Question
Use Java Please Using the Class Account on Pages 192 to 193 (if you have a different edition then the page numbers will be different),
Use Java Please
Using the Class Account on Pages 192 to 193 (if you have a different edition then the page numbers will be different), write a menu driven driver program that simulates the options for a Savings Account. If you don't have a text yet, you will also find the source code for Class Account in the file above the drop box.
1. Instantiate an instance of Class Account for one customer in your Driver.
2. Hard code the following information to initialize the account:
- Customer Name ("First Last")
- Account number
- Initial Balance
NOTE: To hard code data means that the programmer assigns the data to the corresponding variables within the body of the program. This is usually done when the program is first executed - but before any other tasks occur. For this assignment, hard code the data before the menu loop begins.
3. Prompt the User to enter her/his account number.
4. Display an error message if the account number does not exist.
5. Display the menu below if the account number is valid.
Your menu will offer the following options:
- Deposit
- Withdrawal
- Returns Balance
- Returns Balance with Interest
- Returns Account Number
- Returns all Account Information
- Exit
6. Be sure to place the menu within a loop so that thorough testing can be done. Be sure to test each and every method to make sure it works before turning in your completed assignment. See the document below for tips on how to test the each method.
This assignment will have two separate files - the Class Account file that is provided and the Class Driver file that you create.
//******************************************************************** // Account.java Java Foundations // // Represents a bank account with basic services such as deposit // and withdraw. //******************************************************************** import java.text.NumberFormat; public class Account { private final double RATE = 0.035; // interest rate of 3.5% private String name; private long acctNumber; private double balance; //----------------------------------------------------------------- // Sets up this account with the specified owner, account number, // and initial balance. //----------------------------------------------------------------- public Account (String owner, long account, double initial) { name = owner; acctNumber = account; balance = initial; } //----------------------------------------------------------------- // Deposits the specified amount into this account and returns // the new balance. The balance is not modified if the deposit // amount is invalid. //----------------------------------------------------------------- public double deposit (double amount) { if (amount > 0) balance = balance + amount; return balance; } //----------------------------------------------------------------- // Withdraws the specified amount and fee from this account and // returns the new balance. The balance is not modified if the // withdraw amount is invalid or the balance is insufficient. //----------------------------------------------------------------- public double withdraw (double amount, double fee) { if (amount+fee > 0 && amount+fee < balance) balance = balance - amount - fee; return balance; } //----------------------------------------------------------------- // Adds interest to this account and returns the new balance. //----------------------------------------------------------------- public double addInterest () { balance += (balance * RATE); return balance; } //----------------------------------------------------------------- // Returns the current balance of this account. //----------------------------------------------------------------- public double getBalance () { return balance; } //----------------------------------------------------------------- // Returns a one-line description of this account as a string. //----------------------------------------------------------------- public String toString () { NumberFormat fmt = NumberFormat.getCurrencyInstance(); return (acctNumber + "\t" + name + "\t" + fmt.format(balance)); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
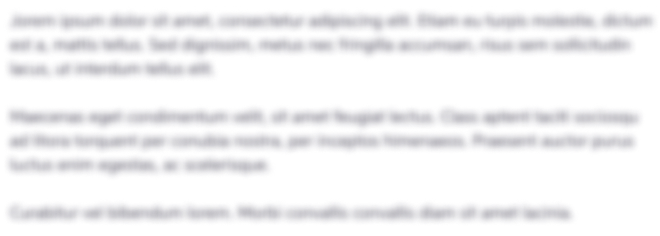
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started