Question
To implement QueueADT interface that defines a queue. Then you are going to use a queue in an application to store objects. Write a LinkedQueue
To implement QueueADT interface that defines a queue. Then you are going to use a queue in an application to store objects. Write a LinkedQueue class that implements the QueueADT.java interface using links. Do not use an instance variable count in your implementation of the queue You may use a local integer variable to count number of nodes in method size . Use the LinearNode class to implement the queue with links . Methods dequeue and first in the LinkedQueue class must throw an EmptyQueueException when queue is empty. Write the EmptyQueueException class. Write an application to store Strings in the queue. Test the following methods from the LinkedQueue class: isEmpty() first() dequeue() enqueue(string) size() toString() deal with the exception thrown by dequeue and first methods Write a method merge in the application. Method merge receives two queues and creates a new queue with objects from both queues. The merging process is similar to the process of merging cars from two lanes into one lane (an object from first lane merges, then a car from the second line merges. Use Try and catch where it is required. Test method merge . Document your code with Javadoc comments
Provided Files: QueueADT and LinearNode
package collections;
/** * A single node for a singly-linked list */ public class LinearNode
/** element stored at this node */ private T element = null; /** Link to the next node in list */ private LinearNode
/** * Creates an empty node */ public LinearNode() { next = null; element = null; }
/** * Creates a node storing the specified element * @param element element to be stored */ public LinearNode(T element) { this.element = element; next = null; }
/** * Creates a node storing the specified element and the specified node * @param element Item to be stored * @param next Reference to the next node in the list */ public LinearNode(T element, LinearNode
/** * Returns the element stored at this node * @return T element stored element at this node */ public T getElement() { return element; }
/** * Set the reference to the stored element * @param element The item to be stored at this node */ public void setElement(T elem) { element = elem; }
/** * Returns the next node * @return SinglyLinkedNode
/** * Sets the reference to the next node in the list * @param next The next node */ public void setNext(LinearNode
package collections; import exceptions.*;
public interface QueueADT
/** * Removes and returns the element at the front of this queue. * @return the element at the front of this queue * @throws EmptyQueueException if an empty collection exception occurs */ public T dequeue()throws EmptyQueueException ;
/** * Returns without removing the element at the front of this queue. * @return the first element in this queue * @throws EmptyQueueException if an empty collection exception occurs */ public T first()throws EmptyQueueException ; /** * Returns true if this queue contains no elements. * @return true if this queue is empty, false otherwise */ public boolean isEmpty();
/** * Returns the number of elements in this queue. * @return the integer representation of the size of this queue */ public int size();
/** * Returns a string representation of this queue. * @return the string representation of this queue */ public String toString(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
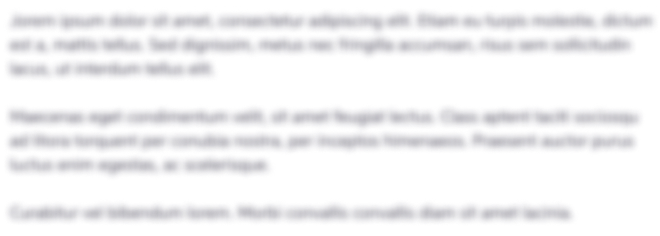
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started