Question
Using inheritance for the World of Zuul project, create a TransporterRoom class that inherits the Room class and transports you to another room Some pieces
Using inheritance for the World of Zuul project, create a TransporterRoom class that inherits the Room class and transports you to another room
Some pieces of code that may help:
import java.util.Scanner;
public class Game { private Room currentRoom; public Game() { createRooms(); }
/** * Create all the rooms and link their exits together. */ private void createRooms() { Room outside, theater, teleport;
// create the rooms outside = new Room("outside *burp* the main entrance *burp* of the university"); theater = new Room("in a lecture theater"); teleport = new Room("in my space cruiser");
// initialise room exits outside.setExit("east", theater); theater.setExit("west", outside); outside.setExit("north", teleport); teleport.setExit("south", outside);
currentRoom = outside; //start game outside }
/** * Given a command, process (that is: execute) the command. * @param command The command to be processed. * @return true If the command ends the game, false otherwise. */ private boolean processCommand(Command command) { boolean wantToQuit = false;
CommandWord commandWord = command.getCommandWord();
switch (commandWord) { case UNKNOWN: System.out.println("I don't know what you mean..."); break;
case GO: goRoom(command); setRoom(); break; } return wantToQuit; }
/** *Sets the current room for player if the description of *the current location matches the room */ private void setRoom() { String str = currentRoom.getShortDescription().toString();
if (!(str.equals(null))) { if (str.equals("in a lecture theater")) player.setCurrentRoom("theater");
else if (str.equals("outside *burp* the main entrance *burp* of the university")) player.setCurrentRoom("outside");
else if (str.equals("in my space cruiser")) { player.setCurrentRoom("teleport"); } }
else return; } /** * Try to go in a direction. If there is an exit, enter the new * room, otherwise print an error message. * @param command User input the direction desired */ private void goRoom(Command command) { String direction = command.getSecondWord();
// Try to leave current room. Room nextRoom = currentRoom.getExit(direction);
currentRoom = nextRoom; } }
import java.util.Set; import java.util.HashMap;
public class Room { private String description; public HashMap
/** * Create a room * @param description The room's description */ public Room(String description) { this.description = description; exits = new HashMap<>(); }
public void setExit(String direction, Room neighbor) { exits.put(direction, neighbor); }
public String getShortDescription() { return description; }
public Room getExit(String direction) { return exits.get(direction); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
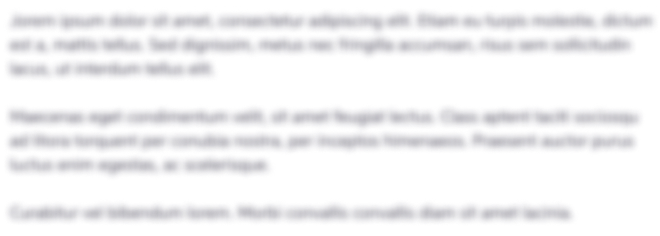
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started