Answered step by step
Verified Expert Solution
Question
1 Approved Answer
USE C + + : Implement a generic stack container. Implement a program that converts infix expression to postfix expression and implement a program that
USE C: Implement a generic stack container. Implement a program that converts
infix expression to postfix expression and implement a program that evaluates postfix
expression using the stack container you developed.
Project Description:
A stack is a type of data containerdata structure that implements the LASTINFIRSTOUT
LIFO strategy for inserting and recovering data. This is a very useful strategy, related to many
types of natural programming tasks.
Remember that in the generic programming paradigm, every data structure is supposed to
provide encapsulation of the data collection, enabling the programmer to interact with the entire
data structure in a meaningful way as a container of data. By freeing the programmer from
having to know its implementation details and only exporting the interface of its efficient
operations, a generic Stack provides separation of data accessmanipulation from internal data
representation. Programs that access the generic Stack only through the interface can be re
used with any other Stack implementation, which results in modular programs with clear
functionality and that are more manageable
Goals:
Implement a generic Stack.
Write a program that parses Infix arithmetic expressions to Postfix arithmetic
expressions using a Stack.
Write a program that evaluates Postfix arithmetic expressions using a Stack.
Task : Implement a GenericStack:
GenericStack: MUST store elements internally in a BasicContainer. It should use only the public
interface to BasicContainer, as you will compilelink your GenericStack implementation with the
BasicContainer class. Therefore, in particular:
GenericStack MUST:
o be able to store elements of an arbitrary type.
o have a noargument constructor that initializes it to some size. This size MUST
NOT be so large as to prevent a calling program to initialize several
GenericStack instances in a single execution. Suggested length values are
or
Every GenericStack instance holding n elements MUST accept insertions as long as the
system has enough free memory to dynamically allocate an array of n elements at
insertion request time, regardless of the initial capacity of the
GenericStack. If the system does not have such free memory, GenericStack MUST
report an error message as the result of the insertion request.
GenericStack MUST implement the full interface specified below.
You MUST provide both the template in a class named GenericStack.h and the
implementation in a class named GenericStack.cpp
Interface:
The interface of GenericStack is specified below. It provides the following public functionality, all
of which can be efficiently achieved using an internal array representation for the data.
GenericStack: noargument constructor. Initializes the Stack to some prespecified capacity.
~GenericStack : destructor.
GenericStack const GenericStack &: copy constructor.
GenericStack& operatorconst GenericStack &: assignment operator.
bool empty const: returns true if the GenericStack contains no elements, and false otherwise.
void pushconst T& x: adds x to this GenericStack.
void pop: removes and discards the most recently added element of the GenericStack.
T& top: mutator that returns a reference to the most recently added element of the
GenericStack.
const T& top const: accessor that returns the most recently added element of the
GenericStack.
int size: returns the number of elements stored in this GenericStack.
void printstd::ostream& os char ofc const: print this instance of GenericStack to
ostream os Note that gsprint prints elements in opposite order as if bcprint was invoked in
the underlying BasicContainer.
The following nonmember functions should also be supported:
std::ostream& operatorstd::ostream& osconst GenericStack& a: invokes the print
method to print the GenericStack a in the specified ostream.
bool operatorconst GenericStack&const GenericStack &: returns true if the two
compared "GenericStack"s have the same elements, in the same order.
bool operatorconst GenericStack& a const GenericStack & b: returns true if every
element in GenericStack a is smaller than corresponding elements of GenericStatck b ie if
repeatedly invoking top and pop on both a and b will generate a sequence of elements ai
from a and bi from b and for every i ai bi until a is empty.USE C
Step by Step Solution
There are 3 Steps involved in it
Step: 1
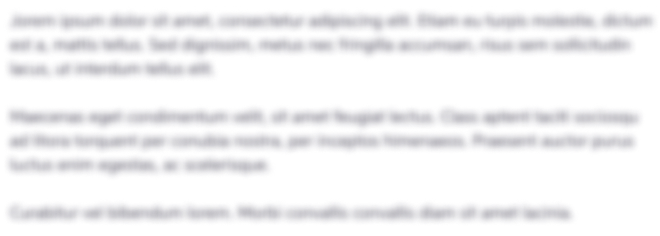
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started