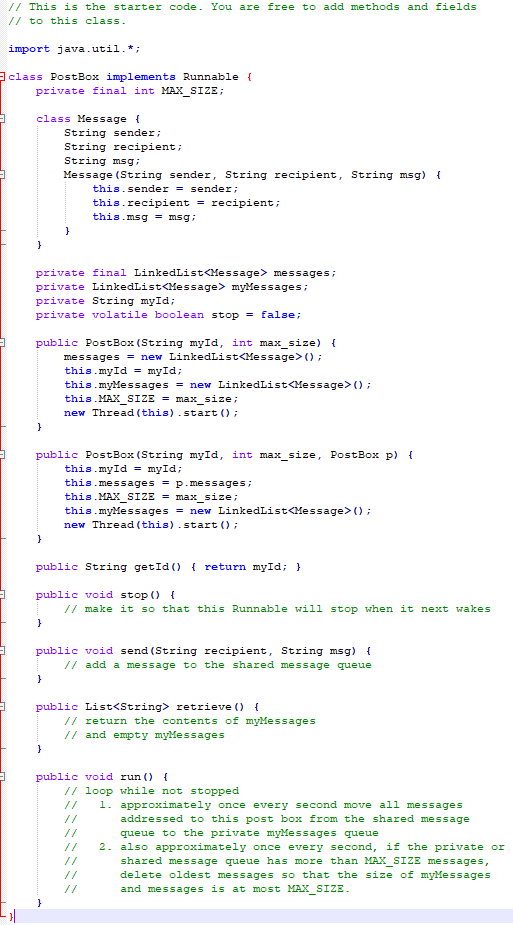
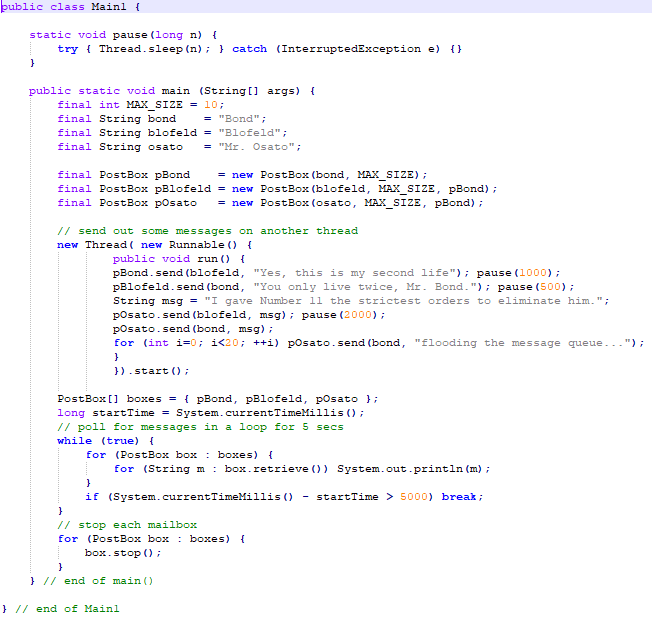
This is a representation of a messaging server implementation.
Finish the stop(),send(), retrieve(), and run() methods.
// This is the starter code. You are free to add methods and fields // to this class.
import java.util.*;
class PostBox implements Runnable { private final int MAX_SIZE;
class Message { String sender; String recipient; String msg; Message(String sender, String recipient, String msg) { this.sender = sender; this.recipient = recipient; this.msg = msg; } }
private final LinkedList messages; private LinkedList myMessages; private String myId; private volatile boolean stop = false;
public PostBox(String myId, int max_size) { messages = new LinkedList(); this.myId = myId; this.myMessages = new LinkedList(); this.MAX_SIZE = max_size; new Thread(this).start(); }
public PostBox(String myId, int max_size, PostBox p) { this.myId = myId; this.messages = p.messages; this.MAX_SIZE = max_size; this.myMessages = new LinkedList(); new Thread(this).start(); }
public String getId() { return myId; }
public void stop() { // make it so that this Runnable will stop when it next wakes }
public void send(String recipient, String msg) { // add a message to the shared message queue }
public List retrieve() { // return the contents of myMessages // and empty myMessages }
public void run() { // loop while not stopped // 1. approximately once every second move all messages // addressed to this post box from the shared message // queue to the private myMessages queue // 2. also approximately once every second, if the private or // shared message queue has more than MAX_SIZE messages, // delete oldest messages so that the size of myMessages // and messages is at most MAX_SIZE. } }
public class Main1 {
static void pause(long n) { try { Thread.sleep(n); } catch (InterruptedException e) {} }
public static void main (String[] args) { final int MAX_SIZE = 10; final String bond = "Bond"; final String blofeld = "Blofeld"; final String osato = "Mr. Osato";
final PostBox pBond = new PostBox(bond, MAX_SIZE); final PostBox pBlofeld = new PostBox(blofeld, MAX_SIZE, pBond); final PostBox pOsato = new PostBox(osato, MAX_SIZE, pBond);
// send out some messages on another thread new Thread( new Runnable() { public void run() { pBond.send(blofeld, "Yes, this is my second life"); pause(1000); pBlofeld.send(bond, "You only live twice, Mr. Bond."); pause(500); String msg = "I gave Number 11 the strictest orders to eliminate him."; pOsato.send(blofeld, msg); pause(2000); pOsato.send(bond, msg); for (int i=0; i
PostBox[] boxes = { pBond, pBlofeld, pOsato }; long startTime = System.currentTimeMillis(); // poll for messages in a loop for 5 secs while (true) { for (PostBox box : boxes) { for (String m : box.retrieve()) System.out.println(m); } if (System.currentTimeMillis() - startTime > 5000) break; } // stop each mailbox for (PostBox box : boxes) { box.stop(); } } // end of main()
} // end of Main1
// This is the starter code. You are free to add methods and fields // to this class. import java.util.*; class PostBox implements Runnable { private final int MAX_SIZE; class Message { String sender; String recipient; String msg; Message (String sender, String recipient, String msg) { this. sender = sender; this recipient = recipient; this.msg = msgi } private final LinkedList
messages; private LinkedList myMessages; private String myId; private volatile boolean stop = false; public PostBox(String myId, int max_size) { messages = new LinkedList(); this.myId = myId; this.myMessages = new LinkedList(); this.MAX_SIZE = max_size; new Thread(this).start(); } public PostBox(String myId, int max_size, PostBox p) { this.myId = myId; this messages = p. messages; this.MAX_SIZE = max_size; this.myMessages = new LinkedList(); new Thread(this) .start(); } public String getId() { return myId; } public void stop() { // make it so that this Runnable will stop when it next wakes } public void send (String recipient, String msg) { // add a message to the shared message queue } public List retrieve() { // return the contents of myMessages // and empty myMessages } public void run() { // loop while not stopped 1. approximately once every second move all messages // addressed to this post box from the shared message // queue to the private myMessages queue 2. also approximately once every second, if the private or shared message queue has more than MAX_SIZE messages, delete oldest messages so that the size of myMessages // and messages is at most MAX_SIZE. public class Mainl { static void pause (long n) { try { Thread sleep(n); } catch (InterruptedException e) {} } public static void main (String[] args) { final int MAX_SIZE = 10; final String bond = "Bond"; final String blofeld = "Blofeld"; final String osato = "Mr. Osato"; final PostBox pBond = new PostBox (bond, MAX_SIZE); final PostBox pBlofeld = new PostBox (blofeld, MAX_SIZE, pBond); final PostBox posato = new PostBox (osato, MAX_SIZE, pBond); // send out some messages on another thread new Thread( new Runnable() { public void run() { pBond send (blofeld, "Yes, this is my second life"); pause (1000); pBlofeld. send (bond, "You only live twice, Mr. Bond."); pause (500); String msg = "I gave Number ll the strictest orders to eliminate him."; posato send (blofeld, msg); pause (2000); posato send (bond, msg); for (int i=0; i 5000) break; } // stop each mailbox for (PostBox box : boxes) { box.stop(); } } // end of main() } // end of Mainl // This is the starter code. You are free to add methods and fields // to this class. import java.util.*; class PostBox implements Runnable { private final int MAX_SIZE; class Message { String sender; String recipient; String msg; Message (String sender, String recipient, String msg) { this. sender = sender; this recipient = recipient; this.msg = msgi } private final LinkedList messages; private LinkedList myMessages; private String myId; private volatile boolean stop = false; public PostBox(String myId, int max_size) { messages = new LinkedList(); this.myId = myId; this.myMessages = new LinkedList(); this.MAX_SIZE = max_size; new Thread(this).start(); } public PostBox(String myId, int max_size, PostBox p) { this.myId = myId; this messages = p. messages; this.MAX_SIZE = max_size; this.myMessages = new LinkedList(); new Thread(this) .start(); } public String getId() { return myId; } public void stop() { // make it so that this Runnable will stop when it next wakes } public void send (String recipient, String msg) { // add a message to the shared message queue } public List retrieve() { // return the contents of myMessages // and empty myMessages } public void run() { // loop while not stopped 1. approximately once every second move all messages // addressed to this post box from the shared message // queue to the private myMessages queue 2. also approximately once every second, if the private or shared message queue has more than MAX_SIZE messages, delete oldest messages so that the size of myMessages // and messages is at most MAX_SIZE. public class Mainl { static void pause (long n) { try { Thread sleep(n); } catch (InterruptedException e) {} } public static void main (String[] args) { final int MAX_SIZE = 10; final String bond = "Bond"; final String blofeld = "Blofeld"; final String osato = "Mr. Osato"; final PostBox pBond = new PostBox (bond, MAX_SIZE); final PostBox pBlofeld = new PostBox (blofeld, MAX_SIZE, pBond); final PostBox posato = new PostBox (osato, MAX_SIZE, pBond); // send out some messages on another thread new Thread( new Runnable() { public void run() { pBond send (blofeld, "Yes, this is my second life"); pause (1000); pBlofeld. send (bond, "You only live twice, Mr. Bond."); pause (500); String msg = "I gave Number ll the strictest orders to eliminate him."; posato send (blofeld, msg); pause (2000); posato send (bond, msg); for (int i=0; i 5000) break; } // stop each mailbox for (PostBox box : boxes) { box.stop(); } } // end of main() } // end of Mainl