Question
USING JAVA: We will need to do two things here. First create and Emergency Contact class that holds all the information that the company needs.
USING JAVA:
We will need to do two things here. First create and Emergency Contact class that holds all the information that the company needs. Secondly modify the Employee class so that it has an ArrayList for the emergency contacts and methods to allow access. Then check your coding using the EmployeeChecker.java.
Part 1: Emergency Contacts
Create a new java class called EmergencyContact.java. This will hold the name, relationship and phone number of an emergency contact of an employee.
Reference the following UML diagram:
Notes on Methods/Variables for EmergencyContact.java:
-Constructors
We will only have one constructor as we cant set up a blank contact. The constructor takes on 3 string (n name, r relationship, p phone) that should get saved to the instance variables
-Getters and Setters
These should be standard, they return and set values for each corresponding field
-printContact()
This method should print out the information of the contact following this format:
Name: {name}
Relationship: {relationship}
Phone Number: {phone}
Part 2: Modify Employee.java with ArrayLists:
(Employee.java code will be below)
Now that we have the EmergencyContact created we need a ArrayList on our employee to hold those. For this part we are going to add that along with some getter methods to help with our runner/checker files.
Reference the updated Employee UML diagram: (Changes are highlighted)
Notes on Methods/Variables for Employee.java update:
-New Variable:
You will need to import the ArrayList and add a new ArrayList of type EmergencyContact into the employee. NOTE: we are not initializing it, that is done in the constructors.
-Constructors
Now that we have an ArrayList we need to add in each constructor an initializing of it EXCEPT the copy constructor. In that constructor you should use the getEmergencyContactList() method. In the other constructors the ArrayList should be set to the new ArrayList(); code.
-Getters
The method at the bottom of the UML diagram is our getter. It has a return type of the ArrayList and should return the emergencyContact variable.
-printEmergencyContacts()
This method should loop through the ArrayList and print out each contact that is the list. But with a special format. Make sure to have a space between the title and between each contact. (see below)
First at the top BEFORE the loop you should print out:
**** Emergency Contacts ****
Then BEFORE printing each contact (so this would be in the for loop), you should print:
**** Contact # **** Where the # is what contact we are looking at.
So the overall output would be something like:
-clearContacts ()
This method should clear out the ArrayList
-addNewContact(EmergencyContact contact)
This method will receive a new contact and add it into the emergency list.
-removeContact(EmergencyContact contact)
This method will receive a contact to remove from the employees list. Note that this method returns a Boolean data type. It should return true if the contact is in the list and if the removing was successful, otherwise it will return false.
To do this you should use an if statement and the ArrayList .contains() method. Inside you should use the .remove() method to remove from the list and return true. Use the else to return false.
-removeContact(int index)
This another overloaded that method will remove a contact based on an index location along with return true/false if it was done successful.
For this though, we dont want to throw an exception SO you should also have an if statement making sure the size of the emergencyContact lists is >= index && > 0. This would be where you call the remove method to remove the index and return true, otherwise you return false.
Employee.java to modify:
import java.text.NumberFormat; public class Employee { private String firstName; private String lastName; private int employeeNum; private String department; private String jobTitle; private double hoursWorked; private double payRate; //create static variable NumberFormat static NumberFormat currency = NumberFormat.getCurrencyInstance(); //Create Constructors Employee(String firstN, String lastN, int empNum) { hoursWorked = 0.0; firstName = firstN; lastName = lastN; employeeNum = empNum; department = ""; jobTitle = ""; payRate = 0.0; } Employee(Employee emp) { hoursWorked = 0.0; firstName = emp.getFirstName(); lastName = emp.getLastName(); employeeNum = emp.getEmployeeNumber(); department = emp.getDepartment(); jobTitle = emp.getJobTitle(); payRate = emp.getPayRate(); } Employee() { hoursWorked = 0.0; firstName = ""; lastName = ""; employeeNum = 0; department = ""; jobTitle = ""; payRate = 0.0; } //create getters public String getFirstName() { return firstName; } public String getLastName() { return lastName; } public int getEmployeeNumber() { return employeeNum; } public String getDepartment() { return department; } public String getJobTitle() { return jobTitle; } public double getHoursWorked() { return hoursWorked; } public double getPayRate() { return payRate; } //create setters public void setFirstName(String firstN) { firstName = firstN; } public void setLastName(String lastN) { lastName = lastN; } public void setEmployeeNumber(int empNum) { employeeNum = empNum; } public void setDepartment(String depart) { department = depart; } public void setJobTitle(String jbTitle) { jobTitle = jbTitle; } public void setPayRate(double payRt) { payRate = payRt; } //create addHours public void addHours() { hoursWorked += 1; } //create addHours(double h) public void addHours(double h) { if(h > 0) hoursWorked +=h; } //create calculateWeeklyPay() double calculateWeeklyPay(){ return payRate * hoursWorked; } //create resetHours public void resetHours() { hoursWorked = 0; } //printEmployee() void printEmployee() { System.out.println("Name: " + firstName + " " + lastName); System.out.println("ID: " + employeeNum); System.out.println("Department: " + department); System.out.println("Title: " + jobTitle); System.out.println("Pay: " + currency.format(payRate)); System.out.println("Hours Worked: " + hoursWorked); } Employee(String firstN, String lastN, int empNum, String depart, String jbTitle, double payRt) { hoursWorked = 0.0; firstName = firstN; lastName = lastN; employeeNum = empNum; department = depart; jobTitle = jbTitle; payRate = payRt; } }
NEXT: Check the coding using the EmployeeChecker.java:
EmployeeChecker.java:
public class A8EmployeeChecker { public static void main(String[] args) { // setup employees Employee e1 = new Employee("Steve", "Rodgers", 3781, "Sales", "Manager", 28.50); Employee e2 = new Employee("Clint", "Barton", 6847, "Sales", "Customer Representative", 15.34); Employee e3 = new Employee("Tony", "Stark", 5749, "Service", "Lead Service Manager", 32.85); // everyone gets Nick Furry as a contact EmergencyContact nickFurry = new EmergencyContact("Nick Furry", "Boss", "He will contact you"); e1.addNewContact(new EmergencyContact("Peggy Carter", "Friend", "202-896-3633")); e1.addNewContact(new EmergencyContact("Sharon Carter", "Friend", "202-836-9963")); e1.addNewContact(nickFurry); e2.addNewContact(new EmergencyContact("Laura Barton", "Spouse", "319-225-7451")); e2.addNewContact(nickFurry); // nick furry e3.addNewContact(new EmergencyContact("Virginia Potts", "Spouse", "646-746-1025")); e3.addNewContact(new EmergencyContact("Happy Hogan", "Bodyguard", "646-021-4411")); e3.addNewContact(nickFurry); // nick furry System.out.println("***** Assignment 8 Employee Checker ***** "); System.out.println(" ** Steve Rodgers **"); e1.printEmergencyContacts(); System.out.println(" ** Clint Barton **"); e2.printEmergencyContacts(); System.out.println(" ** Tony Stark **"); e3.printEmergencyContacts(); // change tony's wife to nickname Pepper e3.getEmergencyContactList().get(0).setName("Pepper Potts"); System.out.println(" **Updated Tony Stark **"); e3.printEmergencyContacts(); // remove sharon from contact, he went back in time e1.removeContact(1); System.out.println(" **Updated Steve Rodgers **"); e1.printEmergencyContacts(); // also remove nick furry, again went back in time and didn't know Nick (per say ;) ) e1.removeContact(nickFurry); System.out.println(" **Updated (Again) Steve Rodgers **"); e1.printEmergencyContacts(); // makes sure you implemented removeContact correctly here. e1.removeContact(10); e1.removeContact(-2); e1.removeContact(nickFurry); } }
The output should look something like:
>EmergencyContact(String n, String r, String p) +getName (): String +setName(String n): void +getRelationship (): String +setRelationship(String r : void +getPhone (): String +setPhone(String p) : void +printContact (): void Employee.java -firstName : String -lastName : String -employeeNum : int -department : String -jobTitle : String -hoursWorked : double -payRate : double -emergencyContacts : ArrayList -currency: NumberFormat >Employee(String fn, String In, int en, String dept, String job, double pr) > Employee(String fn, String In, int en) > Employee(Employee e) >Employee() +getFirstName() : String +setFirstName(String fn) : void +getLastName() : String +setLastName(String ln) : void +getEmployeeNumber() : int +setEmployeeNumber(int en) : void +getDepartment() : String +setDepartment(String dept) : void +getJobTitle() : String +setJobTitle(String job) : void +getHoursWorked() : double +addHours() : void +addHours(double h) : void +resetHours() : void +getPayRate() : double +setPayRate(double pr) : void +calculateWeeklyPay() : double +printEmployee() : void +printEmergencyContacts() : void +clearContacts : void +addNewContact(EmergencyContact contact) : void +getEmergencyContactList() : ArrayList +removeContact(EmergencyContact contact) : boolean) +removeContact(int index): boolean Emergency Contacts **** Contact 1 **** Name: John Smith Relationship: Friedn Phone: 888-888-8888 *** Contact 2 **** Name: Michelle Epps Relationship: Spouse Phone: 4567891236 **** Assignment 8 Employee Checker ** Steve Rodgers Emergency Contacts Contact 1 Name: Peggy Carter Relationship: Friend Phone Number: 2028963633 **** Contact 2 Name: Sharon Carter Relationship: Friend Phone Number: 2028369963 **** Contact 3 Name: Nick Furry Relationship: Boss Phone Number: He will contact you Phone Number: 3192257451 Rame: Nick Furry Phone Number: He will contact you P** Clint Barton
Step by Step Solution
There are 3 Steps involved in it
Step: 1
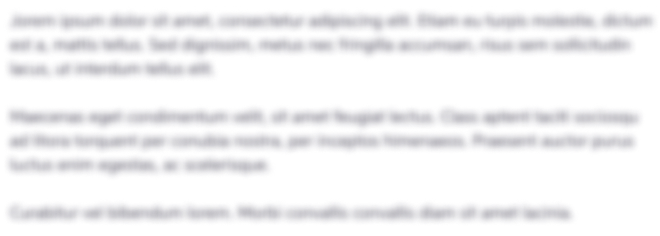
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started