Question
We have a two player tennis game right now and the following code animates the motion of tennis ball. When it hits the net or
We have a two player tennis game right now and the following code animates the motion of tennis ball. When it hits the net or bounces twice on a same side the game restarts. It also sets up the velocity and initial position of the ball.
importturtle,math,random
classBall(turtle.Turtle):
def__init__(self,px,py,vx,vy):
turtle.Turtle.__init__(self)
self.vx=vx
self.vy=vy
self.shape("circle")
self.turtlesize(0.3)
self.penup()
self.setpos(px,py)
self.bounce=0
defmove(self):
self.vy=self.vy-0.981
new_px=self.xcor()+self.vx
new_py=self.ycor()+self.vy
ifnew_py<=0:
new_py=self.ycor()*0.75
self.vy=-0.75*self.vy
#incrementbouncecounter
self.bounce+=1
#showbouncelocation
ifnew_py>0:
print('bounceright')
elifnew_py<0:
print('bounceleft')
#ifballcrossedcenterline,resetbouncecounter
if(self.xcor()<0and0
self.bounce=0
#ifdoublebounce
ifself.bounce>=2:
print('pointover')
#resetbouncecounter
self.bounce=0
#resetballposition
self.reset()
else:
self.setpos(new_px,new_py)
defhit(self):
print("spacebarpressed")
self.vx=-self.vx
self.vy=15
defreset(self):
"""resettheballintoanewrandomlocation"""
print('ballreset')
#setrandomxandyvelocity
self.vx=random.randint(-12,6)
self.vy=random.randint(4,10)
#setrandomxandyposition
px=random.randint(-100,100)
py=random.randint(30,100)
self.setpos(px,py)
classGame:
def__init__(self):
turtle.delay(0)
turtle.tracer(0,0)
turtle.setworldcoordinates(-500,-500,500,500)
#drawcourtandnet
#initializenetheight
self.net_height=30
#createnewturtleobject
t=turtle.Turtle()
t.penup()
#movetoleftsideofcourt
t.setpos(-400,0)
t.pendown()
#gotoeastsideofcourt
t.goto(400,0)
t.penup()
#gotomiddleofcourt
t.setpos(0,0)
#drawnet
t.left(90)
t.pendown()
t.forward(self.net_height)
#hidetheturtle,butgivecredittohishardwork
t.hideturtle()
#initializeside
self.side=None
self.ball=Ball(
random.randint(-100,100),
random.randint(30,100),
random.randint(-12,6),
random.randint(4,10),
)
turtle.update()
self.gameloop()
turtle.onkeypress(self.ball.hit,"space")
turtle.listen()
turtle.mainloop()
defgameloop(self):
#getxandypositionofball
x=self.ball.xcor()
y=self.ball.ycor()
#ifnetishit
ifx==0andy<=self.net_height:
print('nethit')
self.side=None
self.ball.reset()
#ifballisinleftofnet
elifx<0:
#ifcomingfromrightofnet
ifself.side=='right':
##ifheightislowerthannetheightduringcrossingwindow
ify<=self.net_height:
print('nethitfromright')
self.side=None
self.ball.reset()
else:
print('passedovernetgoingleft')
#setsidetoleft
self.side='left'
#ifballisinrightofnet
elif0 #ifcomingfromleftofnet ifself.side=='left': #ifheightislowerthannetheightduringcrossingwindow ify<=self.net_height: print('nethitfromleft') self.side=None self.ball.reset() else: print('passedovernetgoingright') #setsidetoright self.side='right' self.ball.move() turtle.update() turtle.ontimer(self.gameloop,30) if__name__=="__main__": Game() Now we do the following things: Add more player control to the game by allowing the player to change the angle of their shot. This reflects the original tennis for two, where each player's controller was a knob to represent the angle of the shot, and a button. The angle of a player's paddle should control how much force is applied in the x and y directions. Add a visual aid for each player that shows the direction that their paddle will hit the ball. This can be accomplished by creating a turtle for each player and having it point in the direction that their paddle will send the ball when they hit it. Change your code so that it is a two-player game, with a different key for hitting each direction. This sounds like a simple change but keep in mind that you should not allow one player to hit the ball twice in a row. This is illegal and the point is over and should be reset. Alternatively, have one of the players be a computer player. Use some randomness to determine how hard and at what angle it hits the ball. (If you pick both this and option 3 you need some way to switch between two player mode and one player vs. one computer).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
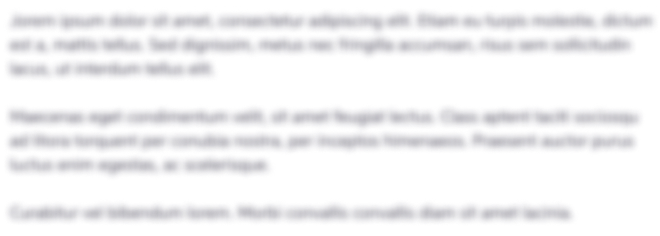
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started