Question
We all love the std::string class. Now lets make our own string class. The goal today is to create a simple str class that mimic
We all love the std::string class. Now lets make our own string class. The goal today is to create a simple str class that mimic the functionality of the std::string class. To keep things civilized, I have taken the liberty of implementing part of the str class. You are asked to complete the rest of the functions.
Task
Please implement your functions in the str.cpp class.
Header file declaring str class.
// str.h
#ifndef __str_h__ #define __str_h__
#include
using namespace std;
class str {
protected: char* _buf; // pointer to the underlying storage int _n; // size of the buffer
public:
// constructors of various forms
str(); str(int n); str(char ch); str(const char* c_str);
// lets not forget the destructor
~str();
// inline functions for finding length info of the str
inline const int& length() const { return _n; } inline bool is_empty() const { return length() == 0; }
// index operators
char& operator[](int i) { return _buf[i]; } const char& operator[](int i) const { return _buf[i]; }
// TODO 1. You need to implement the assignment operator
const str& operator=(const str& s); // TODO 2. You need to implement the + operator concatenates two str
friend str operator+(const str& a, const str& b);
friend ostream& operator<<(ostream& os, const str& s);
friend istream& operator>>(istream& is, str& s); };
#endif
Meanwhile the str.cpp file looks like // str.cpp #include "str.h" str::str() : _n(0), _buf(0)
{}
str::str(int n) : _n(n) {
_buf = new char[_n]; }
str::str(char ch) : _n(1) {
_buf = new char[_n];
_buf[0] = ch; }
str::str(const char* c_str) {
_n = strlen(c_str); _buf = new char[_n]; for (int i=0; i<_n; ++i) _buf[i]=c_str[i];
}
str::~str() {
if (_buf) delete [] _buf; }
ostream& operator<<(ostream& os, const str& s) {
if (!s.is_empty()) { for (int i=0; i cout << s[i]; }} else cout << "[null str]";return os; }
istream& operator>>(istream& is, str& s) {
char ch; do {
ch = is.get(); if (ch == ' ' || ch == ' ') break; s = s + ch;} while(true); return is;
}
// 1. TODO - assignment operator // 2. TODO - concatenation operatorAnd the main.cpp is // main.cpp #include "str.h"
int main() {
str s1; cout << s1 << endl;str s2("Hello"); cout << s2 << endl;str s3("World"); str s4 = s2 + " " + s3;cout << s4 << endl;str s5, s6; cin >> s5 >> s6; cout << s5 << ' ' << s6;return 0; }
When I compile and run the code (g++ main.cpp str.cpp -o str) I get the following output
$ ./str <---- user [null str] Hello Hello World123 345 <---- user 123 345
Step by Step Solution
There are 3 Steps involved in it
Step: 1
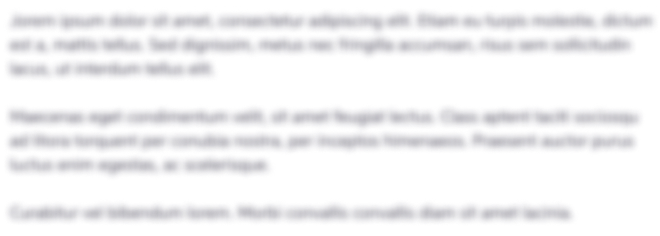
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started