Question
Tic Tac Toe Write a program that allows two players to play a game of tic-tac-toe. Use a two-dimensional char array with three rows and
Tic Tac Toe
Write a program that allows two players to play a game of tic-tac-toe. Use a two-dimensional char array with three rows and three columns as the game board. Each element of the array should be initialized with an asterisk (*). The program should run a loop that:
-
Displays the contents of the board array
-
Allows player 1 to select a location on the board for an X. The program should ask the user to enter the row number and then the column number.
-
Allows player 2 to select a location on the board for an O. The program should ask the user to enter the row number and then the column number.
-
Validates the user's input to ensure that the row and column entered is within the range [13] and that cell is not already taken
-
Determines whether a player has won, or a tie has occurred. If a player has won, the program should declare that player the winner and end. If a tie has occurred, the program should declare that and end.
-
Player 1 wins when there are three Xs in a row on the game board. The Xs can appear in a row, in a column, or diagonally across the board. The same logic applies to player 2, but with Os. A tie occurs when all of the locations on the board are full, but there is no winner.
-
Declare global constant ints ROWS and COLS, each equal to 3.
-
In main, create a 2D array that is 3x3 to hold the game state.
-
Your program must use the following functions. They will be unit tested:
void fillBoard(char [][COLS]);
- Fill the given array with all stars.
- Do not print anything here.
void showBoard(char [][COLS]);
- Output the game board, with labeled rows and columns.
- Print a row with just the column numbers
- Create a nested for loop.
- For each row, print the row number, then run an inner loop to print the the three array values for that row
void getChoice(char [][COLS], bool);
- Input the player's choice and mark it on the game board.
- The first parameter is the game board.
- The second parameter is a bool for which player is currently selected
- false indicates player 1
- true indicates player 2
- this will be toggled when you switch players
- Input the player's choice
- Do not let the user input a row outside the range [13]
- Do not let the user input a column outside the range [13]
- Do not let the user input into a cell that already has an 'X' or 'O'
- If the user enters an invalid input, just ask them for that input again.
- If a row input is invalid, immediately ask for a new row input
- If a column input is invalid, immediately ask for a new column input
- Once a valid row and column are entered, make sure that cell is not taken. If it is taken, ask for new row and column inputs.
- This is best done using nested do-while loops. See the starter code for a useful structure for this.
bool gameOver(char [][COLS]);
-
Determine whether a game ending condition has occurred.
-
If so, print one of the following outputs:
- Player 1 wins!
- Player 2 wins!
- Tie!
-
Return true if a game ending condition has occurred, false if otherwise.
-
To use this design effectively, the code for main is given to you in the starter code:
#includeusing namespace std; const int ROWS = 3; const int COLS = 3; //declare function prototypes int main() { char board[ROWS][COLS]; bool playerToggle = false; fillBoard(board); showBoard(board); while (!gameOver(board)) { getChoice(board, playerToggle); showBoard(board); playerToggle = !playerToggle; } return 0; }
Below is a sample output where player 1 wins:
1 2 3 1 * * * 2 * * * 3 * * * Player 1, Row: 1 Player 1, Column: 1 1 2 3 1 X * * 2 * * * 3 * * * Player 2, Row: 1 Player 2, Column: 2 1 2 3 1 X O * 2 * * * 3 * * * Player 1, Row: 2 Player 1, Column: 2 1 2 3 1 X O * 2 * X * 3 * * * Player 2, Row: 3 Player 2, Column: 1 1 2 3 1 X O * 2 * X * 3 O * * Player 1, Row: 3 Player 1, Column: 3 1 2 3 1 X O * 2 * X * 3 O * X Player 1 wins!
Next is a sample output where player 2 wins after ignoring some invalid input:
1 2 3 1 * * * 2 * * * 3 * * * Player 1, Row: 1 Player 1, Column: 1 1 2 3 1 X * * 2 * * * 3 * * * Player 2, Row: 3 Player 2, Column: 1 1 2 3 1 X * * 2 * * * 3 O * * Player 1, Row: 1 Player 1, Column: 2 1 2 3 1 X X * 2 * * * 3 O * * Player 2, Row: 1 Player 2, Column: 3 1 2 3 1 X X O 2 * * * 3 O * * Player 1, Row: 1 Player 1, Column: 3 Player 1, Row: 9 Player 1, Row: 9 Player 1, Row: 2 Player 1, Column: 1 1 2 3 1 X X O 2 X * * 3 O * * Player 2, Row: 2 Player 2, Column: 2 1 2 3 1 X X O 2 X O * 3 O * * Player 2 wins!
The next example produces a tie:
1 2 3 1 * * * 2 * * * 3 * * * Player 1, Row: 1 Player 1, Column: 1 1 2 3 1 X * * 2 * * * 3 * * * Player 2, Row: 2 Player 2, Column: 1 1 2 3 1 X * * 2 O * * 3 * * * Player 1, Row: 1 Player 1, Column: 2 1 2 3 1 X X * 2 O * * 3 * * * Player 2, Row: 2 Player 2, Column: 2 1 2 3 1 X X * 2 O O * 3 * * * Player 1, Row: 2 Player 1, Column: 3 1 2 3 1 X X * 2 O O X 3 * * * Player 2, Row: 1 Player 2, Column: 3 1 2 3 1 X X O 2 O O X 3 * * * Player 1, Row: 3 Player 1, Column: 1 1 2 3 1 X X O 2 O O X 3 X * * Player 2, Row: 3 Player 2, Column: 2 1 2 3 1 X X O 2 O O X 3 X O * Player 1, Row: 3 Player 1, Column: 3 1 2 3 1 X X O 2 O O X 3 X O X Tie!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
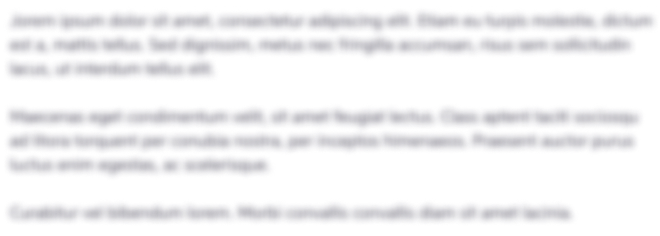
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started