Question
This is in java and please show all the work. it would be helpful, if you could put the steps number as comment. this like
This is in java and please show all the work. it would be helpful, if you could put the steps number as comment. this like my thired time posting this question, please someone help.
Directed Lab Work
Implementing the Event Queue
All but two of the classes needed in todays lab exist. The first class that will be created is the
SimulationEventQueue.
Step 1. In the QueuePackage, create a new class named SimulationEventQueue.
Step 2. In the class declaration, make it implement SimulationEventQueueInterface.
Step 3. Create method stubs for each of the methods in the interface.
Checkpoint: The class should compile now.
Step 4. Create a private variable to store the current simulation time.
Step 5. Create a private variable to store the contents of the queue.
Step 6. Implement all of the methods except for add(). You may find the class VectorQueue helpful.
Step 7. In the remove() method, add code to change the current time of the event queue.
Step 8. Refer to the pre-lab exercises and implement the add() method.
Checkpoint: The class should compile.
The Bank Line Animation
Checkpoint: The bank application should run. At the very start of the set up phase init() will be called. The
customer generator in its constructor puts its initial event on the event queue. That should show up as the next
event. No customers should be in the line. The bank teller Fred should be waiting patiently for customers to
show up. The report should indicate that there are no customers waiting or served. The simulation time is 0.0.
At this point, it would be nice to see the event queue in operation. Code to drive the simulation will be added
into the BankActionThread.
Creating the Event Loop
Step 9. In the method executeApplication() in BankActionThread, add code that will repeatedly
take events from the event queue and process them. Refer to the pre-lab exercises.
The display for the simulation has a few requirements for what happens in the event loop.
Step 10. Inside the loop after the event has been processed, get the post action report from the event and
use it to set lastEventReport.
Step 11. If there is a next event, get the description and use it to set nextEventAction.
Step 12. Update the time for the report.
Step 13. The last code in the loop should be the line that will pause the animation.
animationPause();
Checkpoint: Compile and run the application. Press go. Customers should be generated and placed one at a
time into the line. You should see them. Unfortunately, Fred is busy drinking coffee and is not yet helping the
customers. The simulation should stop once it hits 1000.
Completing the Teller Event
It is time for Fred to get to work. The process method for CheckForCustomerEvent inside the Teller class needs
to be completed.
Step 14. Refer to the pre-lab exercises and complete the code for the method process().
Step 15. If no customer was served, set serving to null.
Step 16. At the end of processing, make sure to set postActionReport with a string describing the
actions taken by the event.
Step 17. In the constructor for Teller, add code that will generate the first CheckForCustomerEvent.
(This is similar to how the customer generator operates.)
Checkpoint: The teller should now take customers from the line. As customers are serviced, the report should
change. Step and carefully trace the operation of the simulation. Verify that it is operating correctly.
Change the service interval time and verify that customers are handled quicker.
Graphing the Results
Step 18. Run the simulation with a maximum interval of 20 and a simulation time limit of 1000. Fill in the
following table.
MAXIMUM SERVICE TIME | AVERAGE WAIT TIME FOR CUSTOMERS SERVED |
6 | |
8 | |
10 | |
12 | |
14 | |
16 | |
18 | |
20 | |
22 | |
24 |
Step 19. Use the table to plot points on the following graph.
Suppose the service time is greater than the interval that customers appear. You expect that the teller will fall
behind and the length of the line will increase without bound. If the service time is less, you expect that the
teller will be able to keep up.
The interesting question is what happens when the service and interval times are the same. How long will the
line be on average in this case? It turns out that the average, as the simulation time increases, will approach
infinity. This will also have an effect on the average waiting time.
Step 20. Run the application 20 times with the initial settings and record the average. (Warning: If you set
the animation delay time to be too small, the animation may not stop at the end of the simulation but restart at
time zero.)
Step 21. What was the maximum average wait?
Step 22. What was the minimum average wait?
/
/
/
/
here are all the class, someone of them are useless.
EmptyQueueException class
/* * An exception used to signal that attempted to get a value from an empty queue. * * @author Charles Hoot * @version 4.0 */
package QueuePackage;
public final class EmptyQueueException extends RuntimeException {
public EmptyQueueException(String s) { super(s); } } /
/
/
/
PriorityQueueInterface class
package QueuePackage;
/** An interface for the priority queue ADT. * * This code is from Chapter 10 of * Data Structures and Abstractions with Java 4/e * by Carrano */ public interface PriorityQueueInterface
/
/
/
/
QueueInterface class
package QueuePackage;
/** An interface for the queue ADT * * This code is from Chapter 10 of * Data Structures and Abstractions with Java 4/e * by Carrano */ public interface QueueInterface
/
/
/
/
SimulationEvent class
package QueuePackage;
/** * An abstract class that can be used as the base for other event classes. * * @author Charles Hoot * @version 4.0 */ public abstract class SimulationEvent implements SimulationEventInterface { private double atTime; private String description; protected String postActionReport; public SimulationEvent (double theTime, String action) { atTime = theTime; description = action; postActionReport = "Event has not fired yet"; } /** * Get the time for the event. * * @return The time of the event. */ public double getTime() { return atTime; } /** * Process the event. */ abstract public void process(); /** * Get a string representing the action for the event. * * @return A description of the event. */ public String getDescription() { return description; } /** * Get a string representing reporting on the status of the event * after it has finished executing. * * @return A post event action report. */ public String getPostActionReport() { return postActionReport; } } // end of Event
/
/
/
/
SimulationEventInterface class
package QueuePackage;
/** * A description of the protocol that an event must respond to. * * @author Charles Hoot * @version 4.0 */
public interface SimulationEventInterface { /** * Get the time for the event. * * @return The time of the event. */ public double getTime(); /** * Process the event. */ public void process(); /** * Get a string representing the action for the event. * * @return A description of the event. */ public String getDescription(); /** * Get a string representing reporting on the status of the event * after it has finished executing. * * @return A post event action report. */ public String getPostActionReport(); } // end SimulationEventInterface
/
/
/
/
SimulationEventQueueInterface class
package QueuePackage;
/** * An interface for a priority queue that will be used to hold events for a simulation. * * @author Charles Hoot * @version 4.0 */
public interface SimulationEventQueueInterface { /** Adds a new event to this event queue. If the time of the event to be added * is earlier the the time for this event queue, do not add the event. * @param newEntry An event. */ public void add(SimulationEvent newEntry); /** Removes and returns the item with the earliest time. * @return The event with the earliest time or, * if the event queue was empty before the operation, null. */ public SimulationEvent remove(); /** Retrieves the item with the earliest time. * @return The event with the earliest time or, * if the event queue was empty was empty before the operation, null. */ public SimulationEvent peek(); /** Detects whether this event queue is empty. * @return True if the event queue is empty. */ public boolean isEmpty(); /** Gets the size of this event queue. * @return The number of entries currently in the event queue. */ public int getSize(); /** Removes all entries from this event queue. */ public void clear(); /** * The current time of the simulation * * @return The time for the first event on the queue. */ public double getCurrentTime(); }
/
/
/
/
VectorQueue class
package QueuePackage;
import java.util.Vector; import java.util.List;
/** A queue implemented using a Vector. Since it only uses methods * from the List interface, we can use other classes like ArrayList * or LinkedList instead of Vector. * * This code is loosely based on the implementations of a Queue from Chapter 11 of * Data Structures and Abstractions with Java 4/e * by Carrano * */
public class VectorQueue
/** Removes and returns the entry at the front of this queue. * @return The object at the front of the queue. * @throws EmptyQueueException if the queue was empty before the operation. * */ public T dequeue() { T front = null; if (isEmpty()) throw new EmptyQueueException("Attempting to access entries on an empty queue."); else front = queue.remove(0); return front; } // end dequeue
/** Retrieves the front of this queue. * @return The object at the front of the queue. * @throws EmptyQueueException if the queue is empty. * */ public T getFront() { T front = null; if (isEmpty()) throw new EmptyQueueException("Attempting to access entries on an empty queue."); else front = queue.get(0); return front; } // end getFront
/** Detects whether the queue is empty. * @return True if the queue is empty, or false otherwise. * */ public boolean isEmpty() { return queue.isEmpty(); } // end isEmpty /** Removes all entries from this queue. * */ public void clear() { queue.clear(); } // end clear }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
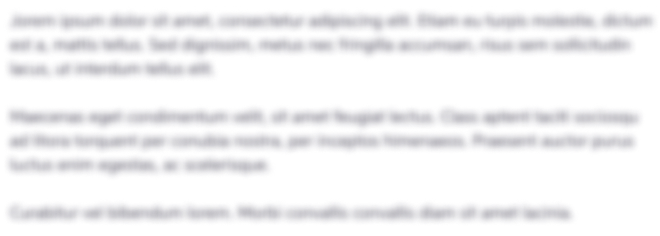
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started