Question
To create linked list structure(Step1), you may use the following code, and complete the rest of the program. #include #include #include #define NULL 0 struct
To create linked list structure(Step1), you may use the following code, and complete the rest of the program.
#include
#include
#include
#define NULL 0
struct employeeInfo
{
int empNr;
char name[15];
float workHours;
float hourlySalary;
float monthlySalary;/* will be calculated by workHours x hourlySalary */
};
struct node
{
struct employeeInfo info;
struct node *link;
};
typedef struct node *NODEPTR;
NODEPTR getnode(void);
void readFileIntoLinkList(NODEPTR *);
void list1(NODEPTR);
void menu();
int main()
{
NODEPTR head1, headNum, headNam;
int choice;
/* Step1 will be done using these two functions
head1 = NULL;
readFileIntoLinkList(&head1);
list1(head1);
do{
menu();
scanf("%d",&choice);
switch(choice)
{ // complete each section calling necessary function that you coded
case 1:{. . . . . . . . . . . . . . . . . . . . . . . . . break;}
case 2:{. . . . . . . . . . . . . . . . . . . . . . . . . .break;}
case 3:{. . . . . . . . . . . . . . . . . . . . . . . . . break;}
case 4:{. . . . . . . . . . . . . . . . . . . . . . . . . .break;}
case 5:{. . . . . . . . . . . . . . . . . . . . . . . . . break;}
case 6:{. . . . . . . . . . . . . . . . . . . . . . . . . ;}
}
}while(choice!=7);
return 0;
} // end of main program
void readFileIntoLinkList(NODEPTR *head)
{
NODEPTR p, save ;
struct employeeInfo temp;
FILE *empFile;
empFile = fopen("C:\\assgn2Solution\\employee.txt" , "r");
while ( !feof(empFile))
{
fscanf(empFile ,"%d %s %f %f" ,&temp.empNr,temp.name,&temp.workHours,&temp.hourlySalary);
temp.monthlySalary = temp.hourlySalary*temp.workHours;
p = getnode();
p->info = temp;
if (*head == NULL)
{
*head = p;
save = p;
}
else{
save->link = p;
save = p;
}
}
p->link=NULL;
fclose(empFile);
}
void menu()
{
printf(" *********MAIN MENU********** ");
printf("1.Create Sorted Link list using Names and List ");
printf("2.Create Sorted Link list using Employee Number and List ");
printf("3.Search with an employee Number ");
printf("4.Search with an Employee Name ");
printf("5.Insert a New Employee information ");
printf("6.Delete an Employee from both of the link list structure ");
printf("7.Exit ");
}
void list1(NODEPTR head)
{
NODEPTR save;
save = head;
while(save!=NULL)
{
printf("%d %s %f %f %f ",save->info.empNr,save->info.name,save->info.hourlySalary,save->info.workHours,save->info.monthlySalary);
save = save->link;
}
}
NODEPTR getnode()
{
NODEPTR q;
q = (NODEPTR) malloc(sizeof(struct node));
return(q);
}
USER CATALOGUE WILL BE PREPARED WITH THE FOLLOWING HEADLINES:
Definition of the problem: Given problem will be explained.
User documentation: How can a single user run your program? Describe in details.
Input environment: What does your program get as an input of information? Explain the content of your input files.
Output environment: What does your program produce? Production of your program will be explained.
Software design issues: Describe the main program and functions (if any) your program.
Program list: List of the program and Input/Output files.
Programming time: How long did you spend to prepare this program for the following steps.
Analyze and design :
Coding :
Debugging :
Documentation :
Total :
Step by Step Solution
There are 3 Steps involved in it
Step: 1
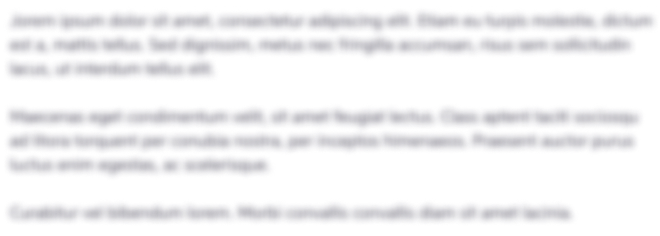
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started