Question
Use the Average Waiting Time program to determine a reasonable number of queues to use if there are 1000 customers and: a) The inner-arrival time
Use the Average Waiting Time program to determine a reasonable number of queues to use if there are 1000 customers and:
a) The inner-arrival time is 5 and the service time is 5
b) The inner-arrival time is 1 and the service time is 5
c) The inner-arrival time ranges from 0 to 20, and the service time ranges from 20 to 100
d) The inner-arrival time ranges from 0 to 2, and the service time ranges from 20 to 100
In each case, descripe how you arrived at your result
//--------------------------------------------------------------------------- // ArrayUnbndQueue.java by Dale/Joyce/Weems Chapter 5 // // Implements UnboundedQueueInterface with an array to hold queue elements. // // Two constructors are provided; one that creates a queue of a default // original capacity and one that allows the calling program to specify the // original capacity. // // If an enqueue is attempted when there is no room available in the array, a // new array is created, with capacity incremented by the original capacity. //---------------------------------------------------------------------------
package ch05.queues;
public class ArrayUnbndQueue
public ArrayUnbndQueue() { queue = (T[]) new Object[DEFCAP]; rear = DEFCAP - 1; origCap = DEFCAP; }
public ArrayUnbndQueue(int origCap) { queue = (T[]) new Object[origCap]; rear = origCap - 1; this.origCap = origCap; }
private void enlarge() // Increments the capacity of the queue by an amount // equal to the original capacity. { // create the larger array T[] larger = (T[]) new Object[queue.length + origCap]; // copy the contents from the smaller array into the larger array int currSmaller = front; for (int currLarger = 0; currLarger < numElements; currLarger++) { larger[currLarger] = queue[currSmaller]; currSmaller = (currSmaller + 1) % queue.length; } // update instance variables queue = larger; front = 0; rear = numElements - 1; }
public void enqueue(T element) // Adds element to the rear of this queue. { if (numElements == queue.length) enlarge();
rear = (rear + 1) % queue.length; queue[rear] = element; numElements = numElements + 1; }
public T dequeue() // Throws QueueUnderflowException if this queue is empty; // otherwise, removes front element from this queue and returns it. { if (isEmpty()) throw new QueueUnderflowException("Dequeue attempted on empty queue."); else { T toReturn = queue[front]; queue[front] = null; front = (front + 1) % queue.length; numElements = numElements - 1; return toReturn; } }
public boolean isEmpty() // Returns true if this queue is empty; otherwise, returns false { return (numElements == 0); } }
//---------------------------------------------------------------------------- // UnboundedQueueInterface.java by Dale/Joyce/Weems Chapter 5 // // Interface for a class that implements a queue of T with no bound // on the size of the queue. A queue is a "first in, first out" structure. //----------------------------------------------------------------------------
package ch05.queues;
public interface UnboundedQueueInterface
{ void enqueue(T element); // Adds element to the rear of this queue. }
//---------------------------------------------------------------------------- // QueueInterface.java by Dale/Joyce/Weems Chapter 5 // // Interface for a class that implements a queue of T. // A queue is a "first in, first out" structure. //----------------------------------------------------------------------------
package ch05.queues;
public interface QueueInterface
{ T dequeue() throws QueueUnderflowException; // Throws QueueUnderflowException if this queue is empty; // otherwise, removes front element from this queue and returns it.
boolean isEmpty(); // Returns true if this queue is empty; otherwise, returns false. }
AWT model
public class GlassQueue
public class ArrayUnbndQueue
public interface UnboundedQueueInterface
{
void enqueue(T element);
//Adds element to the rear of this quee.
}
public interface QueueInterface
{
T dequeue() throws QueueUnderflowException;
//Throws QueueUnderflowException if this queue is empty;
//otherwise, removes front element from this queue and returns it
boolean isEmpty();
//Returns true if this queue is empty; otherwise, return false
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
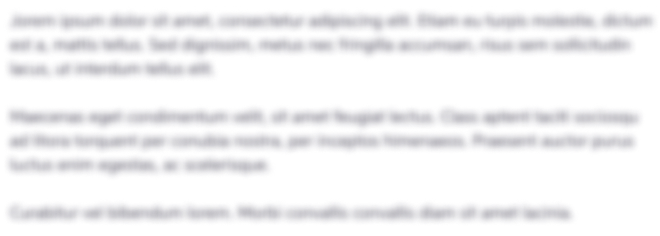
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started