Question
Vehicle regisstration Java program. Task is to write a program to registers information about vehicles, and should be buildt according to: Write an abstract class
Vehicle regisstration Java program. Task is to write a program to registers information about vehicles, and should be buildt according to:
Write an abstract class Vehicle with the following properties:
- private String colour, name, serialNumber.
- private int model (rs modell), price, direction.
- private double speed.
- protected java.util.Scanner input.
- An empty constructor.
- A constructor to initialize all variables ( except for speed, (should be 0)).
- public void setAllFields() method which uses user-input to read in all values from user and put them in object(except from direction and speed,which shoul be 0))
- public abstract void turnLeft(int degrees).
- public abstract void turnRight(int degrees). Both abstract methods should implemented by subclasses.
- get and set methods for all members.
- public String toString() method to print information on all members of the class.
Car inherits from Vehicle
- private int power (hp).
- private Calendar productionDate.
- An empty constructor.
- A constructor to initialize all variables ( except for speed, (should be 0)).
- Override av setAllFields method to also initialize members in Car class.
- turnRight(int degrees) method should first check if 0
- turnLeft(int degrees) should do the same, in opposite direction.
- get and set methods for all members
- Override toString() method to print additional relevant information from Car class.
Bicycle inherits from Vehicle.
- private int gears.
- private Calendar productionDate.
- An empty constructor.
- A constructoor to initialize all variables( except fro speed(should be 0)).
- Override of setAllFields method to also inititialize members of Bicicle class.
- turnRight(int degrees) method should print the amount of degrees turned( no check needed)
- turnLeft(int degrees) should do the same, in opposite direction.
- get and set methods for all members.
- Override toString() method to print additional relevant info from Bicycle class.
TestVehicles should implement all functions marked as TODO.
The TestVehicles code is partly written and is found below :
import java.util.*; /** * Class that tests functionality of Vehicle, Bicycle and Car classes. */ public class TestVehicles { ArrayListVehicle> vehicles = new ArrayListVehicle>(); public static void main(String[] args) { TestVehicles vtest = new TestVehicles(); try { vtest.menuLoop(); } catch (InputMismatchException e) { System.out.println("InputMismatchException!"); System.out.println(e.getMessage()); System.exit(1); } } private void menuLoop() throws InputMismatchException { Scanner input = new Scanner(System.in); Vehicle vehicle; vehicles.add(new Car("Volvo 740", "blue", 85000, 1985, "1010-11", 0, 120)); vehicles.add(new Car("Ferrari Testarossa", "red", 1200000, 1996, "A112", 0, 350)); vehicles.add(new Bicycle("Monark 1", "yellow", 4000, 1993, "BC100", 0, 10)); vehicles.add(new Bicycle("DBS 2", "pink", 5000, 1994, "42", 0, 10)); while (true) { System.out.println("1...................................New car"); System.out.println("2...............................New bicycle"); System.out.println("3......................Find vehicle by name"); System.out.println("4..............Show data about all vehicles"); System.out.println("5.......Change direction of a given vehicle"); System.out.println("6..............................Exit program"); System.out.print(".............................Your choice? "); int choice = input.nextInt(); switch (choice) { case 1: System.out.println("Input car data:"); vehicle = new Car(); vehicle.setAllFields(); // TODO Override method vehicles.add(vehicle); break; case 2: System.out.println("Input bicycle data:"); vehicle = new Bicycle(); vehicle.setAllFields(); // TODO Override method vehicles.add(vehicle); break; case 3: // TODO ask for vehicle name and print out vehicle with that name break; case 4: // TODO list all vehicles break; case 5: // TODO ask for vehicle name and change its direction break; case 6: input.close(); System.exit(0); default: System.out.println("Invalid option!"); } } } }
--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Here is an example of the program:
-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
And here is the UML-diagram for the classes:
4. . . . . . . .. .. . . . Show data about all vehicles Input car data: Nam Test Colour: Test Price: 123456 Model: 1234 Serial #: 12-345 Power: 123 4. . . . . . . .. .. . . . Show data about all vehicles Name of vehicle: test Name: Test Colour: Test Price: 123456 Model: 1234 Serial# : 12-345 Direction : 0 Speed: 0.00 Power : 123 Production date: 2018-01-29 1... .New car 3 4. Find vehicle by name . . .. . . . ..Show data about all vehicles 6. .Exit progn . . Name : Volvo 740 Colour: blue Price: 85000 Model : 1985 Serial# : 1010-11 Direction : 0 Speed: 0.00 Power: 120 Production date: 2018-01-29 Name: Testarossa Colour: red Price: 1200000 Model: 1996 Serial# : A112 Direction: 0 Speed: 0.00 Power : 350 Production date: 2018-01-29 Name: Monark 1 Colour: yellow Price: 4000 Model: 1993 Serial#: BC100 Direction : 0 Speed : 0.00 Gears: 10 Production date: 2018-01-29 Name: DBS 2 Colour: pink Price: 5000 Model : 1994 Serial#: 42 Direction : 0 Speed: 0.00 Gears: 10 Production date: 2018-01-29 Name: Test Colour: Test Price: 123456 Model: 1234 Serial# : 12-345 Direction : 0 Speed: 0.00 Power : 123 Production date: 2018-01-29
Step by Step Solution
There are 3 Steps involved in it
Step: 1
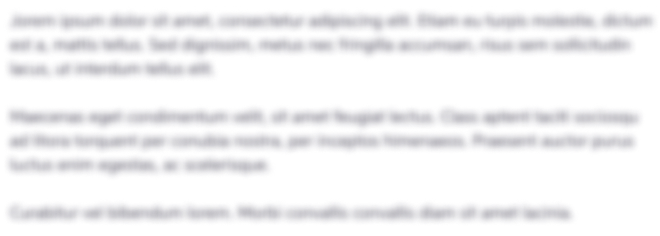
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started