Question
Unit 5 JavaScript - Dom Event and Dom Elements - Just Need JavaScript code - Help Please. CIS 198: JavaScript Learning Unit 5: Activity Lab
Unit 5 JavaScript - Dom Event and Dom Elements - Just Need JavaScript code - Help Please.
element is changed when a user clicks on it: = 'Ya done clicked Click Here
In this example, a function is called from the event handler: Click Here
= CIS 198: JavaScript Learning Unit 5: Handout HTML Event Attributes To assign events to HTML elements you can use event attributes. Assign an onclick event to a button element: In the example above, a function named displayDOB will be executed when the button is clicked. Assign Events Using the HTML DOM The HTML DOM allows you to assign events to HTML elements using JavaScript: Assign an onclick event to a button element: In the example above, a function named displayDOB is assigned to an HTML element with the id="myBtn." The function will be executed when the button is clicked. OFTEN USED EVENTS onload onunload onchange onmouseover onmouseout onmousedown onmouseup onclick onload onfocus Triggered when user enters page Triggered when user leaves page Triggered when a change has occurred Triggered when user mouses over HTML element Triggered when user mouses out of HTML element Triggered when the user clicks down on the mouse Triggered when the mouse button is released Triggered when the mouse click is completed Triggered when the page has finished loading Triggered when an HTML gets focus CIS 198: JavaScript Learning Unit 5: Handout JavaScript HTML DOM EventListener . The addEventListener() method The addEventListener() method attaches an event handler to the specified element. The addEventListener() method attaches an event handler to an element without overwriting existing event handlers. You can add many event handlers to one element. You can add many event handlers of the same type to one element, i.e. two "click" events. You can add event listeners to any DOM object not only HTML elements. I.e. the window object. The addEventListener() method makes it easier to control how the event reacts to bubbling. When using the addEventListener() method, the JavaScript is separated from the HTML markup, for better readability and allows you to add event listeners even when you do not control the HTML markup. You can easily remove an event listener by using the removeEventListener() method. . Syntax element.addEventListener (event, function, useCapture); The first parameter is the type of the event (like "click" or "mousedown"). The second parameter is the function we want to call when the event occurs. The third parameter is a boolean value specifying whether to use event bubbling or event capturing. This parameter is optional. . Note that you don't use the "on" prefix for the event; use "click" instead of "onclick." Add an Event Handler to an Element Alert "Hello World!" when the user clicks on an element: element.addEventListener("click", function () { alert ("Hello!"); }); You can also refer to an external "named" function: Alert "Hello World!" when the user clicks on an element: element.addEventListener("click", myFunction); function myFunction () { alert ("Hello World!"); } CIS 198: JavaScript Learning Unit 5: Handout Add Many Event Handlers to the Same Element The addEventListener() method allows you to add many events to the same element, without overwriting existing events: element.addEventListener("click", myFunction); element.addEventListener("click", mySecondFunction); You can add events of different types to the same element: element.addEventListener ("mouseover", myFunction); element.addEventListener("click", mySecondFunction); element.addEventListener ("mouseout", myThirdFunction); Add an Event Handler to the Window Object The addEventListener() method allows you to add event listeners on any HTML DOM object such as HTML elements, the HTML document, the window object, or other objects that support events, like the xmlHttpRequest object. Add an event listener that fires when a user resizes the window: window.addEventListener("resize", function () { document.getElementById("demo").innerHTML = sometext; }); Passing Parameters When passing parameter values, use an "anonymous function" that calls the specified function with the parameters: element.addEventListener("click", function () { myFunction (p1, p2); }); Event Bubbling or Event Capturing? There are two ways of event propagation in the HTML DOM, bubbling and capturing. Event propagation is a way of defining the element order when an event occurs. If you have a
element inside a
element, which element's "click" event should be handled first? In bubbling the inner most element's event is handled first and then the outer: the
element's click event is handled first, then the
element's click event. With the addEventListener() method you can specify the propagation type by using the "useCapture" parameter: addEventListener (event, function, useCapture); The default value is false, which will use the bubbling propagation, when the value is set to true, the event uses the capturing propagation. Example document.getElementById("myP").addEventListener("click", myFunction, true); document.getElementById("myDiv").addEventListener("click", myFunction, true); The removeEventListener() method The removeEventListener() method removes event handlers that have been attached with the addEventListener() method: element.removeEventListener ("mousemove", myFunction); Browser Support The numbers in the table specifies the first browser version that fully supports these methods. Method addEventListener() removeEventListener() Chrome 1.0 Edge (IE) 9.0 9.0 FireFox 1.0 1.0 Safari 1.0 Opera 7.0 1.0 1.0 7.0 Note: The addEventListener() and removeEventListener() methods are not supported in IE 8 and earlier versions and Opera 6.0 and earlier versions. However, for these specific browser versions, you can use the attachEvent() method to attach an event handlers to the element, and the detachEvent() method to remove it: element.attachEvent (event, function); element.detachEvent (event, function); CIS 198: JavaScript Learning Unit 5: Handout Example Cross-browser solution: // For all major var x = document.getElementById("myBtn"); if (x.addEventListener) { browsers, except IE 8 and earlier x.addEventListener("click", myFunction); } else if (x.attachEvent) { earlier versions x.attachEvent("onclick", myFunction); } 1 For IE 8 and CIS 198: JavaScript Learning Unit 5: Activity Lab Activity 1. Create a folder called Quiz. 2. Within the Quiz" folder create another folder called js. 3. Within the Quiz folder create another folder called images. 4. Download all files located in the JavaScript Data Files folder for LU5 and save in Quiz" folder. 5. Place all images in the images folder and all.js files in the js folder. 6. Open oaetxt.htm. Enter your name and date in the head section and save the file as oae.htm in the Quiz folder. 7. Above the closing tag, insert an external script element that points to the functions contained in the functions.js file. It should look like the following: a. 8. Below this script element, insert opening and closing scripts. . 9. Within the script element declare two variables. The first variable is named "seconds" and will store the current elapsed time that the user has worked on the exam. Set the initial value of the "seconds variable to 0. b. The second variable, clockld, will be used to reference the commands used to repeatedly update the clock value. Do not set an initial value for the "clockld" variable. a. 10. Within the second script create a function named runClock(). The purpose of this function is to update the time value in the Web page's clock. There are no parameters. Add the following commands to the function. Increase the value of the seconds variable by 1. b. Change the value of the quizclock field in the quiz form to the value of the seconds variable. document.getElementById('quizclock').value = seconds; 11. Create a function named startClock(). The purpose of the startClock() function is start the Web page clock and then to repeatedly update elapsed time displayed in the clock. There are no parameters to this function. Add the following commands: a) Call the showQuiz() function to display the questions in the online exam. b) Call the runClock() function every second, storing the ID of this timed-interval command in the clockld variable. clockld = setInterval(runClock, 1000); c) Add line that disables start button. document.getElementById("btnStartClock").disabled=true; 12. Create a function named stopClock(). The purpose of this function is to stop the timer, display the user's score, and disable the exam to prevent further entry. There are no parameters to this function. Add the following commands: a) Halt the repeated calls to the runClock() function (Hint: Use the clearlnterval() method.) b) Call the gradeQuiz() function, storing the value returned by the function in a variable named correctAns. CIS 198: JavaScript Learning Unit 5: Activity c) Display an alert box containing the following text string: You have correctAns correct of 5 in timer seconds. Where correctAns is the value of the correctAns variable and timer is the value of the quizclock field in thequiz form. 13. Locate the input button for the Start Quiz button. Add an event handler attribute that runs the startClock() function when the button is clicked. 14. Go to the bottom of the file and locate the input button for the Submit Answers button. Add an event handler attribute that runs the stopClock() function when the button is clicked. 15. Make the appropriate changes to ensure the path to images and path to JavaScript code is correct. 16. Save your changes to the file. 17. Open oae.htm in your web browser. Verify that by clicking the Start Quiz button the quiz questions are made visible and the timer starts running. Further verify that clicking the Submit Answers button stops the timer, disables the exam, and displays an alert box with the number of correct answers and the elapsed time to complete the exam. Note that to restore the timer and the Web form to its original state, you will have to reload the page in the browser. Clicking the browser's Refresh button will not remove the Web form values or zero the timer. 18. Submit the completed Web site. REMEMBER TO SUBMIT ALL FILES. CIS 198: JavaScript Learning Unit 5: Handout JavaScript HTML DOM Events HTML DOM allows JavaScript to react to HTML events. A JavaScript can be executed when an event occurs, like when a user clicks on an HTML element. Examples of HTML events: . . When a user clicks the mouse When a web page has loaded When an image has been loaded When the mouse moves over an element When an input field is changed When an HTML form is submitted When a user strokes a key . In this example, the content of the
element is changed when a user clicks on it: = 'Ya done clicked Click Here
In this example, a function is called from the event handler: Click Here
= CIS 198: JavaScript Learning Unit 5: Handout HTML Event Attributes To assign events to HTML elements you can use event attributes. Assign an onclick event to a button element: In the example above, a function named displayDOB will be executed when the button is clicked. Assign Events Using the HTML DOM The HTML DOM allows you to assign events to HTML elements using JavaScript: Assign an onclick event to a button element: In the example above, a function named displayDOB is assigned to an HTML element with the id="myBtn." The function will be executed when the button is clicked. OFTEN USED EVENTS onload onunload onchange onmouseover onmouseout onmousedown onmouseup onclick onload onfocus Triggered when user enters page Triggered when user leaves page Triggered when a change has occurred Triggered when user mouses over HTML element Triggered when user mouses out of HTML element Triggered when the user clicks down on the mouse Triggered when the mouse button is released Triggered when the mouse click is completed Triggered when the page has finished loading Triggered when an HTML gets focus CIS 198: JavaScript Learning Unit 5: Handout JavaScript HTML DOM EventListener . The addEventListener() method The addEventListener() method attaches an event handler to the specified element. The addEventListener() method attaches an event handler to an element without overwriting existing event handlers. You can add many event handlers to one element. You can add many event handlers of the same type to one element, i.e. two "click" events. You can add event listeners to any DOM object not only HTML elements. I.e. the window object. The addEventListener() method makes it easier to control how the event reacts to bubbling. When using the addEventListener() method, the JavaScript is separated from the HTML markup, for better readability and allows you to add event listeners even when you do not control the HTML markup. You can easily remove an event listener by using the removeEventListener() method. . Syntax element.addEventListener (event, function, useCapture); The first parameter is the type of the event (like "click" or "mousedown"). The second parameter is the function we want to call when the event occurs. The third parameter is a boolean value specifying whether to use event bubbling or event capturing. This parameter is optional. . Note that you don't use the "on" prefix for the event; use "click" instead of "onclick." Add an Event Handler to an Element Alert "Hello World!" when the user clicks on an element: element.addEventListener("click", function () { alert ("Hello!"); }); You can also refer to an external "named" function: Alert "Hello World!" when the user clicks on an element: element.addEventListener("click", myFunction); function myFunction () { alert ("Hello World!"); } CIS 198: JavaScript Learning Unit 5: Handout Add Many Event Handlers to the Same Element The addEventListener() method allows you to add many events to the same element, without overwriting existing events: element.addEventListener("click", myFunction); element.addEventListener("click", mySecondFunction); You can add events of different types to the same element: element.addEventListener ("mouseover", myFunction); element.addEventListener("click", mySecondFunction); element.addEventListener ("mouseout", myThirdFunction); Add an Event Handler to the Window Object The addEventListener() method allows you to add event listeners on any HTML DOM object such as HTML elements, the HTML document, the window object, or other objects that support events, like the xmlHttpRequest object. Add an event listener that fires when a user resizes the window: window.addEventListener("resize", function () { document.getElementById("demo").innerHTML = sometext; }); Passing Parameters When passing parameter values, use an "anonymous function" that calls the specified function with the parameters: element.addEventListener("click", function () { myFunction (p1, p2); }); Event Bubbling or Event Capturing? There are two ways of event propagation in the HTML DOM, bubbling and capturing. Event propagation is a way of defining the element order when an event occurs. If you have a
element inside a
element, which element's "click" event should be handled first? In bubbling the inner most element's event is handled first and then the outer: the
element's click event is handled first, then the
element's click event. With the addEventListener() method you can specify the propagation type by using the "useCapture" parameter: addEventListener (event, function, useCapture); The default value is false, which will use the bubbling propagation, when the value is set to true, the event uses the capturing propagation. Example document.getElementById("myP").addEventListener("click", myFunction, true); document.getElementById("myDiv").addEventListener("click", myFunction, true); The removeEventListener() method The removeEventListener() method removes event handlers that have been attached with the addEventListener() method: element.removeEventListener ("mousemove", myFunction); Browser Support The numbers in the table specifies the first browser version that fully supports these methods. Method addEventListener() removeEventListener() Chrome 1.0 Edge (IE) 9.0 9.0 FireFox 1.0 1.0 Safari 1.0 Opera 7.0 1.0 1.0 7.0 Note: The addEventListener() and removeEventListener() methods are not supported in IE 8 and earlier versions and Opera 6.0 and earlier versions. However, for these specific browser versions, you can use the attachEvent() method to attach an event handlers to the element, and the detachEvent() method to remove it: element.attachEvent (event, function); element.detachEvent (event, function); CIS 198: JavaScript Learning Unit 5: Handout Example Cross-browser solution: // For all major var x = document.getElementById("myBtn"); if (x.addEventListener) { browsers, except IE 8 and earlier x.addEventListener("click", myFunction); } else if (x.attachEvent) { earlier versions x.attachEvent("onclick", myFunction); } 1 For IE 8 and
Step by Step Solution
There are 3 Steps involved in it
Step: 1
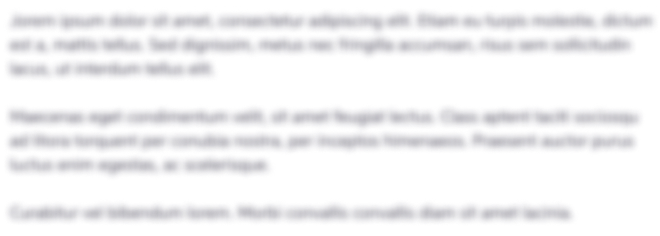
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started