Question
This is an assignment for java 2 and it is a DNA matcher program. I will post everything the assignment entails below. I cannot get
This is an assignment for java 2 and it is a DNA matcher program. I will post everything the assignment entails below. I cannot get things to work correctly and I have tried so many times.
This assignment addresses the following primary course learning objectives:
Writing recursive algorithms
Using recursive algorithms
Creating new Exceptions
Handling Exceptions
Package/library design and implementation
This assignment also provides you an opportunity to engage with the following objectives:
Document object-oriented designs using diagramming techniques and notations (e.g., UML)
Utilize graphical IDEs
Importance and limitations of testing during and after development
Write and review test cases and unit tests based on documented pre and post conditions
Write a recursive solution to a problem
Program Background
Recursion is an approach to implementing algorithms that can be restated as a different version of the same problem. Programming recursive algorithms requires writing a method or methods that either directly or indirectly call upon themselves. Recursive solutions must be defined in terms as bases cases and general, or recursive cases. Many problems can be easier to understand and implement with a recursive approach.
Program Description
DNA, or deoxyribonucleic acid, is a molecule that contains genetic instructions for living organisms. Each is composed of a sequence of four bases, adenine (A), cytosine (C), guanine (G), and thymine (T). These sequences can be represented by an ordered string of the symbols for the bases. When comparing two strands of DNA we can look at their longest common subsequence. The longer this string the closer the DNA strands are related, which is helpful for biologists trying to understand the underlying relationships between various genes.
Subsequences do not require the characters be adjacent in the string, only the order matters. Substrings are a subset of subsequences, as characters in substrings must be consecutive. The common substring of two strings is a string which appears in both strings. The longest common substring is the string out of these that has the most characters. For this problem we will only be concerned with the more restrictive substring.
In this program, the user will enter a DNA character sequence as the master sequence to be compared. They can then enter any number of additional candidate DNA sequences. For each of these candidate sequences entered it will check if its longest common substring with the master sequence is longer than any seen previously. If so, the master sequence will be updated with the value of its best match and the common substring. Once all candidates have been entered the program will report its findings.
The following classes are required in your solution. Each class name below is shown in fully-qualified form to illustrated the required package structure for your solution. Class name links provide Javadoc descriptions for the required data members (ie, fields in javadoc) and methods. Note that you are allowed, and some cases may need to, add additional helper methods and data members. When doing so, consider the level of access that these additional members require at a minimum.
Partially provided classes:
dna.DNADriver (main method provided, you must write the inputDNAString method)
Classes you must write:
dna.InvalidDNAStrandException
dna.DNA
dna.DNAMatcher (The rabinKarpHahses method MUST be recursive; non-recursive solutions will lose significant amount of points)
The UML diagram below shows the relationships of these classes including the package structure, association, and aggregation (has-a) relationships.
Lines with diamond shaped arrowheads indicate aggregation (has-a) relationships. Dashed lines with the normal arrowhead indicates a dependency caused by using a value of a given type somewhere in the code, but does not imply the existence of a has-a relationship. For example, the DNA class uses the InvalidDNAStrandException class in its definition, but not all DNA objects will have an InvalidDNAStrandException. In UML diagrams, it's easy to confuse dependency relationships with "implements" relationships on interfaces---pay careful attention to the shape of the arrowhead!
Details about data member and methods are hidden for readability, for this information refer to the javadoc links above.
Getting Started
Download, Unzip, and Import the HW3 Starter Project into your Eclipse workspace.
Once you've done this, you'll have the provided DNADriver in the dna package. From here, you can start writing the other required classes. There is no test file provided for this assignment, however creating one to incrementally test your classes is strongly suggested. You've seen some examples of test classes in the previous assignments; use these at templates to your testing approach. Some of the methods are private and therefore cannot be accessed outside of their class. You can either write publically accessable test methods in these classes, or temporarily make them public for testing purposes. If you do this be sure to change them back to private before submitting. Our grading script will test many of the individual methods of these classes.
Below is the partially provided driver file
package dna;
import java.util.Scanner;
/**
* Driver class for utilizing a DNA matcher.
* Retrieves Master DNA strand from user and as many match candidates as user wants to test.
* @author Mr. Cavanaugh
*
*/
public class DNADriver
{
/**
* Scanner used for user input.
*/
private static Scanner in = new Scanner(System.in);
//You must write the inputDNAString method. See DNADriver documentation for details.
//DO NOT make changes to main.
/**
* Entry point of execution. Runs DNA matching application.
* @param args Not used.
*/
public static void main(String[] args)
{
//Create DNA and DNAMatcher references
DNA master;
DNA candidate;
DNAMatcher matcher;
System.out.println("***Enter Master DNA***");
//Get DNA for the master strand
//Master DNA cannot be null, so continue until valid DNA is entered
do
{
master = inputDNAString();
}
while (master == null);
//Create the DNAMatcher with master DNA
matcher = new DNAMatcher(master);
System.out.println("***Enter DNA match candidates, EXIT to quit***");
//Accept candidate DNA until user quits
//inputDNAString will return null when "EXIT" is entered, so continue while null is not returned
while ((candidate = inputDNAString()) != null)
{
matcher.checkMatch(candidate);
}
//Output results!
System.out.println(" " + matcher);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
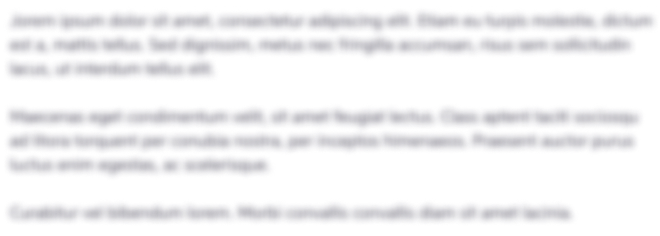
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started