THIS MUST BE IN C# AND WORK AS INTENDED, PLEASE INCLUDE A SCREENSHOT OF THE DESIRED OUTPUT THAT IS REQUESTED TO SHOW THAT THE PROGRAM WORKS.
I NEED THIS TO BE PROGRAMMED IN VISUAL STUDIO 2019, THANKS IN ADVANCE
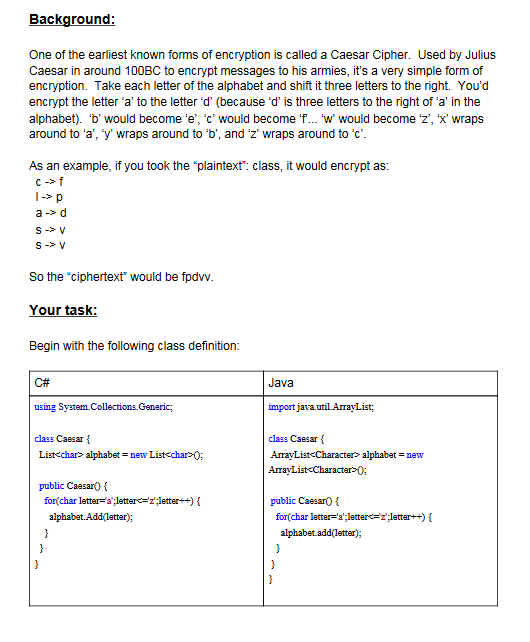
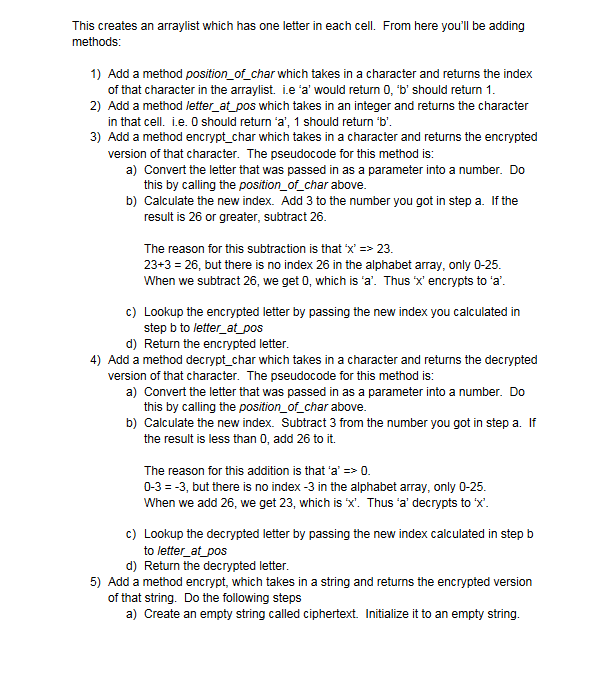
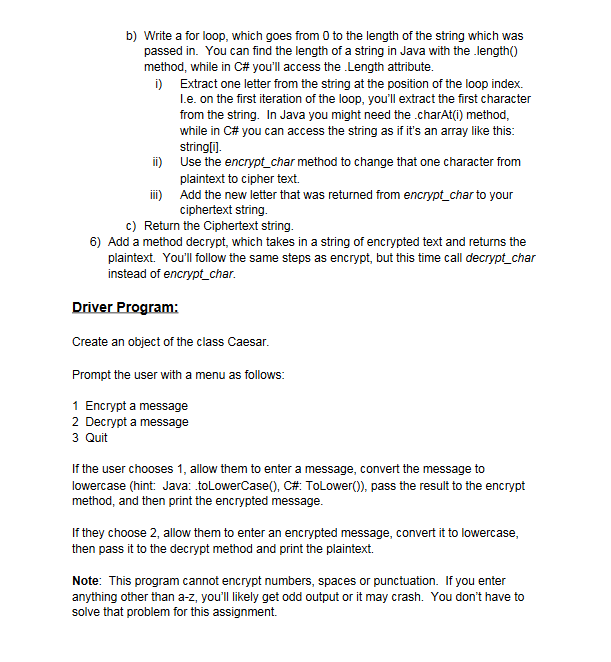
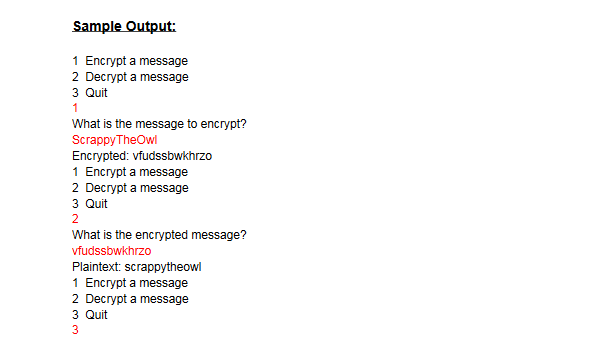
Background: One of the earliest known forms of encryption is called a Caesar Cipher. Used by Julius Caesar in around 100BC to encrypt messages to his armies, it's a very simple form of encryption. Take each letter of the alphabet and shift it three letters to the right. You'd encrypt the letter 'a' to the letter 'd' (because 'd' is three letters to the right of 'a' in the alphabet). 'D' would become 'e', 'c' would become 'f... 'W' would become 'z', 'x' wraps around to 'a', 'y' wraps around to 'b', and z' wraps around to 'c'. As an example, if you took the plaintext": class, it would encrypt as: 1 ->p a>d S-V S->V So the "ciphertext would be fpdvv. Your task: Begin with the following class definition: C# Java using System.Collections.Generic; import java.util.ArrayList; class Caesar List
alphabet = new List0; class Caesar ArrayListO: public Caesaro { for(char letter='a';letter=2;letter++) { alphabet.Add(letter); } 3 } public Caesar() { for(char letter="'a';letter 23. 23+3 = 26, but there is no index 26 in the alphabet array, only 0-25. When we subtract 26, we get 0, which is 'a'. Thus x' encrypts to 'a'. c) Lookup the encrypted letter by passing the new index you calculated in step b to letter_at_pos d) Return the encrypted letter. 4) Add a method decrypt_char which takes in a character and returns the decrypted version of that character. The pseudocode for this method is: a) Convert the letter that was passed in as a parameter into a number. Do this by calling the position_of_char above. b) Calculate the new index. Subtract 3 from the number you got in step a. If the result is less than 0, add 26 to it. The reason for this addition is that 'a' => 0. 0-3 = -3, but there is no index -3 in the alphabet array, only 0-25. When we add 26, we get 23, which is 'x'. Thus 'a' decrypts to "x". c) Lookup the decrypted letter by passing the new index calculated in step b to letter_at_pos d) Return the decrypted letter. 5) Add a method encrypt, which takes in a string and returns the encrypted version of that string. Do the following steps a) Create an empty string called ciphertext. Initialize it to an empty string. b) Write a for loop, which goes from 0 to the length of the string which was passed in. You can find the length of a string in Java with the length() method, while in C# you'll access the.Length attribute. i) Extract one letter from the string at the position of the loop index. L.e. on the first iteration of the loop, you'll extract the first character from the string. In Java you might need the .charAt(i) method, while in C# you can access the string as if it's an array like this: string[]. ii) Use the encrypt_char method to change that one character from plaintext to cipher text. iii) Add the new letter that was returned from encrypt_char to your ciphertext string. c) Return the Ciphertext string. 6) Add a method decrypt, which takes in a string of encrypted text and returns the plaintext. You'll follow the same steps as encrypt, but this time call decrypt_char instead of encrypt_char. Driver Program: Create an object of the class Caesar. Prompt the user with a menu as follows: 1 Encrypt a message 2 Decrypt a message 3 Quit If the user chooses 1, allow them to enter a message, convert the message to lowercase (hint: Java: to LowerCase(), C#: ToLower), pass the result to the encrypt method, and then print the encrypted message. If they choose 2, allow them to enter an encrypted message, convert it to lowercase, then pass it to the decrypt method and print the plaintext. Note: This program cannot encrypt numbers, spaces or punctuation. If you enter anything other than a-z, you'll likely get odd output or it may crash. You don't have to solve that problem for this assignment. Sample Output: 1 Encrypt a message 2 Decrypt a message 3 Quit 1 2 What is the message to encrypt? ScrappyTheOwl Encrypted: vfudssbwkhrzo 1 Encrypt a message 2 Decrypt a message 3 Quit What is the encrypted message? vfudssbwkhrzo Plaintext: scrappytheowl 1 Encrypt a message 2 Decrypt a message 3 Quit 3 Background: One of the earliest known forms of encryption is called a Caesar Cipher. Used by Julius Caesar in around 100BC to encrypt messages to his armies, it's a very simple form of encryption. Take each letter of the alphabet and shift it three letters to the right. You'd encrypt the letter 'a' to the letter 'd' (because 'd' is three letters to the right of 'a' in the alphabet). 'D' would become 'e', 'c' would become 'f... 'W' would become 'z', 'x' wraps around to 'a', 'y' wraps around to 'b', and z' wraps around to 'c'. As an example, if you took the plaintext": class, it would encrypt as: 1 ->p a>d S-V S->V So the "ciphertext would be fpdvv. Your task: Begin with the following class definition: C# Java using System.Collections.Generic; import java.util.ArrayList; class Caesar List alphabet = new List0; class Caesar ArrayListO: public Caesaro { for(char letter='a';letter=2;letter++) { alphabet.Add(letter); } 3 } public Caesar() { for(char letter="'a';letter 23. 23+3 = 26, but there is no index 26 in the alphabet array, only 0-25. When we subtract 26, we get 0, which is 'a'. Thus x' encrypts to 'a'. c) Lookup the encrypted letter by passing the new index you calculated in step b to letter_at_pos d) Return the encrypted letter. 4) Add a method decrypt_char which takes in a character and returns the decrypted version of that character. The pseudocode for this method is: a) Convert the letter that was passed in as a parameter into a number. Do this by calling the position_of_char above. b) Calculate the new index. Subtract 3 from the number you got in step a. If the result is less than 0, add 26 to it. The reason for this addition is that 'a' => 0. 0-3 = -3, but there is no index -3 in the alphabet array, only 0-25. When we add 26, we get 23, which is 'x'. Thus 'a' decrypts to "x". c) Lookup the decrypted letter by passing the new index calculated in step b to letter_at_pos d) Return the decrypted letter. 5) Add a method encrypt, which takes in a string and returns the encrypted version of that string. Do the following steps a) Create an empty string called ciphertext. Initialize it to an empty string. b) Write a for loop, which goes from 0 to the length of the string which was passed in. You can find the length of a string in Java with the length() method, while in C# you'll access the.Length attribute. i) Extract one letter from the string at the position of the loop index. L.e. on the first iteration of the loop, you'll extract the first character from the string. In Java you might need the .charAt(i) method, while in C# you can access the string as if it's an array like this: string[]. ii) Use the encrypt_char method to change that one character from plaintext to cipher text. iii) Add the new letter that was returned from encrypt_char to your ciphertext string. c) Return the Ciphertext string. 6) Add a method decrypt, which takes in a string of encrypted text and returns the plaintext. You'll follow the same steps as encrypt, but this time call decrypt_char instead of encrypt_char. Driver Program: Create an object of the class Caesar. Prompt the user with a menu as follows: 1 Encrypt a message 2 Decrypt a message 3 Quit If the user chooses 1, allow them to enter a message, convert the message to lowercase (hint: Java: to LowerCase(), C#: ToLower), pass the result to the encrypt method, and then print the encrypted message. If they choose 2, allow them to enter an encrypted message, convert it to lowercase, then pass it to the decrypt method and print the plaintext. Note: This program cannot encrypt numbers, spaces or punctuation. If you enter anything other than a-z, you'll likely get odd output or it may crash. You don't have to solve that problem for this assignment. Sample Output: 1 Encrypt a message 2 Decrypt a message 3 Quit 1 2 What is the message to encrypt? ScrappyTheOwl Encrypted: vfudssbwkhrzo 1 Encrypt a message 2 Decrypt a message 3 Quit What is the encrypted message? vfudssbwkhrzo Plaintext: scrappytheowl 1 Encrypt a message 2 Decrypt a message 3 Quit 3