Question
Using the given class definitions C++, create a minimum heap that stores integers and and implements a minimum priority queue. (Your program can be hard
Using the given class definitions C++, create a minimum heap that stores integers and and implements a minimum priority queue. (Your program can be "hard coded" for integers - it does not need to use templates, generics, or polymorphism.)
Your data structure must always store its internal data as a heap.
Your toString function should return a string with the heap values as a comma separated list, also including the size of the heap as well. toString must show the values in the same ordering as they are in the array/heap.
Your heapsort function should create a new array with the sorted values and not modify(*) the heap. That resulting array must be sized to exactly hold the number of elements in the result. Since this is a minimum heap, the heap sort should generate data in descending order. (*): Note that "not modify" means that your data when the function terminates is the same as when you started, not necessarily that it wasn't changed at some time during execution of the function.
Your heapinsert function should return false if you try to insert values into a full heap (and return true on a sucessful insert.) minimum and extractMinimum should return 0 on an empty heap.
You are required to implement and use parent(i), left(i), and right(i) helper functions. I also recommend use of a "minimum of three" helper function as dscribed in class.
Don't forget a bounds checks as needed. (In general, make sure that all of your public functions are robust!)
For this assingment, please create a zero-based heap (where A[0] is the root) as described in class, and not a one-based heap (where A[1] is the root) as described in the textbook.
You may make minor adjustments to the class definitions (for example, adding extra helper functions or overloading the constructor to accept initial values,) but any major changes must have prior approval in writing (do this via email.)
// integer minimum heap with PQ class intMinHeap{ public: intMinHeap(int); // empty heap wth this capacity ~intMinHeap(); // clean up allocated memory int *heapsort(); // return sorted array from heap string toString(); bool heapinsert(int); // add element to heap; return success // min functions should return 0 in empty heaps int minimum(); // return value of A[root] int extractmin(); // return and remove A[root] void decreasekey(int i, int k); // A[i] decreased to k bool isEmpty(){return size==0;} bool isFull(); private: int minOf3(int, int, int); // with bounds check! int left(int); int right(int); int parent(int); void buildheap(); // convert array to a heap void heapify(int i); // heapify at position i int *A; // array of integers - data int capacity; // size of array A int size; // data size in array };
class intMinHeap implements Serializable{ public intMinHeap(int cap){ ... } public int [] heapsort(){ ... } public String toString(){ ... } public bool heapinsert(int n){ ... } public int minimum(){ ... } // A[root] or 0 if empty public int extractMin(){ ... } // A[root] or 0 public void decreaseKey(int i, int k){ ... } public boolean isEmpty(){ ... } public boolean isFull(){ ... } private void buildheap(){ ... } private int minOf3(int i, int j, int k){ ... } private int left(int i){ ... } private int right(int i){ ... } private int parent(int i){ ... } private void heapify(int i){ ... } // at position i private int [] A; private int capacity; // size of array A private int size; // size of data in array A }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
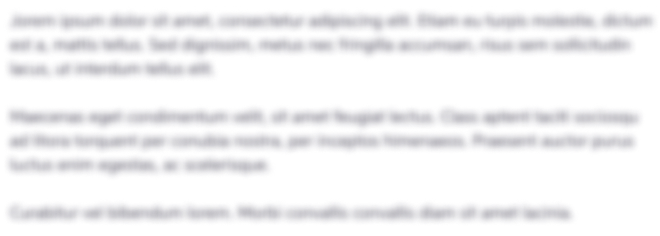
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started