Question
USE PYTHON3; DO NOT IMPORT PACKAGES Please do all of part two(everything in the images) and pay careful attention to the requirements for the functions.
USE PYTHON3; DO NOT IMPORT PACKAGES
Please do all of part two(everything in the images) and pay careful attention to the requirements for the functions. Please dont add or remove any parameters. Thank you!
In this part, you will implement several image processing methods in the ImageProcessing class.
Notes:
-
All methods in this class have a decorator @staticmethod. This decorator indicates that these functions do not belong to any instances, and should be called by the class itself. For example, to use the function negate(), you should call it like ImageProcessing.negate(image), instead of initializing an ImageProcessing instance first.
-
All methods in this class must return a new RGBImage instance with the processed pixels matrix. After calling any of these methods, the original image should not be modified.
Hint:
If you find processing 3-dimensional pixels difficult, try to approach these problems in a 2-dimensional perspective (pick any color channel to work with first) and broadcast your solution to all three channels. If you are stuck, try to write down the (row, column) matrix before and after applying the function and derive a pattern.
You need to implement the following methods:
The format of the code in the text editor:
# Part 2: Image Processing Methods # class ImageProcessing: """ TODO: add description """
@staticmethod def negate(image): """ TODO: add description """ # YOUR CODE GOES HERE #
@staticmethod def grayscale(image): """ TODO: add description """ # YOUR CODE GOES HERE #
@staticmethod def clear_channel(image, channel): """ TODO: add description """ # YOUR CODE GOES HERE #
@staticmethod def crop(image, tl_row, tl_col, target_size): """ TODO: add description """ # YOUR CODE GOES HERE #
@staticmethod def chroma_key(chroma_image, background_image, color): """ TODO: add description """ # YOUR CODE GOES HERE #
Here's the link to the already answered part 1 of this assignment if it helps: https://www.chegg.com/homework-help/questions-and-answers/use-python3-import-packages-please-part-1-everything-images-don-t-change-parameters-adding-q69181518?trackid=Cl4ktDAx
You need to implement the following methods: @staticmethod negate (image) A method that returns the negative image of the given image. To produce a negative image, all pixel values must be inverted. Specifically, for each pixel with current intensity value vol, this method should update it with (255 - vol). Requirement: No explicit for/while loops. You can use list comprehensions or map O instead. Example: image negate (image) DSC DSC 20 20 @staticmethod grayscale (image) A method that converts the given image to grayscale. For each pixel (R, G, B) in the pixels matrix, calculate the average (R + G + B) / 3 and update all channels with this average, i.e. (R, G, B) -> ((R + G + B) / 3, (R + G + B) / 3, (R G = B) / 3). Note that since intensity values must be integer, you should use the integer division Requirement: No explicit for/while loops. You can use list comprehensions or map() instead. Example: image grayscale (image) 63 DSC 20 20 @staticmethod clear_channel (image, channel) A method that clears the given channel of the image. By clearing a channel, you need to update every intensity value in the specified channel to 0. Requirement: No explicit for/while loops. You can use list comprehensions or map instead. Example: image clear_channel (image, 0) clear_channel (image, 2) DSC DSC 20 20 DSC 20 @staticmethod crop (image, tl_row, tl_col, target_size) A method that crops the image. Arguments tl_row and t2_col specify the position of the top-left corner of the cropped image. In other words, position (tl_row, tl_col) before cropping becomes position (0,0) after cropping. The argument target_size specifies the size of the image after cropping. It is a tuple of (number of rows, number of columns). However, when the specified target_size is too large, the actual size of the cropped image might be smaller, since the original image has no content in the overflowed rows and columns. Tip: When a target_size (n_rows, n_cols) is possible to achieve given the original size of the image, tl_row, and tl_col, (tl_row + n_rows) and (tl_col + n_cols) give you br_row and br_col, which is the position of the bottom-right corner of the cropped image. Requirement: No explicit for/while loops. You can use list comprehensions or map() instead. Example: image crop (image, 50, 75, (75, 50)) crop (image, 100, 50, (100, 150)) EDT DSC 20 actual size = (75, 50), actual size = (90, 140), target_size does not target_size overflows overflow both row and column, thus the actual size is smaller in both dimensions. size = (190, 190) @staticmethod chroma_key (chroma_image, background_image, color) A method that performs the chroma key algorithm on the chroma_image by replacing all pixels with the specified color in the chroma_image to the pixels at the same places in the background_image. If the color does not present in the chroma_image, this function won't replace any pixel, but it will still return a copy. You can assume that color is a valid (R, G, B) tuple. Tip: When testing this function, you can find pictures with a green or blue screen in the background online, find your favorite pictures as background images, and use this function to replace the background color with the background image you choose. Make sure you crop them to the same size before applying this function. Requirement: Assert that chroma_image and background_image are RGBImage instances and have the same size. Example: chroma image background color color image (255, 255, 255) (255, 205, 210) = DSC 20 DSC 20 DSC 20, (white background (pink font color replaced) replaced) You need to implement the following methods: @staticmethod negate (image) A method that returns the negative image of the given image. To produce a negative image, all pixel values must be inverted. Specifically, for each pixel with current intensity value vol, this method should update it with (255 - vol). Requirement: No explicit for/while loops. You can use list comprehensions or map O instead. Example: image negate (image) DSC DSC 20 20 @staticmethod grayscale (image) A method that converts the given image to grayscale. For each pixel (R, G, B) in the pixels matrix, calculate the average (R + G + B) / 3 and update all channels with this average, i.e. (R, G, B) -> ((R + G + B) / 3, (R + G + B) / 3, (R G = B) / 3). Note that since intensity values must be integer, you should use the integer division Requirement: No explicit for/while loops. You can use list comprehensions or map() instead. Example: image grayscale (image) 63 DSC 20 20 @staticmethod clear_channel (image, channel) A method that clears the given channel of the image. By clearing a channel, you need to update every intensity value in the specified channel to 0. Requirement: No explicit for/while loops. You can use list comprehensions or map instead. Example: image clear_channel (image, 0) clear_channel (image, 2) DSC DSC 20 20 DSC 20 @staticmethod crop (image, tl_row, tl_col, target_size) A method that crops the image. Arguments tl_row and t2_col specify the position of the top-left corner of the cropped image. In other words, position (tl_row, tl_col) before cropping becomes position (0,0) after cropping. The argument target_size specifies the size of the image after cropping. It is a tuple of (number of rows, number of columns). However, when the specified target_size is too large, the actual size of the cropped image might be smaller, since the original image has no content in the overflowed rows and columns. Tip: When a target_size (n_rows, n_cols) is possible to achieve given the original size of the image, tl_row, and tl_col, (tl_row + n_rows) and (tl_col + n_cols) give you br_row and br_col, which is the position of the bottom-right corner of the cropped image. Requirement: No explicit for/while loops. You can use list comprehensions or map() instead. Example: image crop (image, 50, 75, (75, 50)) crop (image, 100, 50, (100, 150)) EDT DSC 20 actual size = (75, 50), actual size = (90, 140), target_size does not target_size overflows overflow both row and column, thus the actual size is smaller in both dimensions. size = (190, 190) @staticmethod chroma_key (chroma_image, background_image, color) A method that performs the chroma key algorithm on the chroma_image by replacing all pixels with the specified color in the chroma_image to the pixels at the same places in the background_image. If the color does not present in the chroma_image, this function won't replace any pixel, but it will still return a copy. You can assume that color is a valid (R, G, B) tuple. Tip: When testing this function, you can find pictures with a green or blue screen in the background online, find your favorite pictures as background images, and use this function to replace the background color with the background image you choose. Make sure you crop them to the same size before applying this function. Requirement: Assert that chroma_image and background_image are RGBImage instances and have the same size. Example: chroma image background color color image (255, 255, 255) (255, 205, 210) = DSC 20 DSC 20 DSC 20, (white background (pink font color replaced) replaced)Step by Step Solution
There are 3 Steps involved in it
Step: 1
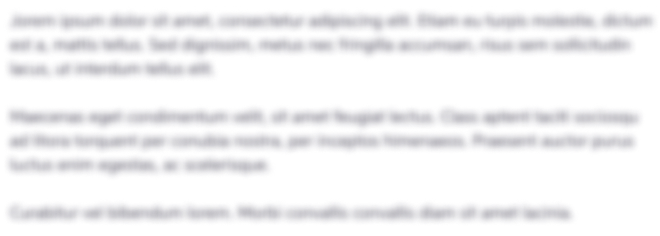
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started