Question
Using C++, Python, Swift or Java, write a program that: In this programming exercise you will perform an empirical analysis of the QuickSort algorithm to
Using C++, Python, Swift or Java, write a program that:
In this programming exercise you will perform an empirical analysis of the QuickSort algorithm to study the actual average case behavior and compare it to the mathematically predicted behavior. That is, you will write a program that counts the number of comparisons performed by QuickSort on an array of a given size. You will run the program on a large number of arrays of a certain size and determine the average number of comparisons performed. You will do this for several different sizes and compare the observed results with what is expected.
Using C++, Python, Swift or Java, write a program that:
prompts the user to enter the number n, of arrays to be processed and the size m, of each array
creates an array, of the given size, containing the integers between 1 and m
for the given number of arrays
randomly unsorts the array
sorts the array using the version of QuickSort developed in your text and in class
counts the number of comparisons performed during the partitioning subroutine of the sort
calculates the average number of comparisons performed in processing all the arrays
You will need to use the versions of QuickSort and Partition given in your text since that was what was used to derive the predicted average complexity you will be comparing your results with.
The program should output the number of arrays processed, the size of the arrays processed and the average number of comparisons per array.
For example, if the user enters 50 and 100 the output would be similar to:
# of arrays processed: 50
# of items in each array: 100
average number of comparisons: 926.02 (Your results may be different!)
The following algorithm can be used to unsort an array A, of size m.
1) FOR i := 1 to m
2) Generate a random number r, between 1 and m
3) Swap A[i] and A[r]
Use your program to determine the average number of comparisons performed in sorting 1000 arrays for each of the array sizes, 10, 50, 100, 500, 1000, 5000, 10,000, 50,000 and 100,000. Compare the observed average with the average predicted by the derivation of the average-case time complexity in section 2.4 of your text. Display your results in a table comparing the predicted average with the observed average. You will want to look at the percent difference between the predicted and observed values. Write a paragraph discussing your conclusions about any differences you notice and what they tell you about the actual asymptotic behavior of the quick sort.
Incremental Development
It is usually not a good idea to try to code a complicated algorithm from beginning to end. Taking a few extra minutes to create your program incrementally can save you HOURS of debugging. In developing this program, I would suggest the following approach:
Write functions that implement QuickSort and Partition as given in your text.
Write a main function that creates the array of exercise 19 on p. 91 and sorts it using your QuickSortfunction.
Add code to count the number of comparisons performed during the sort. This will require an additional parameter to both QuickSort and Partition.
Check that the number of comparisons used to sort the array of exercise 19 on p. 91 is what it should be.
Write your unsort function and test it thoroughly.
Change main so that it prompts the user for the size m, of the array, creates an array of size m that contains the integers 1 to m, unsorts it, then uses QuickSort to sort it and counts the comparisons.
Finally, change main so that it prompts the user for the number of arrays n, to test and prints the average number of comparisons done.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
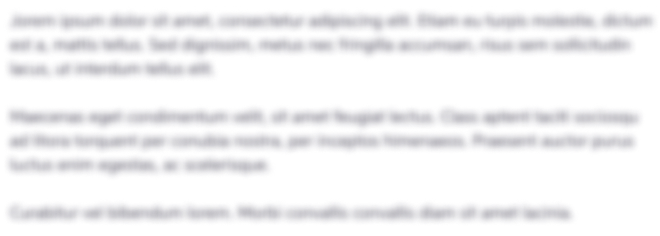
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started