusStation enumeration. This is a datatype that represents bus stations in our system, and has the ability to change from bus station code and city name. Include five values: PHX, LAX, LVS, SAN, and SFO. Also add a static method called getBusStationCity. This should be a single file containing the enum declaration, with the method declaration nested inside. (Hint: The attached file, ExampleSmartEnum.java,contains an example of including a method into an enumeration.) [8 points]
getBusStationCity(...) will take a BusStation enumeration value as a parameter, and return a String containing the city where that bus station is located (e.g., "Phoenix", "Las Vegas", "San Diego", "San Francisco"). Use a switch statement. Have a default case that returns "Unknown City".
Q3: Create a Bus class. This class will represent a bus in our system. It should contain a constructor, an instance variable (a BusType enumeration) and two methods (getBusType, toString) [10 points]
The constructor will take a BusType to set up the BusType enumeration.
getBusType(): will return the BusType of the bus (e.g., BusType.Greyhound).
toString(): will return the name of the bus's bus type (e.g., "Greyhound"), followed by a star (" * ").
Q4: Create a Time class. This class will represent a point in time, such as a departure time. It should contain 2 constructors, 2 instance variables (hour and minute), and 10 methods (see below). All methods but toString should be in terms of the 24 hour format. [30 points]
default constructor: Creates a Time object for 12:00AM.
overloaded constructor: Creates a Time object at a specific hour and minute.
getHour(): Returns an integer representing the hour of the Time object.
getMinute(): Returns an integer representing the minute of the Time object.
addHours(...): Updates the object by moving it forward a number of hours.
addMinute(...): Updates the object by moving it forward a number of minutes. (Hint: Be careful that you don't allow minutes to be more than 59.)
addTime(...): Updates the object by moving it forward by the hour and minute from another Time object.
getCopy(): Returns a new Time object that has the same hour and minute of the existing Time object.
isEarlierThan(...): Returns true if this Time object is earlier than another Time object.
isSameTime(...): Returns true if this Time object is the same as another Time object.
isLaterThan(...): Returns true if this Time object is later than another Time object.
toString(): Returns a string representing the Time object. Uses 12 hour AM/PM format and pads minutes to be two digits. See the sample output for an example.
Q5:
Create a BusRoute class that uses the Bus and Time class. This class will represent a bus route between two bus stations, using a specific Bus, and departing at a specific Time. It should contain a constructor, 7 instance variables (bus, bus number, cost, departure, duration, source, destination), and 9 methods (see below). [25 points]
overloaded constructor: Creates a BusRoute object that is setup up with a Bus, a bus number, a cost, a departure Time, a duration time, a source BusStation, and a destination BusStation.
getBus(): Returns the Bus that operates this bn rusoute.
getNumber(): Returns the bus number as a String.
getCost(): Returns the bus route cost.
getDestination(): Returns the destination BusStation.
getDeparture(): Returns the departure Time.
getArrival(): Returns a Time object with the arrival time (computed from the departure time and duration).
getSource(): Returns a Bus Station object for the departure location.
toOverviewString(): Returns a String representing an overview of the bus route. Use NumberFormat to display the price. See the sample output for an example.
toDetailedString(): Returns a String representing the bus route's detailed information. See the sample output for an example.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
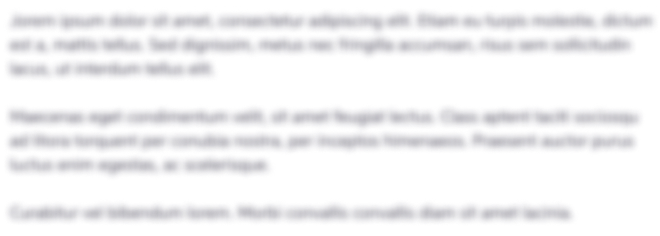
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started