Question
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace DelegatesCompareNames { // this is the delegate declaration. any delegate method implimentation must match
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
namespace DelegatesCompareNames { // this is the delegate declaration. any delegate method implimentation must match this signature public delegate int OurComparer(EmployeeName obj1, EmployeeName obj2); // notice it is sitting outside of all classes, but in the namespace
public class EmployeeName // this is a new class we provide that holds names { // fields private string FirstName = null; private string LastName = null;
public EmployeeName(string first, string last) // constructor { FirstName = first; LastName = last; }
public override string ToString() // overriding the normal ToString so we have an easy way of writing out the values, we'll use later below { return FirstName + " " + LastName; }
// This method provides a delegate method that satisfies the Comparer definition public static int CompareFirstNames(EmployeeName name1, EmployeeName name2) // note it returns an int and takes in 2 objects, so it supports the delegate defintion // this one compares just using the built in String compare, so list will be sorted alphabetically by first name { string n1 = (name1).FirstName; string n2 = (name2).FirstName;
if (String.Compare(n1, n2) 0) { return -1; } else { return 0; } }
//+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ // You need to write a 2nd method // This Method needs to provide a different delegate method // note it must return an int and takes in 2 objects, so it supports the delegate defintion // this one should compare objects based on the length of the first and second name combined // so for example Bob Smith is less than Bobby Smitty but equal to Jim Jones
//+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
//+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ // You need to write a 3rd method that does a compare based on by the number of vowels in both // the first and last name // vowels are a e i o u // you MUST use a helper method to count the vowels // in the helper method, change the passed in theString To.Upper() then you only have 5 values to test for, not 10
//+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
public static int HowManyVowels5(string theString) { // write the required code here. }
}
}
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
namespace DelegatesCompareNames { // This is our big fancy class that does wonderful things for us, // It stores EmployeeNames as objects in an array // It will print all the employees for us // It will sort the list for us, with its "powerful" bubble sort algorithm // (as long as we tell it how to decide what "bigger" means by passing in a delegate method! public class NamesStorage { EmployeeName[] names = new EmployeeName[5]; // array that can hold 5 EmployeeName objects
public NamesStorage() // constuctor nicely loads the array for us { names[0] = new EmployeeName("Joe", "May"); names[1] = new EmployeeName("John", "Hancock"); names[2] = new EmployeeName("Jane", "Doe"); names[3] = new EmployeeName("Jennifer", "Aniston"); names[4] = new EmployeeName("Jacoby", "Ellsbury"); }
// this class provides a Sort method, // BUT - it requires you to pass in a delegate to tell it how to sort public void OurBubbleSort(OurComparer compareMethod) { EmployeeName temp; // to do the sort, we need to flip the order sometimes, so we need a place to put one aside
for (int i = 0; i
public void PrintNames() // simple method to display the array values { foreach (EmployeeName name in names) { Console.WriteLine(name.ToString()); // makes use of our overide of the ToString method } }
} }
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
namespace DelegatesCompareNames { public static class program { // our program!
static void Main(string[] args) { NamesStorage ourEmployees = new NamesStorage(); // call constructor and instantiate ourNames object
// this is the delegate instantiation //OurComparer delegateVarOne = new OurComparer(EmployeeName.CompareFirstNames); // point variable name to one of the compare methods OurComparer delegateVarOne; OurComparer delegateVarTwo; OurComparer delegateVarThree; delegateVarOne = EmployeeName.CompareFirstNames; // point variable name to one of the compare methods delegateVarTwo = EmployeeName.CompareLengthNames; delegateVarThree = EmployeeName.CompareVowels; // notice how I had to qualify the method .compareFirstNames with EmployeeName.CompareFirstNames since // that method is not in this class, it is a static method over in the EmployeeName class.
//+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ //after you write the 2nd and 3rd third new compliant methods, as I did above. // assing delegateVarTwo and delegateVarThree to point to their methods. //+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
Console.WriteLine(" Before Sort: ");
ourEmployees.PrintNames(); // our method, in our object ourEmployees, which with the help of our overriden ToString method, will write out our names.
// here we use our delegate, delegateVarOne, as a delegate argument being passed into our Sort method in OurArrayOfNames // we are giving our Sort method the method we want it to use when it sorts ourEmployees.OurBubbleSort(delegateVarOne);
Console.WriteLine(" After 1st Sort: "); ourEmployees.PrintNames(); // write out the names after the 1st sort
Console.WriteLine();
// now use your 2nd delegate, delegateVarTwo, as a delegate argument // and again call our Sort method in ourEmployees, again passing in the method // call the 2nd sort method here
Console.WriteLine(" After 2nd Sort: "); ourEmployees.PrintNames(); // write out the names after the 2nd sort
Console.WriteLine();
// now use your 3rd delegate, delegateVarThree, as a delegate argument // and again call our Sort method in ourEmployees, again passing in the method // call the 3rd sort method here
Console.WriteLine(" After 3rd Sort: "); ourEmployees.PrintNames(); // write out the names after the 2nd sort
Console.ReadLine(); }
} }
Modify the 3DelCompNames Prob program to add 2nd and 3rd compare methods that satisfy the Comparer delegate signature and enable sorting by 2 new rules. The program already has one working such method to help you get the idea. 1) Write the new compliant 2" method CompareLengthNames. It should return a -1 if the number of letters in the sum of the first and last name of the 1st Name parameter is less than the number of letters in the sum of the first and last name of the 2nd Name parameter It should return a 0 if they are equal It should return a +1 if they are greater 2) Write the new compliant 3method, Compare Vowels. It should return a -1 if the number of vowels in the sum of the first and last name of the 1st Name parameter is less than the number of vowels in the sum of the first and last name of the 2nd Name parameter It should return a 0 if they are equal It should return a +1 if they are greater This method should itself use a new helper method that you write, public static int How Many Vowels(string name) which returns the number of vowels, upper and lower case, in the passed in string 3) After you have these methods defined, modify the code per the green comments in the Program Main class to call the sort method 2 times using these 2 new methods, so that the output look likes: Before Sort: Joe May John Hancock Jane Doe Jennifer Aniston Jacoby Ellebury After 1st Sort: Jacoby Ellsbury Jane Doe Jennifer Aniston John Hancock After 2nd Sort: Toe May Jane Doe John Hancock Jacoby Ellsbury Jennifer Aniston After 3rd Sort: Toe May John Hancock Jane Doe Jacohy Ellsbury Jennifer Aniston Modify the 3DelCompNames Prob program to add 2nd and 3rd compare methods that satisfy the Comparer delegate signature and enable sorting by 2 new rules. The program already has one working such method to help you get the idea. 1) Write the new compliant 2" method CompareLengthNames. It should return a -1 if the number of letters in the sum of the first and last name of the 1st Name parameter is less than the number of letters in the sum of the first and last name of the 2nd Name parameter It should return a 0 if they are equal It should return a +1 if they are greater 2) Write the new compliant 3method, Compare Vowels. It should return a -1 if the number of vowels in the sum of the first and last name of the 1st Name parameter is less than the number of vowels in the sum of the first and last name of the 2nd Name parameter It should return a 0 if they are equal It should return a +1 if they are greater This method should itself use a new helper method that you write, public static int How Many Vowels(string name) which returns the number of vowels, upper and lower case, in the passed in string 3) After you have these methods defined, modify the code per the green comments in the Program Main class to call the sort method 2 times using these 2 new methods, so that the output look likes: Before Sort: Joe May John Hancock Jane Doe Jennifer Aniston Jacoby Ellebury After 1st Sort: Jacoby Ellsbury Jane Doe Jennifer Aniston John Hancock After 2nd Sort: Toe May Jane Doe John Hancock Jacoby Ellsbury Jennifer Aniston After 3rd Sort: Toe May John Hancock Jane Doe Jacohy Ellsbury Jennifer Aniston
Step by Step Solution
There are 3 Steps involved in it
Step: 1
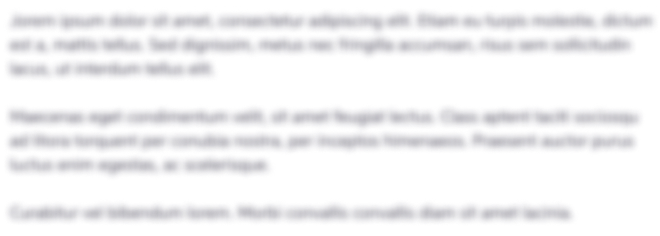
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started