Question
Very Basic Java Snake Game. Nothin elaborate. Just looking for some suggestions/assistance. Thank you. import java.util.Scanner; public class SnakeGame { // SnakeGame is a text
Very Basic Java Snake Game. Nothin elaborate. Just looking for some suggestions/assistance. Thank you.
import java.util.Scanner;
public class SnakeGame { // SnakeGame is a text based snake game. // The snake moves around the board until it runs into itself or goes out of bounds. // The edge of the board is drawn with #s // The snake is drawn with Ss. // The snake has 20 spaces horizontally and 10 spaces vertically where it can move. // The edge of the board is outside the 20 x 10 area // Initially the snake is 2 segments long at (1, 1) and (1, 2). // Let the positions be 0-based. (0, 0) is the upper left. // X grows positive to the right and y grows positive going down. // The initial board, with the 2 initial snake segments, looks like: // If the snake moves to a spot already occupied by the snake or into the edge of the board, then the game is over. // Keep track of the number of moves before the game ends. // Contains main() essentially the code shown above in how to play the game public static void main(String [] args) { Scanner scan = new Scanner(System.in); char move; boolean isRunning = true; // How to play the game (this is basically main()): // Initialize the board and snake // Initialize the number of moves made // Print the board // Continue until the game ends (snake runs into edge or itself) while(isRunning = true) { // Remove the oldest segment from the snake // For example, the first time, (1, 1) will be removed from the snake // Ask the user for a move (l for left, r for right, u for up, and d for down) System.out.print("Move (l/r/u/d)?"); move = scan.next().charAt(0); // Move the snake twice in that direction // For example, if the user chooses r and the last segment added to the snake was (1, 2), // then add (2, 2) and (3, 2) to the snake // Right increases the x-coordinate. So (1, 2) moves to (2, 2) and (2, 2) moves to (3, 2) // I had a method in my SnakeBoard class that did a single move and called it twice. // I felt this option would be more flexible if I wanted to change the game in the future. // Print the board // Increment the number of moves made } // After the game is over, print the number of moves made } }
---------------------------------------------------------------------------------------------------------------------------------------------------------
// SnakeBoard class
public class SnakeBoard { // Need some final instance variables // Width of the board // Height of the board private final static int BoardWidth = 20; private final static int BoardHeight = 10; // 2 instance variables // Array or ArrayList of Position objects will contain all of the Positions of the Snake // I used ArrayList, but you may use a regular array if you prefer // Flag to remember if snake has made an illegal move (moved onto itself or off the edge of the board) // This instance variable is optional as it depends on how you design the solution // If you use it, the game is over when this flag becomes true // Constructor // Initialize the Array/ArrayList // Add first 2 snake segments to the Array/ArrayList // You should create a new Position object, set its x, y, add it to your Array/ArrayList // Then you should create a second new Position, set its x, y, and add it to your Array/ArrayList // Do NOT try to reuse the same Position object youll want to call new a second time // print method to print the board // This method will need nested loops to print the two-dimensional board // Within the loops, I checked to see if the x,y location that I was about to print // was a Position in my Array/ArrayList. If it was in there, then I printed S, // otherwise I printed (space). // This is where I used the snakeHere method listed below // removeSnake method to remove the oldest Position in the Array/ArrayList // moveSnake method to add one more Position to the Array/ArrayList // pass in a direction to move // use the last Position of the Snake and the direction to calculate the x, y of the new Position // add the new Position to your Array/ArrayList if it is a valid move // This is where I used the validMove method listed below // gameOver method that returns true if the game is over, false otherwise // validMove method that returns true if moving to x, y is okay, false otherwise // I also used the snakeHere method to help decide if this is a valid move or not // snakeHere method that returns true if the x,y you are checking is an x,y // currently in your Array/ArrayList }
---------------------------------------------------------------------------------------------------------------------------------------------------------------
// Position class
public class Position
{
// Simple class that contains x, y and matching get/set methods
// The following is an example of a Position class feel free to use it,
// but please add some comments!
private int x;
private int y;
public int getX()
{
return x;
}
public void setX(int x)
{
this.x = x;
}
public int getY()
{
return y;
}
public void setY(int y)
{
this.y = y;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
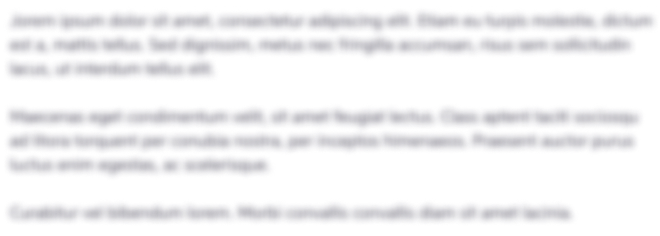
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started