Answered step by step
Verified Expert Solution
Question
1 Approved Answer
To interact with any level you will send raw bytes over stdin to this program. To efficiently solve these problems, first run it to see
To interact with any level you will send raw bytes over stdin to this program. To efficiently solve these problems, first run it to see the challenge instructions. Then craft, assemble, and pipe your bytes to this program. For instance, if you write your assembly code in the file asm.S you can assemble that to an object file: as o asm.o asm.S Note that if you want to use Intel syntax for xwhich of course, you do you'll need to add the following to the start of asm.S: intelsyntax noprefix Then, you can copy the text section your code to the file asm.bin: objcopy O binary onlysectiontext asm.o asm.bin And finally, send that to the challenge: cat asmbin challengerun You can even run this as one command: as o asm.o asm.S && objcopy O binary onlysectiontext asmo asmbin && cat asmbin challengerun In this level you will be working with control flow manipulation. This involves using instructions to both indirectly and directly control the special register rip the instruction pointer. You will use instructions such as: jmp call, cmp and their alternatives to implement the requested behavior. We will be testing your code multiple times in this level with dynamic values! This means we will be running your code in a variety of random ways to verify that the logic is robust enough to survive normal use. The last jump type is the indirect jump, which is often used for switch statements in the real world. Switch statements are a special case of ifstatements that use only numbers to determine where the control flow will go Here is an example: switchnumber: : jmp dothing : jmp dothing : jmp dothing default: jmp dodefaultthing The switch in this example is working on number which can either be or In the case that number is not one of those numbers, the default triggers. You can consider this a reduced elseif type structure. In x you are already used to using numbers, so it should be no suprise that you can make if statements based on something being an exact number. In addition, if you know the range of the numbers, a switch statement works very well. Take for instance the existence of a jump table. A jump table is a contiguous section of memory that holds addresses of places to jump. In the above example, the jump table could look like: x address of dothing xx address of dothing xx address of dothing xx address of dodefaultthing Using the jump table, we can greatly reduce the amount of cmps we use. Now all we need to check is if number is greater than If it is always do: jmp xx Otherwise: jmp jumptableaddress number Using the above knowledge, implement the following logic: if rdi is : jmp x else if rdi is : jmp xf else if rdi is : jmp xd else if rdi is : jmp xe else: jmp xa Please do the above with the following constraints: Assume rdi will NOT be negative Use no more than cmp instruction Use no more than jumps of any variant We will provide you with the number to 'switch' on in rdi. We will provide you with a jump table base address in rsi. Here is an example table: xbxaddrs will change xxf xbxd xxe xbxa Please give me your assembly in bytes up to x bytes:
To interact with any level you will send raw bytes over stdin to this program.
To efficiently solve these problems, first run it to see the challenge instructions.
Then craft, assemble, and pipe your bytes to this program.
For instance, if you write your assembly code in the file asm.S you can assemble that to an object file:
as o asm.o asm.S
Note that if you want to use Intel syntax for xwhich of course, you do you'll need to add the following to the start of asm.S:
intelsyntax noprefix
Then, you can copy the text section your code to the file asm.bin:
objcopy O binary onlysectiontext asm.o asm.bin
And finally, send that to the challenge:
cat asmbin challengerun
You can even run this as one command:
as o asm.o asm.S && objcopy O binary onlysectiontext asmo asmbin && cat asmbin challengerun
In this level you will be working with control flow manipulation. This involves using instructions
to both indirectly and directly control the special register rip the instruction pointer.
You will use instructions such as: jmp call, cmp and their alternatives to implement the requested behavior.
We will be testing your code multiple times in this level with dynamic values! This means we will
be running your code in a variety of random ways to verify that the logic is robust enough to
survive normal use.
The last jump type is the indirect jump, which is often used for switch statements in the real world.
Switch statements are a special case of ifstatements that use only numbers to determine where the control flow will go
Here is an example:
switchnumber:
: jmp dothing
: jmp dothing
: jmp dothing
default: jmp dodefaultthing
The switch in this example is working on number which can either be or
In the case that number is not one of those numbers, the default triggers.
You can consider this a reduced elseif type structure.
In x you are already used to using numbers, so it should be no suprise that you can make if statements based on something being an exact number.
In addition, if you know the range of the numbers, a switch statement works very well.
Take for instance the existence of a jump table.
A jump table is a contiguous section of memory that holds addresses of places to jump.
In the above example, the jump table could look like:
x address of dothing
xx address of dothing
xx address of dothing
xx address of dodefaultthing
Using the jump table, we can greatly reduce the amount of cmps we use.
Now all we need to check is if number is greater than
If it is always do:
jmp xx
Otherwise:
jmp jumptableaddress number
Using the above knowledge, implement the following logic:
if rdi is :
jmp x
else if rdi is :
jmp xf
else if rdi is :
jmp xd
else if rdi is :
jmp xe
else:
jmp xa
Please do the above with the following constraints:
Assume rdi will NOT be negative
Use no more than cmp instruction
Use no more than jumps of any variant
We will provide you with the number to 'switch' on in rdi.
We will provide you with a jump table base address in rsi.
Here is an example table:
xbxaddrs will change
xxf
xbxd
xxe
xbxa
Please give me your assembly in bytes up to x bytes:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
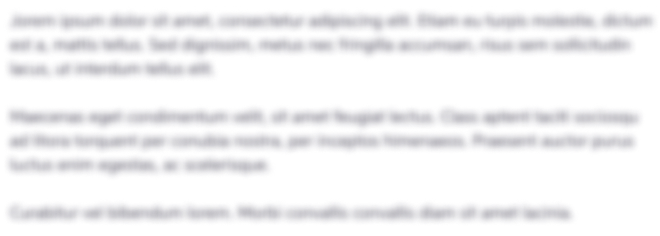
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started