Question
This is coded in C C++ in terminal..For this assignment, you will need to write three source code files as well as two header files.
This is coded in C C++ in terminal..For this assignment, you will need to write three source code files as well as two header files. Each of these files is relatively short, but many inexperienced programmers are overwhelmed by the idea of writing a program as multiple files. "Where do I start?!!" is a common refrain. This assignment sheet attempts to walk you through the steps of writing a multi-file program. The steps outlined below should not be thought of as a purely linear process, but rather an iterative one - For example, work a little on Step 1, then a little on Step 2, then test what you've written (Step 3). Step 1: Write the Seller class declaration The Seller class represents information about a salesperson. The code for the Seller class will be placed in two separate files, which is the norm for non-template C++ classes. The header file for a class contains the class declaration, including declarations of any data members and prototypes for the methods of the class. The name of the header file should be of the form ClassName.h (for example, Seller.h for the header file of the Seller class). A skeleton for the Seller.h file is given below. As shown, a header file should begin and end with header guards to prevent it from accidentally being #included more than once in the same source code file (which would produce duplicate symbol definition errors). The symbol name used in the header guards can be any valid C++ name that is not already in use in your program or the C/C++ libraries. Using a name of the format CLASSNAME_H (like SELLER_H in the code below) is recommended to avoid naming conflicts. #ifndef SELLER_H #define SELLER_H //***************************************************************** // FILE: Seller.h // AUTHOR: your name // LOGON ID: your DogTag // DUE DATE: due date of assignment // // PURPOSE: Contains the declaration for the Seller class. //***************************************************************** class Seller { // Data members and method prototypes for the Seller class go here . . . }; #endif Data Members The Seller class should have the following two private data members: A name (a character array with room for 30 characters PLUS the null character) A sales total (a double variable) Note: Make sure you code your data members in THE EXACT ORDER LISTED ABOVE and with THE EXACT SAME DATA TYPES. If you use float instead of double or only make the name array 30 characters long instead of 31, your final program will not work correctly. Method Prototypes The Seller class declaration should (eventually) contain public prototypes for all of the methods in the Seller.cpp source code file described in Step 2 below. Step 2: Write the Seller class implementation The source code file for a class contains the method definitions for the class. The name of the source code file should be of the form ClassName.cpp or ClassName.cc (for example, Seller.cpp for the source code file of the Seller class). The Seller class implementation should (eventually) contain definitions for all of the methods described below. Make sure to #include "Seller.h" at the top of this file. Seller default constructor This "default" constructor for the Seller class takes no parameters. Like all C++ constructors, it does not have a return data type. This method should set the name data member to a "null string". This can be done by copying a null string literal ("") into the character array using strcpy() or by setting the first element of the array to a null character ('\0'). The sales total data member should be set to 0. Alternate Seller constructor Write another constructor for the Seller class that takes two parameters: 1) a character array that contains a new name, and 2) a double variable that contains a new sales total. DO NOT GIVE THESE PARAMETERS THE SAME NAMES AS YOUR DATA MEMBERS.. Like all C++ constructors, this constructor does not have a return data type. Use strcpy() to copy the new name parameter into the name data member. Assign the new sales total parameter to the sales total data member. getName() This accessor method takes no parameters. It should return the name data member. In C++, the usual return data type specified when you are returning the name of a character array is char* or "pointer to a character" (since returning an array's name will convert the name into a pointer to the first element of the array, which in this case is data type char. getSalesTotal() This accessor method takes no parameters. It will have a return data type of double. It should return the sales total data member. setSalesTotal() This accessor method takes one parameter, a a double variable that contains a new sales total. DO NOT GIVE THIS PARAMETER THE SAME NAME AS YOUR DATA MEMBER.. The method returns nothing. It should assign the new sales total parameter to the sales total data member. print() This method takes no parameters and returns nothing. The method should print the name and sales total data members on the console using cout. Use setw() to line the printed values up in columns (a width of 30 for the name and 9 for the sales total will match the sample output). The name should be left-justified; the sales total should be right justified and printed using fixed-point notation with two places after the decimal point. Step 3: Test and debug the Seller class As you write your declaration and implementation of the Seller class, you should begin testing the code you've written. Create a basic main program called assign2.cpp that tests your class. This is not the final version of assign2.cpp that you will eventually submit. In fact, you'll end up deleting most (or all) of it by the time you're done with the assignment. An example test program is given below. You do not have to have written all of the methods for the Seller class before you begin testing it. Simply comment out the parts of your test program that call methods you haven't written yet. Write one method definition, add its prototype to the class declaration, uncomment the corresponding test code in your test program, and then compile and link your program. If you get syntax errors, fix them before you attempt to write additional code. A larger amount of code that does not compile is not useful - it just makes debugging harder! The goal here is to constantly maintain a working program. #include
Step by Step Solution
There are 3 Steps involved in it
Step: 1
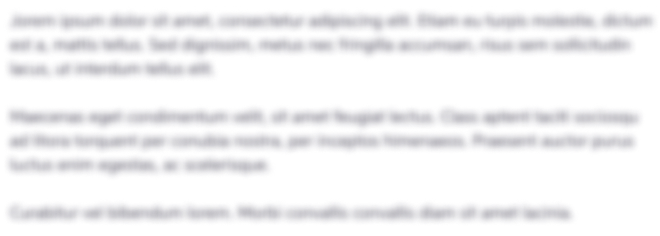
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started