Question
This program involves inputting multiple data items (temperatures) that are each within a certain range and then outputting a report about them utilizing lists and
This program involves inputting multiple data items (temperatures) that are each within a certain range and then outputting a report about them utilizing lists and functions. Write a Python program that will do the following:
1. The program will input from 1 to 35 (inclusive) Fahrenheit temperatures from the user. You must first ask them to enter the number of temperatures that they will be typing in. If the value entered is not between 1 and 35, you need to display a message informing the user that the value they entered is out of range and make them re-input until they provide an acceptable value. Prompt every input.
2. The Fahrenheit values are floats and must range between -150.0 and 350.0 (inclusive.) If an out-of-range temperature is entered, you must display a message informing the user as such and have them re-input until an in-range value is entered.
3. Generate the output specified below.
Notes/Specifications:
1. You must create a list to hold your (float) temperature values.
2. All output needs to be right-justified and aligned by the decimal point.
3. Blank lines are only used to separate sections of the report (#5 below)
4. Display all floating-point (float) numbers to one decimal place.
5. The output must be done in this order:
a. The assignment and your name
b. The values in ascending order (in Fahrenheit and Celsius)
c. The average temperatures (for Fahrenheit and Celsius)
d. The highest and lowest temperatures (for Fahrenheit and Celsius)
e. The amount of temperatures above/equal to/and below the average (once)
f. The standard deviation for Fahrenheit. Use corrected sample standard deviation (n-1 divisor) http://en.wikipedia.org/wiki/Standard_deviation (be sure to address and avoid a potential divide by zero.)
6. Part of your grade is based on how well you break down the problem into smaller components. Each function should only handle one primary task (computing and outputting the same part of a report can be in the same function for this assignment think about whether or not thats best on a case-by-case basis.)
NOTE: Taking all code and putting it into a function thats called from __main__ or other attempts to circumvent this requirement will result in an extremely large deduction. Humongous, in fact.
7. The sort algorithm must be Selection Sort or better and not use any library functions.
8. Your code must be free of syntax and runtime errors.
9. Any variables being accessed in a function must be passed in as arguments and used as parameters. No direct global access (e.g. using global) is permitted. 11.The efficiency of each task is also being graded. Code optimally.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
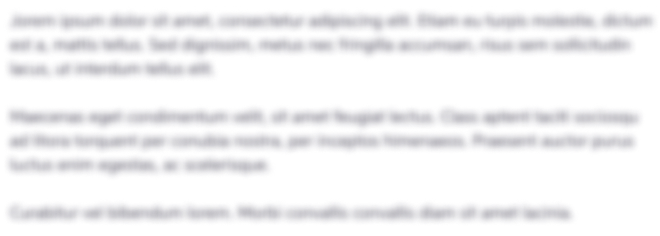
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started