Question
We have already worked with if statements to detect erroneous data. But in some cases users will repeatedly enter the wrong value. In addition, some
We have already worked with if statements to detect erroneous data. But in some cases users will repeatedly enter the wrong value. In addition, some problems lend themselves to repeatedly executing the program. This lab will explore these uses of the Java loop.
You will begin with my solution to the EightBall lab. The 2 programs used there were:
EightBall.java (provided below) EightBallShaker.java (provided below)
Follow the steps below to handle error checking and to allow the user to play as long as he or she wants.
Error checking - part 1
In the original program, we asked the user for a question and then shook the 8 ball to get an answer. In this variation, you will be checking that question to make sure that it conforms to the requirements below.
Your question should not be an empty String. (Hint: The String class has a length() method that will tell you how many characters are in the String.) Prompt the user to enter the question, read in the question and then create a loop that will test for the empty String. If the String is empty, you should tell the user that the empty String is not allowed and then prompt for and read in the question again. You should do this until the user enters a valid String.
After you have read in the proper question, echo the question with a suitable label. For example: "You entered: What is my name?" Then shake the 8 ball, and return the answer.
Error checking - part 2
Once part 1 is working correctly, add in a check for a String longer than 60 characters. If the String is longer than 60 characters, print out an error message that indicates that the String is too long. NOTE: This will not be a new loop, but will be a part of the original loop. Re-prompt the user and read in the question again. Continue checking for the length until the user enters a question longer than the empty String and less or equal to 60 characters.
Note: your error message should be specific. If the problem is an empty String, report that the String is empty -- if the problem is that the String is too long, report that the String is too long.
Once your program has passed these two errors, echo the question with a suitable label, shake the 8 ball and return the answer.
Error checking - part 3
Once part 2 is working correctly, add in another test to make sure that the String is in the form of a question. If the String does not end with the question mark symbol, '?', you should print an appropriate error message and re-prompt the user and read in the question again. NOTE: This will not be a new loop, but will be part of the original loop. Continue to check the length and check for a question mark before continuing.
Once it has passed the three validations, echo the question with a suitable label, shake the 8 ball and return the answer.
Continue to play - part 4
Once part 3 is working correctly, we want to add a loop surrounding the original code that will execute the loop until the user says that he or she no longer wishes to continue. Before the loop begins, ask the user: "Do you want to play Magic 8 Ball? " and get their response. Using a while loop around the rest of your program, test that the user enters yes, Yes, or YES. If they enter one of these values, continue with the code you set up in part 1. If they answer anything else, exit the program with a goodbye message.
Once the user has asked a question of the EightBall and received an answer, you should prompt the user to see if he or she wants to play again with something like "Ask another question?" This should be at the end of the loop. Control will then return to the top of the loop, where you will test for the proper values (this part you've already written).
EightBall.java
public class EightBall {
// declarations public static final int MAX = 10; // you can change this if you // want
// use these finals for your Magic8Ball answers public static final String ANSWER01 = "You're kidding, right?"; public static final String ANSWER02 = "Read my lips..."; public static final String ANSWER03 = "Sure, why not?"; public static final String ANSWER04 = "The answer is within you."; public static final String ANSWER05 = "Get Real!"; public static final String ANSWER06 = "But of course!"; public static final String ANSWER07 = "What have you been doing?"; public static final String ANSWER08 = "New to Earth, are you?"; public static final String ANSWER09 = "That's for me to know and you " + "to find out"; public static final String ANSWER10 = "Why are you asking me?"; public static final String ERROR_MSG = "Huh?";
private Random generator;
/** * Constructor - initialize and instantiate the instance variables. */ public EightBall() {
generator = new Random();
} // constructor
/** * Generate a random number between 1 and MAX and return the phrase * corresponding to it using a switch/case structure. Use the String finals * above, with the ERR_MSG final as the default case. * * @return a random string */ public String shakeEightBall01() {
int randomNum; String randomString;
randomNum = generator.nextInt( MAX );
switch ( randomNum ) { case 0: randomString = ANSWER01; break; case 1: randomString = ANSWER02; break; case 2: randomString = ANSWER03; break; case 3: randomString = ANSWER04; break; case 4: randomString = ANSWER05; break; case 5: randomString = ANSWER06; break; case 6: randomString = ANSWER07; break; case 7: randomString = ANSWER08; break; case 8: randomString = ANSWER09; break; case 9: randomString = ANSWER10; break; default: randomString = ERROR_MSG;
} // end switch
return randomString;
} // method shakeEightBall01
} // class EightBall
EightBallShaker.java
public class EightBallShaker { /** * Main method. * * @param args Unused command line arguments */ public static void main( String[] args ) { // declarations EightBall ball; Scanner scan; // instantiations ball = new EightBall(); scan = new Scanner( System.in ); // do 8 ball stuff System.out.print( " Ask a question of the Magic 8 Ball: " ); scan.nextLine(); // print the answer System.out.println( ball.shakeEightBall01() ); // do 8 ball stuff again System.out.print( " Ask another question of the Magic 8 Ball: " ); scan.nextLine(); // print the answer System.out.println( ball.shakeEightBall02() ); scan.close(); } // method main } // class EightBallDriver
Step by Step Solution
There are 3 Steps involved in it
Step: 1
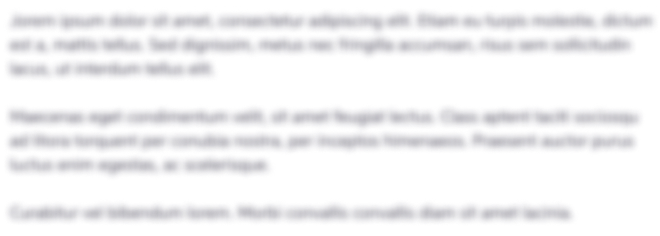
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started