Question
We put a glaze on the surface of the window. The glaze costs $2.54 a fluid ounce, and a fluid ounce will cover 10 square
We put a glaze on the surface of the window. The glaze costs $2.54 a fluid ounce, and a fluid ounce will cover 10 square inches.
Complete the Window class. It has a constructor that takes the width and height in feet of the rectangular part of the window(in that order) as parameters. Instance variables are already defined in the starter program.
Implement the following methods:
- public double getWidth() Gets the width in feet of this Window (provided)
- public double getHeight() Gets the height in feet of this Window (provided)
- public void setDimensions(double theWidth, double theHeight) Sets a new width and height in feet for the window
- public double getArea() Gets the area of the window in square inches
- public double getCostOfGlaze() Gets the cost of the glaze needed for this window.
Requirement: Do not calculate the area again inside method getCostOfGlaze, just call another method of the class. Note: the width of the rectangle is also the diameter of the semi-circle. Note: you will have to do some units conversion. Note: Use Math.PI.
Do not use magic numbers in your code. Use the following constants provided.
public static final double COST_PER_OUNCE = 2.54; public final static int SQUARE_INCHES_PER_OUNCE_OF_GLAZE = 10; public static final int SQUARE_INCHES_PER_SQUARE_FOOT = 144;
Template:
** * models a rectangular window with a semi-circle on top */ public class Window { public static final double COST_PER_OUNCE = 2.54; //constant public static final int SQUARE_INCHES_PER_SQUARE_FOOT = 144; //constant public final static int SQUARE_INCHES_PER_OUNCE_OF_GLAZE = 10; //constant private double width; private double height; //put your constructor here /** * Gets the width of this Window * @return the width of the Window */ public double getWidth() { return width; } /** * Gets the height of this Window * @return the height of the Window */ public double getHeight() { return height; } //put your methods here }
To test your code:
Use the following file:
WindowTester.java
public class WindowTester { public static void main(String[] args) { double width = 1.4; double height = 4.2; Window window1 = new Window(width, height); width = 3; height = 10; Window window2 = new Window(width, height); System.out.printf("%.2f ",window1.getArea()); System.out.println("Expected: 957.56"); System.out.printf("%.2f ",window1.getCostOfGlaze()); System.out.println("Expected: 243.22"); System.out.printf("%.2f ",window2.getArea()); System.out.println("Expected: 4828.94"); System.out.printf("%.2f ",window2.getCostOfGlaze()); System.out.println("Expected: 1226.55"); window1.setDimensions(3, 10); System.out.printf("%.2f ",window1.getCostOfGlaze()); System.out.println("Expected: 1226.55"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
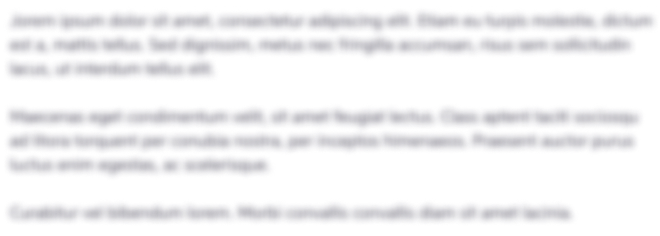
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started