Question
Utilize a ThreadPoolExecutor to load all of the people records in the database. You are going to implement most of this section on your own,
Utilize a ThreadPoolExecutor to load all of the people records in the database. You are going to implement most of this section on your own, but here are a few requirements that should assist your thinking process:
First, create a helper method (outside the PersonDB class) that will act as an intermediary to PersonDBs load_person method:
def load_person(id, db_file): with PersonDB(db_file) as db: return db.load_person(id)
You can use this to simplify the future target for the threads.
-
You are to use exactly 10 worker threads (the argument to ThreadPoolExecutor)
-
You must create the ThreadPoolExecutor with a context manager with block. The future target is the load_person() function defined above
-
You can assume that the record ids to load are 0 through max_people 1. Yes, that means you will spawn max_people futures...
-
Try to launch all of the future workers using a comprehension that returns a list of future objects so you can use wait() or as_completed() to sync back up on the main thread
-
Put all the resulting records in a list and print it out (will be a big list)
Once all records are loaded in a list [], sort the list by last name and first name (multiple sort keys).
-
Print that list. Hint: Review the lambdas demo
This is my CODE
import random
from concurrent.futures import ThreadPoolExecutor
import sqlite3 from sqlite3 import Error
people_db_file = "sqlite.db" # The name of the database file to use max_people = 500 # Number of records to create
last_names = [] first_names = []
def create_people_database(db_file, count): conn = sqlite3.connect(db_file) with conn: sql_create_people_table = """ CREATE TABLE IF NOT EXISTS people ( id integer PRIMARY KEY, first_name text NOT NULL, last_name text NOT NULL); """ cursor = conn.cursor() cursor.execute(sql_create_people_table)
sql_truncate_people = "DELETE FROM people;" cursor.execute(sql_truncate_people)
people = generate_people(count) sql_insert_person = "INSERT INTO people(id,first_name,last_name) VALUES(?,?,?);" for person in people: #print(person) # uncomment if you want to see the person object cursor.execute(sql_insert_person, person) #print(cursor.lastrowid) # uncomment if you want to see the row id cursor.close()
class PersonDB(): def __init__(self, db_file=''): self.db_file = db_file
def __enter__(self): self.conn = sqlite3.connect(self.db_file) return self def __exit__(self, exc_type, exc_value, exc_traceback): self.conn.close() def load_person(self, id): sql = "SELECT * FROM people WHERE id=?" cursor = self.conn.cursor() cursor.execute(sql, (id,)) records = cursor.fetchall() result = (-1,'','') # id = -1, first_name = '', last_name = '' if records is not None and len(records) > 0: result = records[0] cursor.close() return result
def test_PersonDB(): with PersonDB(people_db_file) as db: print(db.load_person(10000)) # Should print the default print(db.load_person(122)) print(db.load_person(300))
def read_name(filename): data = list() with open(filename, 'r') as filehandle: dataname = [line.rstrip() for line in filehandle] return dataname
def generate_people(count): with ThreadPoolExecutor(max_workers = 2) as executor: futureA = executor.submit(read_name, 'LastNames.txt') futureB = executor.submit(read_name, 'FirstNames.txt') last_names = futureA.result() first_names = futureB.result()
names = list()
for i in range(count): names.append((i, first_names[random.randint(0, len(first_names)-1)], last_names[random.randint(0, len(last_names)-1)])) return names
if __name__ == "__main__": create_people_database(people_db_file, max_people) PersonDB.test_PersonDB() #people = generate_people(5) #print(people)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
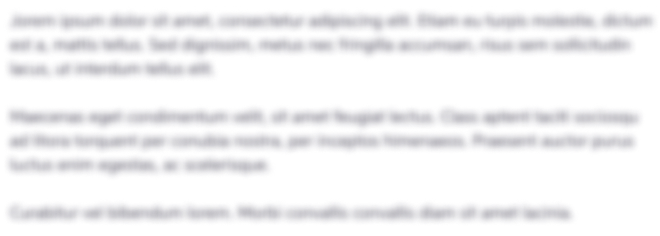
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started