Question
using System; namespace Calculator { class Program { static void Main(string[] args) { double num1, num2, result = 0; char op, save; List savedResults =
using System;
namespace Calculator { class Program { static void Main(string[] args) { double num1, num2, result = 0; char op, save; List
Console.WriteLine("Welcome to the calculator. Enter your equation using the following format: num1 operator num2"); Console.WriteLine("Operators: + for addition, - for subtraction, * for multiplication, / for division, % for remainder"); Console.WriteLine(" ^ for exponentiation, sqrt for square root"); Console.WriteLine("Use the M character to save the last input or result. Use the P character to list saved numbers."); Console.WriteLine("Use the Rn command to retrieve a saved result (e.g. R1 retrieves the first saved result)");
while (true) { Console.Write("Enter your equation: ");
// Get first number validInput = Double.TryParse(Console.ReadLine(), out num1); if (!validInput) { Console.WriteLine("Invalid input. Please enter a valid number."); continue; }
// Get operator op = Console.ReadLine()[0]; if (op != '+' && op != '-' && op != '*' && op != '/' && op != '%' && op != '^' && op.ToString().ToLower() != "sqrt" && op != 'M' && op != 'P' && op != 'R') { Console.WriteLine("Invalid operator. Please enter a valid operator."); continue; } // Get second number validInput = Double.TryParse(Console.ReadLine(), out num2); if (!validInput) { Console.WriteLine("Invalid input. Please enter a valid number."); continue; }
// Perform calculation switch (op) { case '+': result = num1 + num2; break; case '-': result = num1 - num2; break; case '*': result = num1 * num2; break; case '/': result = num1 / num2; break; case '%': result = num1 % num2; break; case '^': result = Math.Pow(num1, num2); break; default: Console.WriteLine("Invalid operator. Please enter a valid operator."); continue; }
Console.WriteLine($"Result: {result}");
// Save result or list saved results if (op == 'M') { savedResults.Add(result); Console.WriteLine("Result saved."); continue; } else if (op == 'P') { Console.WriteLine("Saved Results:"); for (int i = 0; i < savedResults.Count; i++) { Console.WriteLine($"{i + 1}: {savedResults[i]}"); } continue; } else if (op == 'R') { int index; validInput = Int32.TryParse(Console.ReadLine(), out index); if (!validInput || index < 0 || index > savedResults.Count) { Console.WriteLine("Result does not exist at location."); continue; } else { num1 = savedResults[index - 1]; Console.WriteLine($"Retrieved result: {num1}"); op = Console.ReadLine()[0]; } }
// Perform square root or exponentiation function if necessary if (op.ToString().ToLower() == "sqrt") { result = Math.Sqrt(num1); Console.WriteLine($"Result: {result}"); continue; } else if (op == '^') { validInput = Double.TryParse(Console.ReadLine(), out num2); if (!validInput) ; } } } } }
Can you fix this code that after user prints the result in C#
Users now should be able to use the = operator or enter to do calculations.
Program should be able to handle incorrect inputs (like other characters or symbols). If a user enters incorrect input, the program resumes from the last correct input. No exceptions should occur.
Users should be able to use the M character to save the last input or result. Users can save as many numbers as they want. Users can list the saved numbers by using the P character. User can retrieve and continue with any number they want from the list by using Rn where n is any number from the list larger than 0 (e.g. R1 will retrieve the first save element, R2 second, ) Entering invalid number should print: Result does not exist at location. List exists as long as the program runs. Everytime program is restarted the list is empty. Store list in memory.
Please provide screenshot of the output with examples. For example 10 + 20 = 30 and then when user press M it saves the result or when you press P it lists the saved numbers or Rn to retrieve R1 will retrieve the first save element R2 second...
Step by Step Solution
There are 3 Steps involved in it
Step: 1
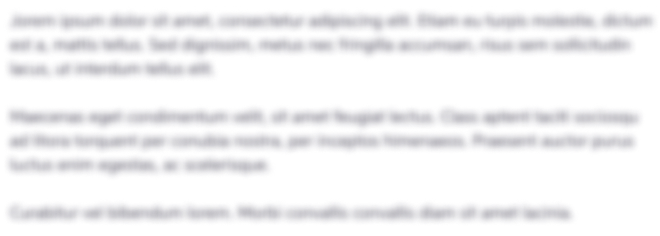
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started