Question
This is in java! Can you please help? the code that i have already written down is at the bottom, everything that is there was
This is in java! Can you please help? the code that i have already written down is at the bottom, everything that is there was provided by the teacher
Your program should take the following inputs:
The size of the magic square
The values to go into the magic square
Your program should perform the following computations:
Calculate the magic number from the magic square size
Check if the inputted list of numbers can even be a valid magic square by giving it to the compareArrays method and examining the boolean return value.
The compareArrays method checks that there is only a single instance of each integer from 1 to (size2 - 1)
Check if the sum of all rows, columns, and diagonals are equal to the magic number
Additional Requirements:
Use only scnr.nextInt() for this lab as we only need to take in ints as inputs.
You must use a 2D array.
Your program will need to print out the various inputs and results in proper formatting, as shown in the sample outputs below.
Sample Outputs
Output 1:
Enter in the magic square size you would like:
3
The magic number for size 3 is 15.
Enter in the values:
4 9 2 3 5 7 8 1 6
You entered:
4 9 2
3 5 7
8 1 6
Analyzing...
This is a magic square!
Output 2:
Enter in the magic square size you would like:
4
The magic number for size 4 is 34.
Enter in the values:
1 15 14 4 12 6 7 9 8 10 11 5 13 3 2 16
You entered:
1 15 14 4
12 6 7 9
8 10 11 5
13 3 2 16
Analyzing...
This is a magic square!
Output 3:
Enter in the magic square size you would like:
7
The magic number for size 7 is 175.
Enter in the values:
30 39 48 1 10 19 28 38 47 7 9 18 27 29 46 6 8 17 26 35 37 5 14 16 25 34 36 45 13 15 24 33 42 44 4 21 23 32 41 43 3 12 22 31 40 49 2 11 20
You entered:
30 39 48 1 10 19 28
38 47 7 9 18 27 29
46 6 8 17 26 35 37
5 14 16 25 34 36 45
13 15 24 33 42 44 4
21 23 32 41 43 3 12
22 31 40 49 2 11 20
Analyzing...
This is a magic square!
Output 4:
Enter in the magic square size you would like:
5
The magic number for size 5 is 65.
Enter in the values:
17 2 23 8 15 24 7 14 16 1 5 21 19 3 17 6 12 18 13 16 13 22 4 20 5
You entered:
17 2 23 8 15
24 7 14 16 1
5 21 19 3 17
6 12 18 13 16
13 22 4 20 5
Analyzing...
The input cannot be a magic square! There must be one of each value from 1 to 25.
Row 1 does not work! These are the values in row 1: 24 7 14 16 1
Row 4 does not work! These are the values in row 4: 13 22 4 20 5
Column 1 does not work! These are the values in column 1: 2 7 21 12 22
Column 2 does not work! These are the values in column 2: 23 14 19 18 4
Column 3 does not work! These are the values in column 3: 8 16 3 13 20
Column 4 does not work! These are the values in column 4: 15 1 17 16 5
Diagonal 1 does not work! These are the values in diagonal 1: 17 7 19 13 5
Diagonal 2 does not work! These are the values in diagonal 2: 15 16 19 12 13
This is not a magic square!
Output 5:
Enter in the magic square size you would like:
4
The magic number for size 4 is 34.
Enter in the values:
16 1 7 10 8 11 6 9 5 14 2 13 12 3 15 4
You entered:
16 1 7 10
8 11 6 9
5 14 2 13
12 3 15 4
Analyzing...
Column 0 does not work! These are the values in column 0: 16 8 5 12
Column 1 does not work! These are the values in column 1: 1 11 14 3
Column 2 does not work! These are the values in column 2: 7 6 2 15
Column 3 does not work! These are the values in column 3: 10 9 13 4
Diagonal 1 does not work! These are the values in diagonal 1: 16 11 2 4
Diagonal 2 does not work! These are the values in diagonal 2: 10 6 14 12
This is not a magic square!
Output 6:
Enter in the magic square size you would like:
4
The magic number for size 4 is 34.
Enter in the values:
1 16 6 11 14 7 9 4 8 5 12 13 3 10 15 2
You entered:
1 16 6 11
14 7 9 4
8 5 12 13
3 10 15 2
Analyzing...
Row 2 does not work! These are the values in row 2: 8 5 12 13
Row 3 does not work! These are the values in row 3: 3 10 15 2
Column 0 does not work! These are the values in column 0: 1 14 8 3
Column 1 does not work! These are the values in column 1: 16 7 5 10
Column 2 does not work! These are the values in column 2: 6 9 12 15
Column 3 does not work! These are the values in column 3: 11 4 13 2
Diagonal 1 does not work! These are the values in diagonal 1: 1 7 12 2
Diagonal 2 does not work! These are the values in diagonal 2: 11 9 5 3
This is not a magic square!
Output 7:
Enter in the magic square size you would like:
3
The magic number for size 3 is 15.
Enter in the values:
1 2 3 4 5 6 7 8 9
You entered:
1 2 3
4 5 6
7 8 9
Analyzing...
Row 0 does not work! These are the values in row 0: 1 2 3
Row 2 does not work! These are the values in row 1: 7 8 9
Column 0 does not work! These are the values in column 0: 1 4 7
Column 2 does not work! These are the values in column 2: 3 6 9
This is not a magic square!
Output 8:
Enter in the magic square size you would like:
4
The magic number for size 4 is 34.
Enter in the values:
34 0 0 0 0 34 0 0 0 0 0 34 0 0 0 0 34
You entered:
34 0 0 0
0 34 0 0
0 0 0 34
0 0 0 0
Analyzing...
The input cannot be a magic square! There must be one of each value from 1 to 16.
Row 3 does not work! These are the values in row 3: 0 0 0 0
Column 2 does not work! These are the values in column 2: 0 0 0 0
Diagonal 1 does not work! These are the values in diagonal 1: 34 34 0 0
Diagonal 2 does not work! These are the values in diagonal 2: 0 0 0 0
This is not a magic square!
Output 9:
Enter in the magic square size you would like:
2
The magic number for size 3 is 15.
Enter in the values:
1 2 2 1
You entered:
1 2
2 1
Analyzing...
The input cannot be a magic square! There must be one of each value from 1 to 4.
Row 0 does not work! These are the values in row 0: 1 2
Row 1 does not work! These are the values in row 1: 2 1
Column 0 does not work! These are the values in column 0: 1 2
Column 1 does not work! These are the values in column 1: 2 1
Diagonal 1 does not work! These are the values in diagonal 1: 1 1
Diagonal 2 does not work! These are the values in diagonal 2: 2 2
This is not a magic square!
Output 10:
Enter in the magic square size you would like:
-1
Please enter in a number in greater than 0
-3
Please enter in a number in greater than 0
5
The magic number for size 5 is 65.
Enter in the values:
9 3 22 16 15 2 21 20 14 8 25 19 13 7 1 18 12 6 5 24 11 10 4 23 17
You entered:
9 3 22 16 15
2 21 20 14 8
25 19 13 7 1
18 12 6 5 24
11 10 4 23 17
Analyzing...
This is a magic square!
import java.util.HashSet;
import java.util.Set;
import java.util.Scanner;
public class MagicSquare {
//equation to find magic sqaure. n(n^2 + 1)/2;
/**
* Description: Checks if the arrays have the same elements using a set comparison
*
* @param arr the 2D integer array to be sorted and compared
* @return true if the array contains all numbers from 1 to size^2, false otherwise
*/
public static boolean compareArrays(int arr[][]) {
Set
for (int i = 1; i <= Math.pow(arr.length, 2.0); i++) {
expectedSet.add(i);
}
// Check if the set of array elements is equal to the expected set
Set
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr.length; j++) {
arrSet.add(arr[i][j]);
}
}
return arrSet.equals(expectedSet);
}
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
System.out.println("Enter in the magic square size you would like:");
int magicSquare = scnr.nextDouble();
int magicNumber = magicSquare * (Math.pow(magicSquare,2) + 1) / 2;
System.out.println("The magic number for size " + magicSquare + " is " + magicNumber);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
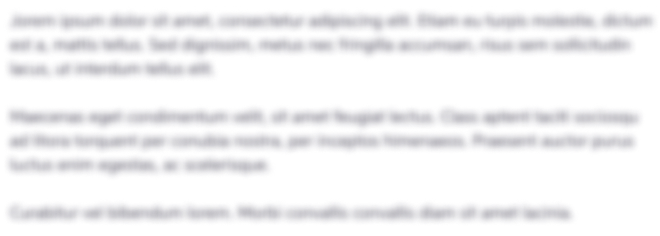
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started