Question
use C# LinkedListLibrary is below the code // Fig. 19.4: LinkedListLibrary.cs // ListNode, List and EmptyListException class declarations. using System; namespace LinkedListLibrary { // class
use C#
LinkedListLibrary is below the code
// Fig. 19.4: LinkedListLibrary.cs
// ListNode, List and EmptyListException class declarations.
using System;
namespace LinkedListLibrary
{
// class to represent one node in a list
class ListNode
{
// automatic read-only property Data
public object Data { get; private set; }
// automatic property Next
public ListNode Next { get; set; }
// constructor to create ListNode that refers to dataValue
// and is last node in list
public ListNode(object dataValue) : this(dataValue, null) { }
// constructor to create ListNode that refers to dataValue
// and refers to next ListNode in List
public ListNode(object dataValue, ListNode nextNode)
{
Data = dataValue;
Next = nextNode;
}
}
// class List declaration
public class List
{
private ListNode firstNode;
private ListNode lastNode;
private string name; // string like "list" to display
// construct empty List with specified name
public List(string listName)
{
name = listName;
firstNode = lastNode = null;
}
// construct empty List with "list" as its name
public List() : this("list") { }
// Insert object at front of List. If List is empty,
// firstNode and lastNode will refer to same object.
// Otherwise, firstNode refers to new node.
public void InsertAtFront(object insertItem)
{
if (IsEmpty())
{
firstNode = lastNode = new ListNode(insertItem);
}
else
{
firstNode = new ListNode(insertItem, firstNode);
}
}
// Insert object at end of List. If List is empty,
// firstNode and lastNode will refer to same object.
// Otherwise, lastNode's Next property refers to new node.
public void InsertAtBack(object insertItem)
{
if (IsEmpty())
{
firstNode = lastNode = new ListNode(insertItem);
}
else
{
lastNode = lastNode.Next = new ListNode(insertItem);
}
}
// remove first node from List
public object RemoveFromFront()
{
if (IsEmpty())
{
throw new EmptyListException(name);
}
object removeItem = firstNode.Data; // retrieve data
// reset firstNode and lastNode references
if (firstNode == lastNode)
{
firstNode = lastNode = null;
}
else
{
firstNode = firstNode.Next;
}
return removeItem; // return removed data
}
// remove last node from List
public object RemoveFromBack()
{
if (IsEmpty())
{
throw new EmptyListException(name);
}
object removeItem = lastNode.Data; // retrieve data
// reset firstNode and lastNode references
if (firstNode == lastNode)
{
firstNode = lastNode = null;
}
else
{
ListNode current = firstNode;
// loop while current.Next is not lastNode
while (current.Next != lastNode)
{
current = current.Next; // move to next node
}
// current is new lastNode
lastNode = current;
current.Next = null;
}
return removeItem; // return removed data
}
// return true if List is empty
public bool IsEmpty()
{
return firstNode == null;
}
// output List contents
public void Display()
{
if (IsEmpty())
{
Console.WriteLine($"Empty {name}");
}
else
{
Console.Write($"The {name} is: ");
ListNode current = firstNode;
// output current node data while not at end of list
while (current != null)
{
Console.Write($"{current.Data} ");
current = current.Next;
}
Console.WriteLine(" ");
}
}
}
// class EmptyListException declaration
public class EmptyListException : Exception
{
// parameterless constructor
public EmptyListException() : base("The list is empty") { }
// one-parameter constructor
public EmptyListException(string name)
: base($"The {name} is empty") { }
// two-parameter constructor
public EmptyListException(string exception, Exception inner)
: base(exception, inner) { }
}
}
A) You need to enhance your generic LinkedListLibrary (.dll file ) class library project ( which has been completed in the class/Lab), by adding the following methods apart from the existing methods. (Note: Create a new solution, and to that solution add a new class library project. Enhance it as per requirements.:) 1. T Minimum() method which will return the smallest itemode value [5 marks] 2. T GetLastNode() which will return the last element in the linked list [5 marks] After that add another project- LinkedListLibraryTest to the above solution where you can test the above library by adding a reference to test project. Take out the build of the above library in the Release Mode.] - Test this library by using it in the project LinkedListLibraryTest by creating two linked lists of integers and doubles ( containing at least 5 elements each ) and calling the methods Minimum() and GetLastNode() B) You need to enhance class library project QueuelnheritanceLibrary ( generic version), which is derived from Linked ListLibrary, by adding following method apart from the existing ones: - T GetLast() which will just return the last element in the queue and not delete it. [2.5 marks] C) Test this library by using it in the project - QueuelnheritanceLibraryTest by creating two linked lists based queue objects of integers and doubles and calling the method GetLast(). [2.5 marks]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
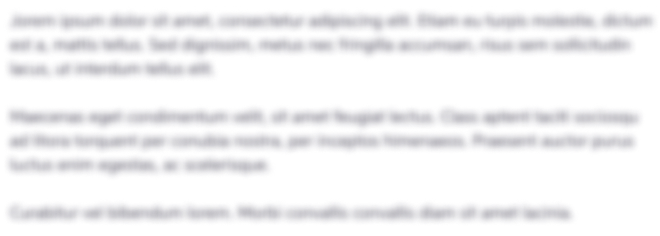
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started