Question
Using C# create a new backing field called _speedIncrement. Inside the constructor initialize this backing field to 5. Adjust other parts of the code for
Using C# create a new backing field called _speedIncrement. Inside the constructor initialize this backing field to 5. Adjust other parts of the code for this new dynamic variable. Then create a new property method called "SetIncrement", to allow the user to change the increment in the class. Incremint has to be an int value and cant be less than 1 or greater than 20. If the value the user enters is not less than 1 or greater than 20, issue a messagebox from within the class which tells the user to re enter a current number. In Form1 add a labelbox, textbox, and a button to allow the user to change the speed increment. In the class' Speed property, before changing the backing speed to its new value, check and see if it exceeds 100mph. If it does, set the value to 100. Issue a messagebox message, from within the class, stating the you have exceeded the maximum speed of the car.
Code to build off of:
Form1:
namespace speedtest { public partial class Form1 : Form { public Form1() { InitializeComponent(); labelMake.Text = car.Make; labelYear.Text = car.Year.ToString(); labelSpeed.Text = car.Speed.ToString(); }
private Car car = new Car(2018, "Wrangler");
private void button2_Click(object sender, EventArgs e)
{ car.Brake(); labelSpeed.Text = car.Speed.ToString(); }
private void buttonAccelerate_Click(object sender, EventArgs e) { car.Accelerate(); labelSpeed.Text = car.Speed.ToString(); }
} }
Car Class
namespace speedtest
{ class Car { private string _Make; private int _Year; private int _Speed;
public Car(int Year, string Make) { _Year = Year; _Make = Make; _Speed = 0; } public int Year { get { return _Year; } set { _Year = value; } }
public string Make { get { return _Make; } set { _Make = value; } }
public void Accelerate() { _Speed = _Speed + 5; }
public void Brake() { _Speed = _Speed - 5; }
public int Speed { get { return _Speed; } set { _Speed = value; } } } }
Form1 Make Year Accelerate Speed: 0Step by Step Solution
There are 3 Steps involved in it
Step: 1
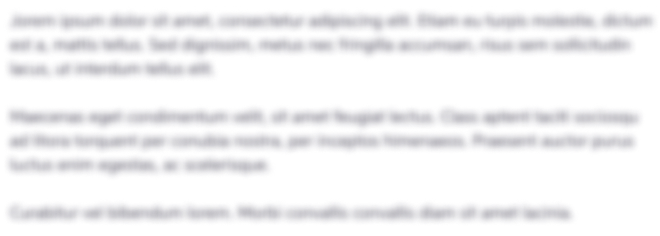
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started