Question
Use Animal.java, GuinnessBook.java, and animalList.txt. public class Animal { private String name; private int topSpeed; //create the getters and setters for the instance variables //there
Use Animal.java, GuinnessBook.java, and animalList.txt.
public class Animal { private String name; private int topSpeed; //create the getters and setters for the instance variables //there is a shortcut by hovering over the variable names // For the setter associated with topSpeed make sure the values are between 0 and 70 // Print "Invalid Speed" if it is not within these values and leave // the speed unchanged. //create a constructor that takes in a String for the name and an int for the topSpeed //use only the setters initialize the instance variables //create an equals(Object other) method that returns True if the two Animal objects have //top speeds that are within 2 mph of each other // Create a toString method // Formatting of the returned String should follow this example: // Name: elephant Top Speed: 25 }
import java.io.File; import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Scanner; public class GuinnessBook { private ArrayListlandAnimals; public GuinnessBook(String filename) throws FileNotFoundException{ landAnimals = new ArrayList (); File animalFile = new File(filename); Scanner scan = new Scanner(animalFile); while(scan.hasNextLine()){ String[] animalInfo = scan.nextLine().split(" "); String animalName = ""; for(int i = 0; i < (animalInfo.length-1); i++){ animalName += animalInfo[i] + " "; } animalName = animalName.trim(); Integer topSpeed = Integer.parseInt(animalInfo[animalInfo.length-1]); //insert the animal into the arraylist here, don't change any of the above code } scan.close(); } public String toString(){ System.out.println("toString() NOT IMPLEMENTED"); return ""; } private void testGuinnessBook() throws FileNotFoundException { // uncomment the println statements to find animals whose speed is within // 2mph of a given speed // System.out.println("Is there an animal whose speed is around 70mph? " + landAnimals.contains(new Animal("fast animal", 70))); // System.out.println("Is there an animal whose speed is around 35mph? " + landAnimals.contains(new Animal("slow animal", 35))); // System.out.println(); // The success of the above two statements depends on correct implementation of an equals method in the Animal class // TAs: please explain this point at some point during the recitation. //If we would like to know which animal it is: // System.out.println(landAnimals.get(landAnimals.indexOf(new Animal("fast animal", 70)))); // System.out.println(landAnimals.get(landAnimals.indexOf(new Animal("slow animal", 35)))); // System.out.println(); //Now print all the animals whose speed is around 50mph } public static void main(String args[]) throws FileNotFoundException { // Once Animal.java is completed and GuinnessBook has a working toString, // uncomment the next two lines to test the constructor and toString // GuinnessBook records = new GuinnessBook(args[0]); // System.out.println(records); // Next, uncomment the following line and lines from testGuinnessBook to test your // Animal class equals method // records.testGuinnessBook(); } }
giraffe 32 pronghorn 60 reindeer 32 mongolian wild 40 wildebeest 56 jackal 35 lion 50 gray fox 42 horse 50 elk 45 mule deer 36 human 27 whippet 35 cape hunting dog 45 coyote 43 hyena 40 cheetah 68 zebra 50 greyhound 45 rabbit 49 gazelle 57
Complete the implementation of Animal.java, by adding the following methods:
1. Getters and setters for name and topSpeed. Print an error if topSpeed is over 70 or below 0 and leave the speed alone.
2. A constructor that takes in a String for the name and an int for the topSpeed. Use the setters to set the instance variables.
3. a toString() method that returns a string with that animal's information. EX: Name: elephant Top Speed: 25
4. an equals(Object other) method that returns True if two animals have the same speed within 2mph, and False otherwise (recall that equals takes in an instance of Object rather than Animal, in order to override the default implementation of equals).
When implementing constructors, getters/setters, toString or equals methods you can use Eclipse to help you write those methods - under the "Source" tab of Eclipse there are options for generating stubs for those methods. In GuinnessBook.java:
1. Complete the code for the constructor. You just need to add code that adds the animals to the landAnimals arrayList.
2. The toString() method. This can make use of Animal's toString()method. It should return a string representation of all the elements in the arrayList. EX:
Name: giraffe Top Speed: 32
Name: pronghorn Top Speed: 61
Name: reindeer Top Speed: 32
Test your code in the main method.
Follow the instructions in main and testGuinnessBook
Step by Step Solution
There are 3 Steps involved in it
Step: 1
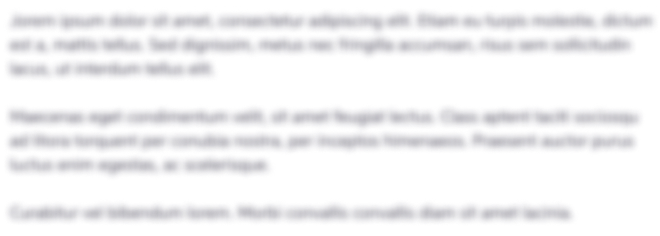
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started