Question
Using Java to create the following classes below. Continue from below, the ChessPiece class and its six subclasses. The ChessPiece class must override the equals()
Using Java to create the following classes below.
Continue from below, the ChessPiece class and its six subclasses.
The ChessPiece class must override the equals() method (and therefore also the hashCode method) as follows:
If two ChessPiece objects have a value within 1 of each other, they are considered equal.
The Pawn class must contain two new instance variables:
boolean hasBeenPromoted;
ChessPiece newPiece;
In the game of chess, when a Pawn reaches the far side of the board, it is exchanged for another ChessPiece; for example, a Pawn can become a Rook, or a Queen, etc. It cannot become a King or Pawn though. Enforce these rules in a new method called public void promote(ChessPiece newPiece).
Override the equals() method of the Pawn class so that Pawns are NOT equal if one has been promoted and another has not. Pawns are also NOT equal if they have been promoted to different ChessPiece types.
Note: Use the @Override annotation every time you override a method.
Please follow the instructions above for a good rate
------------------------------------------------------------------------------------------------------------------
ChessPiece.java ------------------------ public class ChessPiece { private boolean color; private int value; public ChessPiece(boolean color, int value) { this.color = color; this.value = value; } public boolean isColor() { return color; } public void setColor(boolean color) { this.color = color; } public int getValue() { return value; } public void setValue(int value) { this.value = value; } public void move() { System.out.println("moving..."); } @Override public String toString() { return isColor() ? "White" : "Black"; } } ---------------End ------------------------ Pawn.java ----------------------- public class Pawn extends ChessPiece { public Pawn(boolean color, int value) { super(color, value); } @Override public String toString() { return super.toString() + " " + this.getClass().getSimpleName(); } @Override public void move() { System.out.println("forward 1"); } } ----------------------End---------------------------- Knight.java --------------------- public class Knight extends ChessPiece { public Knight(boolean color, int value) { super(color, value); } @Override public String toString() { return super.toString() + " " + this.getClass().getSimpleName(); } @Override public void move() { System.out.println("like an L"); } } --------------------End------------------------ Bishop.java ------------------- public class Bishop extends ChessPiece { public Bishop(boolean color, int value) { super(color, value); } @Override public String toString() { return super.toString() + " " + this.getClass().getSimpleName(); } @Override public void move() { System.out.println("diagonally"); } } ------------------------End---------------------- Rook.java ------------------- public class Rook extends ChessPiece { public Rook(boolean color, int value) { super(color, value); } @Override public String toString() { return super.toString() + " " + this.getClass().getSimpleName(); } @Override public void move() { System.out.println("horizontally or vertically"); } } ------------------------End-------------------------- Queen.java --------------------- public class Queen extends ChessPiece { public Queen(boolean color, int value) { super(color, value); } @Override public String toString() { return super.toString() + " " + this.getClass().getSimpleName(); } @Override public void move() { System.out.println("like a bishop or a rook"); } } -----------------End-------------------------- King.java --------------------------- public class King extends ChessPiece { public King(boolean color, int value) { super(color, value); } @Override public String toString() { return super.toString() + " " + this.getClass().getSimpleName(); } @Override public void move() { System.out.println("one square"); } } --------------------End----------------------------- Main.java
-----------------------
public class Main {
public static void main(String[] args) {
ChessPiece[][] chessBoard = new ChessPiece[8][8];
chessBoard[0][0] = new Rook(false, 5);
chessBoard[0][1] = new Knight(true, 2);
chessBoard[0][2] = new Bishop(false, 3);
chessBoard[0][3] = new Queen(true, 9);
chessBoard[0][4] = new King(false, 1000);
chessBoard[0][5] = new Bishop(true, 3);
chessBoard[0][6] = new Knight(false, 2);
chessBoard[0][7] = new Rook(true, 5);
chessBoard[1][0] = new Pawn(true, 1);
chessBoard[1][1] = new Pawn(false, 1);
chessBoard[1][2] = new Pawn(true, 1);
chessBoard[1][3] = new Pawn(false, 1);
chessBoard[1][4] = new Pawn(true, 1);
chessBoard[1][5] = new Pawn(false, 1);
chessBoard[1][6] = new Pawn(true, 1);
chessBoard[1][7] = new Pawn(false, 1);
chessBoard[2][0] = new ChessPiece(false, 0);
chessBoard[2][1] = new ChessPiece(true, 0);
chessBoard[2][2] = new ChessPiece(false, 0);
chessBoard[2][3] = new ChessPiece(true, 0);
chessBoard[2][4] = new ChessPiece(false, 0);
chessBoard[2][5] = new ChessPiece(true, 0);
chessBoard[2][6] = new ChessPiece(false, 0);
chessBoard[2][7] = new ChessPiece(true, 0);
chessBoard[3][0] = new ChessPiece(true, 0);
chessBoard[3][1] = new ChessPiece(false, 0);
chessBoard[3][2] = new ChessPiece(true, 0);
chessBoard[3][3] = new ChessPiece(false, 0);
chessBoard[3][4] = new ChessPiece(true, 0);
chessBoard[3][5] = new ChessPiece(false, 0);
chessBoard[3][6] = new ChessPiece(true, 0);
chessBoard[3][7] = new ChessPiece(false, 0);
chessBoard[4][0] = new ChessPiece(false, 0);
chessBoard[4][1] = new ChessPiece(true, 0);
chessBoard[4][2] = new ChessPiece(false, 0);
chessBoard[4][3] = new ChessPiece(true, 0);
chessBoard[4][4] = new ChessPiece(false, 0);
chessBoard[4][5] = new ChessPiece(true, 0);
chessBoard[4][6] = new ChessPiece(false, 0);
chessBoard[4][7] = new ChessPiece(true, 0);
chessBoard[5][0] = new ChessPiece(true, 0);
chessBoard[5][1] = new ChessPiece(false, 0);
chessBoard[5][2] = new ChessPiece(true, 0);
chessBoard[5][3] = new ChessPiece(false, 0);
chessBoard[5][4] = new ChessPiece(true, 0);
chessBoard[5][5] = new ChessPiece(false, 0);
chessBoard[5][6] = new ChessPiece(true, 0);
chessBoard[5][7] = new ChessPiece(false, 0);
chessBoard[6][0] = new Pawn(false, 1);
chessBoard[6][1] = new Pawn(true, 1);
chessBoard[6][2] = new Pawn(false, 1);
chessBoard[6][3] = new Pawn(true, 1);
chessBoard[6][4] = new Pawn(false, 1);
chessBoard[6][5] = new Pawn(true, 1);
chessBoard[6][6] = new Pawn(false, 1);
chessBoard[6][7] = new Pawn(true, 1);
chessBoard[7][0] = new Rook(true, 5);
chessBoard[7][1] = new Knight(false, 2);
chessBoard[7][2] = new Bishop(true, 3);
chessBoard[7][3] = new Queen(false, 9);
chessBoard[7][4] = new King(true, 1000);
chessBoard[7][5] = new Bishop(false, 3);
chessBoard[7][6] = new Knight(true, 2);
chessBoard[7][7] = new Rook(false, 5);
for (int i = chessBoard.length-1; i >= 0; i--) {
for (int j = chessBoard.length-1; j >= 0; j--) {
System.out.print(chessBoard[i][j].toString()+"\t");
}
System.out.print(" ");
}
}
}
------------------------- End-------------------------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
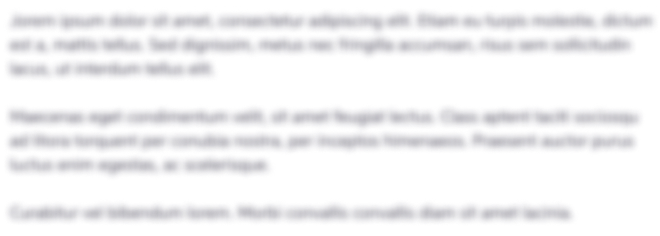
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started