Question
This assignment must be done in Python3. This assignment must be done in 2 phases. The objective of this assignment is to design and implement
This assignment must be done in Python3. This assignment must be done in 2 phases.
The objective of this assignment is to design and implement a simple file transfer application that provides reliable service, using the unreliable UDP datagrams. As a minimum, your application must account for packet loss, delay, corruption, duplication, and re-ordering.
Protocol Description:
This assignment uses a simple protocol that has four message types: start, end, data, and ack.
start, end, and data messages all follow the same general format. Using an OOP approach, create message objects that contain the following information:
type: string start, data, end, ack
sequence number: integer
data: bytes
checksum: unsigned integer
To initiate a connection, send a start message. The receiver will use the sequence number provided as the initial sequence number for all packets in that connection. After sending the start message, send additional packets in the same connection using the data message type, adjusting the sequence number appropriately. Unsurprisingly, the last message in a connection should be transmitted with the end message type to signal the receiver that the connection is complete. Your sender should accept acknowledgements from the receiver using the ack message type. The ack message is the only message that will not have data.
An important limitation is the maximum size of your packets. The UDP protocol has an 8 byte header, and the IP protocol underneath it has a header of ~20 bytes. Because you will be using Ethernet networks, which have a maximum frame size of 1500 bytes, this leaves 1472 bytes for your entire packet (message type, sequence number, data, and checksum).
In this assignment, you will write a client and server application similar to FTP. The client connects to the server and can down-load any file accessible to the server application. Any number of files can be down-loaded and no access control is performed.
Receiver specification
The receiver responds to data packets with cumulative acknowledgements. Upon receiving a message of type start, data, or end, the receiver generates an ack message with the sequence number it expects to receive next, which is the lowest sequence number not yet received. In other words, if it expects a packet of sequence number N, the following two scenarios may occur:
1. If it receives a packet with sequence number not equal to N, it will send ack|N.
2. If it receives a packet with sequence number N, it will check for the highest sequence number (say M) of the in-order packets it has already received and send ack|M+1. For example, if it has already received packets N+1 and N+2 (i.e. M = N+2), but no others past N+2, then it will send ack|N+3.
You can assume that once a packet has been acknowledged by the sender, it has been properly received. The receiver should have a default timeout of 10 seconds; it will automatically close any connections for which it does not receive packets for that duration.
Sender specification
The sender should read an input file and transmit it to a specified receiver using UDP sockets. It should split the input file into appropriately sized chunks of data, specify an initial sequence number for the connection, and append a checksum to each packet. The sequence number should increment by the size of the data sent in the packet (see textbook for specification).
Your sender must implement a reliable transport algorithm (i.e. sliding window). The receivers window size should be set to five packets, and ignore more than this. Your sender must be able to accept ack packets from the receiver. Any ack packets with an invalid checksum should be ignored.
Your sender should provide the following reliable service under the following network conditions:
Loss: arbitrary levels; you should be able to handle periods of 100% packet loss.
Corruption: arbitrary types and frequency.
Re-ordering: may arrive in any order, and
Duplication: you could see a packet any number of times.
Delay: packets may be delayed indefinitely (but in practice, generally not more than 10s).
Your sender should be invoked with the following command: python3 Sender.py -f -a p (filename, ip address, and port) Some final notes about the sender:
The sender should implement a 500ms retransmission timer to automatically retransmit packets that were never acknowledged (potentially due to ack packets being lost). I do not expect you to use an adaptive timeout.
Your sender should support a window size of 5 packets (i.e., 5 unacknowledged packets).
Your sender should roughly meet or exceed the performance (in both time and number of packets required to complete a transfer) of a properly implemented sliding-window based sender.
Your sender should be able to handle arbitrary message data (i.e., it should be able to send an image file just as easily as a text file).
Any packets received with an invalid checksum should be ignored.
Your implementation must run on Windows or Unix system. However, it should work on any system that runs Python 3.x.
Your sender should be written in Python 3.5 or greater and adhere to the standards given in PEP 8, Style Guidelines for Python Code.
Your sender MUST produce console output during normal execution; Python exception messages are great, but try to avoid having your program crash in the first place. The console output should show when acks are received and messages sent. Also, if the server closes the session because of a timeout, a message should show on the console.
Use the Python library binascii.crc32() to create a checksum for your message.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
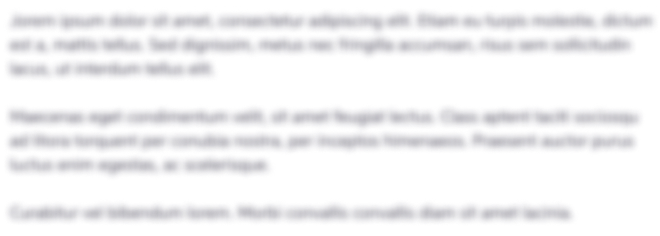
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started