Question
( Unordered Sets ) Redo Programming Exercise 12 of Chapter 12 using templates . redo the files by using templates this is arrayListType.h #ifndef H_arrayListType
(Unordered Sets) Redo Programming Exercise 12 of Chapter 12 using templates.
redo the files by using templates
this is arrayListType.h
#ifndef H_arrayListType #define H_arrayListType #include
using namespace std;
class arrayListType { public: bool isEmpty() const; bool isFull() const; int listSize() const; int maxListSize() const; void print() const; bool isItemAtEqual(int location, int item) const; virtual void insertAt(int location, int insertItem) = 0;
virtual void insertEnd(int insertItem) = 0;
void removeAt(int location);
void retrieveAt(int location, int& retItem) const;
virtual void replaceAt(int location, int repItem) = 0;
void clearList();
virtual int seqSearch(int searchItem) const = 0;
virtual void remove(int removeItem) = 0;
arrayListType(int size = 100);
arrayListType (const arrayListType& otherList); //Copy constructor
virtual ~arrayListType(); //Destructor //Deallocate the memory occupied by the array.
protected: int *list; //array to hold the list elements int length; //variable to store the length of the list int maxSize; //variable to store the maximum //size of the list };
bool arrayListType::isEmpty() const { return (length == 0); } //end isEmpty
bool arrayListType::isFull() const { return (length == maxSize); } //end isFull
int arrayListType::listSize() const { return length; } //end listSize
int arrayListType::maxListSize() const { return maxSize; } //end maxListSize
void arrayListType::print() const { for (int i = 0; i < length; i++) cout << list[i] << " "; cout << endl; } //end print
bool arrayListType::isItemAtEqual(int location, int item) const { if (location < 0 || location >= length) { cout << "The location of the item to be removed " << "is out of range." << endl;
return false; } else return (list[location] == item); } //end isItemAtEqual
void arrayListType::removeAt(int location) { if (location < 0 || location >= length) cout << "The location of the item to be removed " << "is out of range." << endl; else { for (int i = location; i < length - 1; i++) list[i] = list[i+1];
length--; } } //end removeAt
void arrayListType::retrieveAt(int location, int& retItem) const { if (location < 0 || location >= length) cout << "The location of the item to be retrieved is " << "out of range" << endl; else retItem = list[location]; } //end retrieveAt
void arrayListType::clearList() { length = 0; } //end clearList
arrayListType::arrayListType(int size) { if (size <= 0) { cout << "The array size must be positive. Creating " << "an array of the size 100." << endl;
maxSize = 100; } else maxSize = size;
length = 0;
list = new int[maxSize]; } //end constructor
arrayListType::~arrayListType() { delete [] list; } //end destructor
arrayListType::arrayListType(const arrayListType& otherList) { maxSize = otherList.maxSize; length = otherList.length;
list = new int[maxSize]; //create the array
for (int j = 0; j < length; j++) //copy otherList list [j] = otherList.list[j]; }//end copy constructor
#endif
This is main.cpp
#include
using namespace std;
int main() { // Write your main here return 0; }
this is unorderedArrayListType.h
#ifndef H_unorderedArrayListType #define H_unorderedArrayListType
#include
#include "arrayListType.h"
using namespace std; class unorderedArrayListType: public arrayListType { public: virtual void insertAt(int location, int insertItem); virtual void insertEnd(int insertItem); virtual void replaceAt(int location, int repItem); virtual int seqSearch(int searchItem) const; virtual void remove(int removeItem);
unorderedArrayListType(int size = 100); //Constructor };
void unorderedArrayListType::insertAt(int location, int insertItem) { if (location < 0 || location >= maxSize) cout << "The position of the item to be inserted " << "is out of range." << endl; else if (length >= maxSize) //list is full cout << "Cannot insert in a full list" << endl; else { for (int i = length; i > location; i--) list[i] = list[i - 1]; //move the elements down
list[location] = insertItem; //insert the item at //the specified position
length++; //increment the length } } //end insertAt
void unorderedArrayListType::insertEnd(int insertItem) { if (length >= maxSize) //the list is full cout << "Cannot insert in a full list." << endl; else { list[length] = insertItem; //insert the item at the end length++; //increment the length } } //end insertEnd
int unorderedArrayListType::seqSearch(int searchItem) const { int loc; bool found = false;
loc = 0;
while (loc < length && !found) if (list[loc] == searchItem) found = true; else loc++;
if (found) return loc; else return -1; } //end seqSearch
void unorderedArrayListType::remove(int removeItem) { int loc;
if (length == 0) cout << "Cannot delete from an empty list." << endl; else { loc = seqSearch(removeItem);
if (loc != -1) removeAt(loc); else cout << "The item to be deleted is not in the list." << endl; } } //end remove
void unorderedArrayListType::replaceAt(int location, int repItem) { if (location < 0 || location >= length) cout << "The location of the item to be " << "replaced is out of range." << endl; else list[location] = repItem; } //end replaceAt
unorderedArrayListType::unorderedArrayListType(int size) : arrayListType(size) { } //end constructor
#endif
this is unorderedSetType.h
#ifndef H_unorderedSetType #define H_unorderedSetType
#include
#include "unorderedArrayListType.h"
using namespace std;
class unorderedSetType: public unorderedArrayListType { public: void insertAt(int location, int insertItem); void insertEnd(int insertItem); void replaceAt(int location, int repItem);
unorderedSetType(int size = 100); //Constructor };
void unorderedSetType::insertAt(int location, int insertItem) { if (location < 0 || location >= maxSize) cout << "The position of the item to be inserted " << "is out of range." << endl; else if (length >= maxSize) //list is full cout << "Cannot insert in a full list" << endl; else { int loc = seqSearch(insertItem);
if (loc == -1) { for (int i = length; i > location; i--) list[i] = list[i - 1]; //move the elements down
list[location] = insertItem; //insert the item at //the specified position
length++; //increment the length } else cout << "The item to be inserted is already in the list." << endl; } } //end insertAt
void unorderedSetType::insertEnd(int insertItem) { if (length >= maxSize) //the list is full cout << "Cannot insert in a full list." << endl; else { int loc = seqSearch(insertItem);
if (loc == -1) { list[length] = insertItem; length++; //increment the length } else cout << "The item to be inserted is already in the list." << endl; } } //end insertEnd
void unorderedSetType::replaceAt(int location, int repItem) { if (location < 0 || location >= length) cout << "The location of the item to be " << "replaced is out of range." << endl; else { int loc = seqSearch(repItem);
if (loc == -1) list[location] = repItem; else cout << "The item to be inserted is already in the list." << endl; } } //end replaceAt
unorderedSetType::unorderedSetType(int size) : unorderedArrayListType(size) { } //end constructor
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
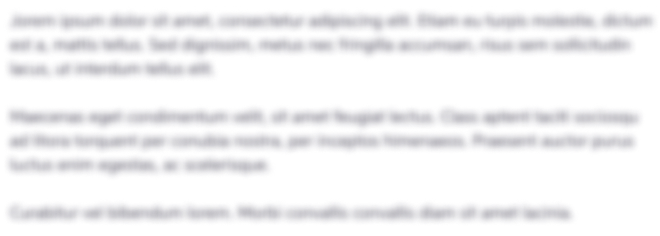
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started