Question
Using JAVA Use data file (fruit.txt) and fruitImages.zip. The fruit.txt file contains name of fruits, and fruitImages.zip contains images of the fruits . You may
Using JAVA
Use data file (fruit.txt) and fruitImages.zip. The fruit.txt file contains name of fruits, and fruitImages.zip contains images of the fruits . You may use ArrayList to save the data into your memory. Your program choose one of the fruits using random method, then display the selected fruit name and its image on a GUI after using Collections.shuffle. User can answer the exact name of the fruit. You are required to use Collections.shuffle for this program. This is a kind of puzzle game. You need to check whether the answer is correct or not. If it is correct, image of the fruit should be displayed. You may add some other functions, such as response time in second, scores, etc. for your extra points.
Your program might produce the following output:
R G E N A O
Orange
[orange image]
G P A R E
Grape
[grape image]
N A A N A B
Banana
[banana image]
Output is displayed in GUI.
Fruit.txt:
B A N A N A A P P L E O R A N G E G R A P E A P R I C O T M A N G O C O C O N U T C H E R R Y R A S P B E R R Y L E M O N
fruitImages.zip is just a zip folder with pictures of the fruit in fruit.txt
This is the source code to start out with:
import java.awt.*; import java.util.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.*;
public class FruitGUI { public static void main(String[] agrs) { new fruitFrame(); } }
class fruitFrame { static final int width = 800, height = 500; private JFrame f; private JPanel p; private JLabel fruitLabel; private JTextField yourAnswerTextField; private JButton StartBT; public String yourAnswer; public String fruitName = ""; public ArrayList
public fruitFrame() { readFruitName(); mainFrame(); }
private void mainFrame() { f = new JFrame("Welcome to Fruit World!"); f.setSize(width, height); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); p = new JPanel(); p.setBackground(Color.GREEN); p.setLayout(null);
fruitLabel = new JLabel(fruitName); fruitLabel.setBounds(width / 5, 30, width - 20, height / 6); fruitLabel.setFont(new Font("Serif", Font.PLAIN, 50));
yourAnswerTextField = new JTextField(50); yourAnswerTextField.setBounds(width / 5, 150, width / 2, height / 6); yourAnswerTextField.setFont(new Font("Serif", Font.PLAIN, 50)); yourAnswerTextField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { yourAnswerTextField.setText(""); fruitLabel.setText(getNextFruit()); } });
StartBT = new JButton("Start"); StartBT.setBounds(width - 200, 50, width / 7, height / 9); StartBT.setFont(new Font("Serif", Font.PLAIN, 30)); StartBT.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent action) { if (action.getSource() == StartBT) { fruitLabel.setText(getNextFruit()); yourAnswerTextField.setText(""); } } });
f.add(p); p.add(fruitLabel); p.add(yourAnswerTextField); p.add(StartBT); f.setVisible(true); }
public void readFruitName() { myFruit.add("APPLE"); myFruit.add("BANANA"); myFruit.add("GRAPE"); myFruit.add("ORANGE"); myFruit.add("MANGO"); myFruit.add("BLUEBERRY"); myFruit.add("KIWI"); myFruit.add("PEACH"); myFruit.add("PAPAYA"); myFruit.add("RASBERRY"); myFruit.add("LEMON"); }
public String getNextFruit() { Random rnd = new Random(); int index; index = rnd.nextInt(myFruit.size()); return myFruit.get(index); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
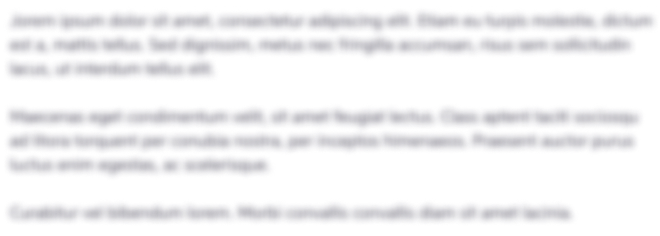
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started