Question
We need to implement a lexical analyzer which is able to recognize octal and decimal numbers with the following requirements. An input number starts with
We need to implement a lexical analyzer which is able to recognize octal and decimal numbers with the following requirements.
An input number starts with a + or - sign.
An octal number starts with a 0 and can continue with an arbitrarily number of
digits. An octal number started with more than one 0 is not an acceptable
number. Note: digits used in an octal number are between 0 and 7.
A decimal number starts with any digit except 0 and can continue with an arbitrarily number of digits. Note: digits used in a decimal number are between 0
and 9.
0 is an acceptable number, no matter whether we accept it as a decimal or as an
octal number.
You wrote a grammar and created a finite automaton for such language in homework 4.
Write C++ code to implement your finite automata.
Your program receives a string of numbers separated by space character. It analyzes every number and outputs whether that number is accepted or rejected.
Here is the sample input: +0 -03489 -00 +9803467 -184 -04650 + -034-21 Here is the sample output:
Accepted: +0 Rejected: -03489 Rejected: -00 Accepted: +9803467 Accepted: -184 Accepted: -04650 Rejected: + Rejected: -034-21
Your program reads the strings of numbers from a text file called test-data.txt and you can simply hard code the name of the data file in your program.
You implement a state-transition machine to accept or reject a number. For every input string the program begins at a starting state. The program reads every character of an input string and decides how to change the state. When the program reaches the end of an input string, the current state indicates whether the string is accepted or rejected.
Evaluation:
Your program will be tested with a test file containing acceptable and reject-able strings. Your program will be graded for:
Compiling without error or warning
Correct output (test file has the same name as this homework, but it contains
another set of test data)
Proper use of C++ data types or data structures to implement the state transition
machine and any auxiliary structure required to satisfy the requirements of this homework.
Constraints:
The whole program can be written in main() function. There is no need for creating other functions.
You are not allowed to use conditional if-else clauses in your program.
You are not allowed to use switch statement in your program.
You are allowed to use C++ standard library data types such as enum, array,
map, vector, or any other container to implement data structures required by your
program.
You need to use a 2D structure to implement the state transition machine.
You need to use a data structure for output messages.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
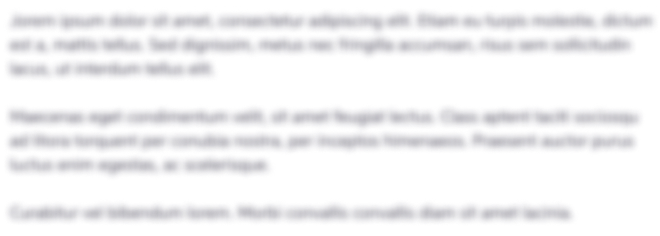
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started