Question
Using the UML Class Diagram write a prototype for the system with the following specifications: 1. Building class: this is the superclass for all objects
Using the UML Class Diagram write a prototype for the system with the following specifications:
1. Building class: this is the superclass for all objects in our system. It contains the following instance members/constructors/methods:
a. projectName the name of the building project e.g. Smith House.
b. completeAddress the complete address of the project e.g. 123 Main Street, Middletown, New York 17077.
c. totalSquareFeet the square footage of the building (e.g. 2,450 square feet)
d. occupancyGroup group code according to Table 1.
e. subgroup subgroup code according to Table 1. f. Constructors there are two constructors. An empty-argument constructor that should set all instance fields to or 0.0 as appropriate. The preferred constructor should support passing parameters for each instance field.
g. draw method eventually this will be used to draw the object to the screen. For the prototype, it will simply print to the console:
Drawing code for >. All of the draw methods in the subclasses will do the same.
h. displayData method will return a formatted String that contains all of the information about the object.
2. Business class: this subclass of Building has the following instance members/constructors/methods:
a. numRentableUnits the number of units in the building that can be rented.
b. Constructors there are two constructors. An empty-argument constructor that should set all instance fields as appropriate for the data type. The preferred constructor should support passing parameters for each instance field.
c. draw method eventually this will be used to draw the object to the screen. For the prototype, it will simply print to the console: Drawing code for >. All of the draw methods in the subclasses will do the same.
d. displayData method will return a formatted String that contains all of the information about the object.
3. Mall class: this subclass of Business has the following instance members/constructors/methods:
a. numRentedUnits the number of units in the building that are currently being rented.
b. medianUnitSize the mall contains rentable units of different size. This instance member represents the median of all of the unit sizes.
c. numParkingSpaces the total number of parking spaces around the mall.
d. Constructors there are two constructors. An empty-argument constructor that should set all instance fields as appropriate for the data type. The preferred constructor should support passing parameters for each instance field.
e. draw method eventually this will be used to draw the object to the screen. For the prototype, it will simply print to the console: Drawing code for >. All of the draw methods in the subclasses will do the same.
f. displayData method will return a formatted String that contains all of the information about the object.
4. Residential class: this subclass of Building represents a building where people can live (has bedrooms and bathrooms) and has the following instance members/constructors/methods:
a. numBedrooms the number of bedrooms in the building.
b. numBathrooms the number of bathrooms in the building.
c. laundryRoom whether or not the building has a laundry room.
d. Constructors there are two constructors. An empty-argument constructor that should set all instance fields as appropriate for the data type. The preferred constructor should support passing parameters for each instance field.
e. draw method eventually this will be used to draw the object to the screen. For the prototype, it will simply print to the console: Drawing code for >. All of the draw methods in the subclasses will do the same.
f. displayData method will return a formatted String that contains all of the information about the object.
5. Apartment class: this subclass of Residential represents a building where more than one family can live and has the following instance members/constructors/methods:
a. numRentableUnits the number of units that can be rented in the building.
b. avgUnitSize the average size of the apartments (square footage) in the building.
c. parkingAvailable a boolean value that indicates if onsite parking is available for this building.
d. Constructors there are two constructors. An empty-argument constructor that should set all instance fields as appropriate for the data type. The preferred constructor should support passing parameters for each instance field.
e. draw method eventually this will be used to draw the object to the screen. For the prototype, it will simply print to the console: Drawing code for >. All of the draw methods in the subclasses will do the same.
f. displayData method will return a formatted String that contains all of the information about the object.
6. SingleFamilyHome class: this subclass of Residential represents a building where a one family lives (has a limit on the number of people who can live there). This class has the following instance members/constructors/methods:
a. garage a boolean value that indicates whether or not the house has a garage.
b. Constructors there are two constructors. An empty-argument constructor that should set all instance fields as appropriate for the data type. The preferred constructor should support passing parameters for each instance field.
c. draw method eventually this will be used to draw the object to the screen. For the prototype, it will simply print to the console: Drawing code for >. All of the draw methods in the subclasses will do the same.
d. displayData method will return a formatted String that contains all of the information about the object.
7. Application class: After you have completed your inheritance hierarchy, write an Application class (not shown in the UML Class Diagram) to test every object. In your test, create an instance of every object and then write code to test every constructor/method/getter/setter/toString for the object. Start with the most generic object and move to the more specific object
Building #projectName: String #completeAddress: String #totalSquare Feet: double #occupancyGroup: String #subgroup: String +Building() +Building(String projectName, String complete Address, double totalSquareFeet, String occupancyGroup, String subgroup) +draw(): void +displayData(): String Residential Business #num RentableUnits: int *Business() +Business(String projectName, String completeAddress, double totalSquareFeet, String occupancyGroup, String subgroup) #numBedrooms:int #num Bathrooms: int #laundry Room: boolean +Residentiall) +Residential(String projectName, String complete Address, double totalSquare Feet, String occupancyGroup, String subgroup) Mall Apartment SingleFamily Home -numRentedUnits -numRentableUnits: int -medianUnitSize: double -garage: boolean -avgUnitSize: double -numParking Spaces: int - parking Available: boolean +Single Family Homel) +Mallo) +Apartment() +Single Family Home(String projectName, +Mall(String projectName, String complete Address, String complete Address, double +Apartment(String projectName, String completeAddress, double totalSquareFeet, String occupancyGroup, totalSquare Feet, String double totalSquare Feet, String occupancyGroup, String occupancyGroup, String subgroup) String subgroup) subgroup) +draw(): void +draw(): void +draw(): void +displayData(): String +displayData(): String +displayData(): String Figure 1: UML Class Diagram for Construction Program Building #projectName: String #completeAddress: String #totalSquare Feet: double #occupancyGroup: String #subgroup: String +Building() +Building(String projectName, String complete Address, double totalSquareFeet, String occupancyGroup, String subgroup) +draw(): void +displayData(): String Residential Business #num RentableUnits: int *Business() +Business(String projectName, String completeAddress, double totalSquareFeet, String occupancyGroup, String subgroup) #numBedrooms:int #num Bathrooms: int #laundry Room: boolean +Residentiall) +Residential(String projectName, String complete Address, double totalSquare Feet, String occupancyGroup, String subgroup) Mall Apartment SingleFamily Home -numRentedUnits -numRentableUnits: int -medianUnitSize: double -garage: boolean -avgUnitSize: double -numParking Spaces: int - parking Available: boolean +Single Family Homel) +Mallo) +Apartment() +Single Family Home(String projectName, +Mall(String projectName, String complete Address, String complete Address, double +Apartment(String projectName, String completeAddress, double totalSquareFeet, String occupancyGroup, totalSquare Feet, String double totalSquare Feet, String occupancyGroup, String occupancyGroup, String subgroup) String subgroup) subgroup) +draw(): void +draw(): void +draw(): void +displayData(): String +displayData(): String +displayData(): String Figure 1: UML Class Diagram for Construction ProgramStep by Step Solution
There are 3 Steps involved in it
Step: 1
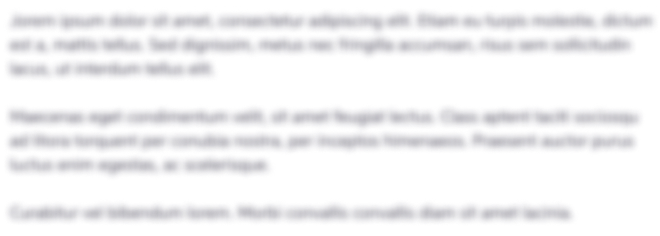
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started