Question
This is in the language of Java all of the files I have right now are what I have right now at the bottom of
This is in the language of Java all of the files I have right now are what I have right now at the bottom of this question. But I need help with getting the JUnit tests involved as well as the JSP as shown in the instructions below.
Attachment.java, Ticket.java,and TicketServlet.javahave been provided for you. You will need to add the Junit tests for the Attachment and Ticket classes. You will also have to put all of these files into the project that you created and get it to a working state. Once you have the above files into the project with the tests and in a working state, then you can move on to the next section.JSP Files In this section you will add the java server pages(jsp) to display the ticket information. To do this you will need to modify the TicketServlet file to call thefollowing .jsp pages instead of using the methods in the class to display the html informationin the switch in doGet and doPost.These are the JSP files that you will need to add.viewTicket will be a jsp page that displays anindividual ticket.You will use the html from the TicketServlet method viewTicket to make it look the same.listTickets will be a jsp page that displays all the tickets. You will use the html from the TicketServlet method listTickets to make it look the same.ticketForm will be a jsp page that gives a form to create a new ticket. You will use the html from the TicketServlet method showTicketForm to make it look the same.
This is the ticket.java file
package com.wrox; import java.util.Collection; import java.util.LinkedHashMap; import java.util.Map; public class Ticket { private String customerName; private String subject; private String body; private Mapattachments = new LinkedHashMap<>(); public String getCustomerName() { return customerName; } public void setCustomerName(String customerName) { this.customerName = customerName; } public String getSubject() { return subject; } public void setSubject(String subject) { this.subject = subject; } public String getBody() { return body; } public void setBody(String body) { this.body = body; } public Attachment getAttachment(String name) { return this.attachments.get(name); } public Collection getAttachments() { return this.attachments.values(); } public void addAttachment(Attachment attachment) { this.attachments.put(attachment.getName(), attachment); } public int getNumberOfAttachments() { return this.attachments.size(); } }
This is attachment.java:
package com.wrox; public class Attachment { private String name; private byte[] contents; public String getName() { return name; } public void setName(String name) { this.name = name; } public byte[] getContents() { return contents; } public void setContents(byte[] contents) { this.contents = contents; } }
This is servelet.java:
package com.wrox; import javax.servlet.ServletException; import javax.servlet.ServletOutputStream; import javax.servlet.annotation.MultipartConfig; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.http.Part; import java.io.ByteArrayOutputStream; import java.io.IOException; import java.io.InputStream; import java.io.PrintWriter; import java.util.LinkedHashMap; import java.util.Map; @WebServlet( name = "ticketServlet", urlPatterns = {"/tickets"}, loadOnStartup = 1 ) @MultipartConfig( fileSizeThreshold = 5_242_880, //5MB maxFileSize = 20_971_520L, //20MB maxRequestSize = 41_943_040L //40MB ) public class TicketServlet extends HttpServlet { private volatile int TICKET_ID_SEQUENCE = 1; private MapticketDatabase = new LinkedHashMap<>(); @Override protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { String action = request.getParameter("action"); if(action == null) action = "list"; switch(action) { case "create": this.showTicketForm(response); break; case "view": this.viewTicket(request, response); break; case "download": this.downloadAttachment(request, response); break; case "list": default: this.listTickets(response); break; } } @Override protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { String action = request.getParameter("action"); if(action == null) action = "list"; switch(action) { case "create": this.createTicket(request, response); break; case "list": default: response.sendRedirect("tickets"); break; } } private void showTicketForm(HttpServletResponse response) throws ServletException, IOException { PrintWriter writer = this.writeHeader(response); writer.append(" Create a Ticket
"); writer.append(" "); this.writeFooter(writer); } private void viewTicket(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { String idString = request.getParameter("ticketId"); Ticket ticket = this.getTicket(idString, response); if(ticket == null) return; PrintWriter writer = this.writeHeader(response); writer.append("Ticket #").append(idString) .append(": ").append(ticket.getSubject()).append("
"); writer.append("Customer Name - ").append(ticket.getCustomerName()) .append("
"); writer.append(ticket.getBody()).append("
"); if(ticket.getNumberOfAttachments() > 0) { writer.append("Attachments: "); int i = 0; for(Attachment attachment : ticket.getAttachments()) { if(i++ > 0) writer.append(", "); writer.append("") .append(attachment.getName()).append(""); } writer.append("
"); } writer.append("Return to list tickets "); this.writeFooter(writer); } private void downloadAttachment(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { String idString = request.getParameter("ticketId"); Ticket ticket = this.getTicket(idString, response); if(ticket == null) return; String name = request.getParameter("attachment"); if(name == null) { response.sendRedirect("tickets?action=view&ticketId=" + idString); return; } Attachment attachment = ticket.getAttachment(name); if(attachment == null) { response.sendRedirect("tickets?action=view&ticketId=" + idString); return; } response.setHeader("Content-Disposition", "attachment; filename=" + attachment.getName()); response.setContentType("application/octet-stream"); ServletOutputStream stream = response.getOutputStream(); stream.write(attachment.getContents()); } private void listTickets(HttpServletResponse response) throws ServletException, IOException { PrintWriter writer = this.writeHeader(response); writer.append("Tickets
"); writer.append("Create Ticket") .append("
"); if(this.ticketDatabase.size() == 0) writer.append("There are no tickets in the system. "); else { for(int id : this.ticketDatabase.keySet()) { String idString = Integer.toString(id); Ticket ticket = this.ticketDatabase.get(id); writer.append("Ticket #").append(idString) .append(": ").append(ticket.getSubject()) .append(" (customer: ").append(ticket.getCustomerName()) .append(")
"); } } this.writeFooter(writer); } private void createTicket(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { Ticket ticket = new Ticket(); ticket.setCustomerName(request.getParameter("customerName")); ticket.setSubject(request.getParameter("subject")); ticket.setBody(request.getParameter("body")); Part filePart = request.getPart("file1"); if(filePart != null && filePart.getSize() > 0) { Attachment attachment = this.processAttachment(filePart); if(attachment != null) ticket.addAttachment(attachment); } int id; synchronized(this) { id = this.TICKET_ID_SEQUENCE++; this.ticketDatabase.put(id, ticket); } response.sendRedirect("tickets?action=view&ticketId=" + id); } private Attachment processAttachment(Part filePart) throws IOException { InputStream inputStream = filePart.getInputStream(); ByteArrayOutputStream outputStream = new ByteArrayOutputStream(); int read; final byte[] bytes = new byte[1024]; while((read = inputStream.read(bytes)) != -1) { outputStream.write(bytes, 0, read); } Attachment attachment = new Attachment(); attachment.setName(filePart.getSubmittedFileName()); attachment.setContents(outputStream.toByteArray()); return attachment; } private Ticket getTicket(String idString, HttpServletResponse response) throws ServletException, IOException { if(idString == null || idString.length() == 0) { response.sendRedirect("tickets"); return null; } try { Ticket ticket = this.ticketDatabase.get(Integer.parseInt(idString)); if(ticket == null) { response.sendRedirect("tickets"); return null; } return ticket; } catch(Exception e) { response.sendRedirect("tickets"); return null; } } private PrintWriter writeHeader(HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html"); response.setCharacterEncoding("UTF-8"); PrintWriter writer = response.getWriter(); writer.append(" ") .append(" ") .append(" ") .append("Customer Support ") .append(" ") .append(" "); return writer; } private void writeFooter(PrintWriter writer) { writer.append(" ").append(" "); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
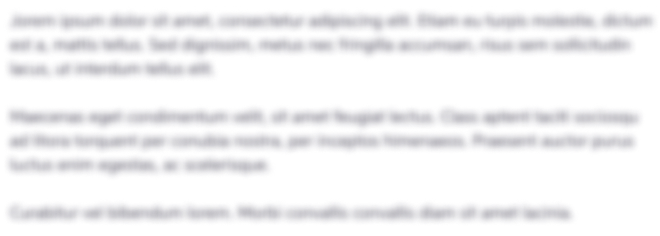
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started