Question
Use Python 3 to build a 2048 Game: you must complete the collapseRow() function. This function is given a list of numbers as an argument
Use Python 3 to build a 2048 Game:
you must complete the collapseRow() function. This function is given a list of numbers as an argument and it should return a LEFT-collapsed list, and a True if the list was collapsed or False if it was not. The code for your function must collapse the list conforming to the rules of 2048. That is, if it is passed the list [2, 0, 2, 4], after collapsing to the left the function should return the list [4, 4, 0, 0].
i.e. if a = [2, 0, 2, 0], it should return [4, 0, 0, 0] if b = [2, 2, 2, 0], it should return [4, 2, 0, 0]
import random as rnd import os import sys
class Grid(): def __init__(self, row=4, col=4, initial=2): self.row = row # number of rows in grid self.col = col # number of columns in grid self.initial = initial # number of initial cells filled self.score = 0
self._grid = self.createGrid(row, col) # creates the grid specified above
self.emptiesSet = list(range(row * col)) # list of empty cells
for _ in range(self.initial): # assignation to two random cells self.assignRandCell(init=True)
def createGrid(self, row, col): row_list= [] for row_index in range(row): col_list= [] for col_index in range(col): col_list.append(self.score) row_list.append(col_list) return row_list
def setCell(self, cell, val): x = 0 while cell >= self.col: cell -= self.col x += 1 self._grid[x][cell] = val
def getCell(self, cell):
x = 0 while cell >= self.col: cell -= self.col x += 1 return self._grid[x][cell]
def assignRandCell(self, init=False):
if len(self.emptiesSet): cell = rnd.sample(self.emptiesSet, 1)[0] if init: self.setCell(cell, 2) else: cdf = rnd.random() if cdf > 0.75: self.setCell(cell, 4) else: self.setCell(cell, 2) self.emptiesSet.remove(cell)
def drawGrid(self):
for i in range(self.row): line = '\t|' for j in range(self.col): if not self.getCell((i * self.row) + j): line += ' '.center(5) + '|' else: line += str(self.getCell((i * self.row) + j)).center(5) + '|' print(line) print()
def collapsible(self):
for i in self._grid: for item in i: if item == 0: return True for i in self._grid: if i[0] == i[1] or i[1] == i[2] or i[2] == i[3]: return True for i in range(self.col): if self._grid[0][i] == self._grid[1][i] or self._grid[1][i] == self._grid[2][i] or self._grid[2][i] == self._grid[3][i]: return True
def collapseRow(self, lst):
This function takes a list lst and collapses it to the LEFT.
This function should return two values: 1. the collapsed list and 2. True if the list is collapsed and False otherwise.
class Game():
def __init__(self, row=4, col=4, initial=2):
self.game = Grid(row, col, initial) self.play()
def printPrompt(self):
if sys.platform == 'win32':
os.system("cls") else: os.system("clear")
print('Press "w", "a", "s", or "d" to move Up, Left, Down or Right respectively.') print('Enter "p" to quit. ') self.game.drawGrid() print(' Score: ' + str(self.game.score))
def play(self):
moves = {'w' : 'Up', 'a' : 'Left', 's' : 'Down', 'd' : 'Right'}
stop = False collapsible = True
while not stop and collapsible: self.printPrompt() key = input(' Enter a move: ')
while not key in list(moves.keys()) + ['p']: self.printPrompt() key = input(' Enter a move: ')
if key == 'p': stop = True else: move = getattr(self.game, 'collapse' + moves[key]) collapsed = move()
if collapsed: self.game.updateEmptiesSet() self.game.assignRandCell()
collapsible = self.game.collapsible()
if not collapsible: if sys.platform == 'win32': os.system("cls") else: os.system("clear") print() self.game.drawGrid() print(' Score: ' + str(self.game.score)) print('No more legal moves.')
def main(): game = Game()
main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
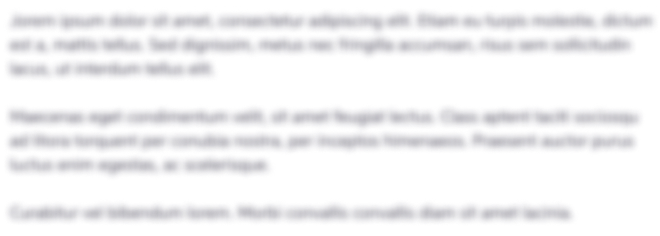
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started